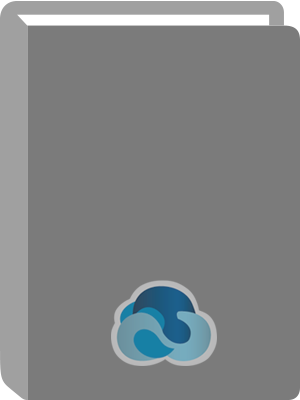
Java Foundations.
Title:
Java Foundations.
Author:
Greanier, Todd.
ISBN:
9780782151152
Personal Author:
Edition:
1st ed.
Physical Description:
1 online resource (363 pages)
Contents:
Java Foundations -- Frontmatter -- Acknowledgments -- Contents -- Introduction -- Chapter 1: The History of Java -- Where Java Technology Came From -- The Green Project -- Enter the Web -- The Features of Java Technology -- Java Is Simple -- Java Is Object Oriented -- Java Is Interpreted -- Java Is Portable -- Java Is Robust -- Java Is Secure -- Java Is Multithreaded -- Java Is High Performance -- Java Saves Time and Money -- Java Solves Important Problems -- How Java Compares with Other Languages -- How to Download and Install Java -- Downloading the J2SE Software -- Terms to Know -- Review Questions -- Chapter 2: Java Fundamentals -- Creating a Java Program -- The HelloWorld Program -- Writing the HelloWorld Source Code -- Compiling the HelloWorld Source Code -- Executing the HelloWorld Program -- Examining the Source Code -- Using Comments -- Using White Space -- Defining the Class -- Defining the Method -- Wrapping Up the HelloWorld Program -- Working with Arguments in the main() Method -- The Basic Java Data Types -- Literal Values -- The Integer Types -- The Floating Point Types -- The Character Type -- The Boolean Type -- Using the Primitive Types -- The String Class -- Primitive Values versus Reference Values -- Terms to Know -- Review Questions -- Chapter 3: Keywords and Operators -- Creating Valid Names in Java -- The Keyword List -- The Primitive Type Keywords -- The Flow Control Keywords -- Modification Keywords -- Class-Related Keywords -- Object-Related Keywords -- Wrapping Up the Keywords -- The Java Operators -- The Arithmetic Operators -- The Assignment Operators -- The Relational Operators -- The Conditional Operators -- Terms to Know -- Review Questions -- Chapter 4: Flow Control -- Application Scope -- The if Statement -- Adding the else Statement -- Testing the Array of Arguments -- The switch and case Statements.
The default Statement -- Deciding between if/else and switch/case -- Processing a Range of Values -- The Ternary Operator -- The for Loop -- Multiple Increment Steps -- Beware the Infinite Loop -- The while Loop -- Comparing for and while Loops -- The do Statement -- The Branching Statements -- The break Statement -- The continue Statement -- The return Statement -- Terms to Know -- Review Questions -- Chapter 5: Arrays -- Understanding Arrays -- Declaring Arrays -- Creating Arrays -- Getting the Length of an Array -- Populating an Array -- Using Array Initializers -- An Array Initializer Variation -- Accessing Array Elements -- Multidimensional Arrays -- Two-Dimensional Array Initializers -- Nonrectangular Arrays -- The java.util.Arrays Class -- Filling an Array -- Sorting an Array -- Searching an Array -- Terms to Know -- Review Questions -- Chapter 6: Introduction to Object-Oriented Programming -- The Object-Oriented Paradigm -- Real-World Objects -- Defining a Class -- Instantiating and Using Objects -- A Closer Look at a Lamp Object -- Sharing a Reference -- Object Messaging: Adding a Lightbulb -- Passing by Value -- Passing by Reference -- The this Keyword -- Bypassing Local Variables Using this -- Passing a Reference Using this -- Static Methods Have No this Reference -- Constructors -- Multiple Constructors -- Constructor Chaining -- Terms to Know -- Review Questions -- Chapter 7: Advanced Object-Oriented Programming -- Claiming Your Inheritance -- Using the extends Keyword -- The Rules of Inheritance -- Reference Types versus Runtime Types -- Expanding the Subclasses -- The Class Hierarchy -- The Reference Type Rule for Methods -- The instanceof Operator and Object Casting -- Object Casting -- Introducing Polymorphism -- Method Overloading -- Method Overriding -- Abstract Classes and Methods -- Interfaces -- Terms to Know -- Review Questions.
Chapter 8: Exception Handling -- The Method Call Stack -- Exception Noted -- The Exception Hierarchy -- Handling Those Exceptions -- Using try and catch -- Using a finally Clause -- Creating Your Own Exception Type -- Throwing Exceptions -- Using the throws Keyword -- The throw Keyword -- Terms to Know -- Review Questions -- Chapter 9: Common Java API Classes -- The java.lang.String Class -- Common String Methods -- The java.lang.StringBuffer Class -- The java.lang.Math Class -- Calculating a Random Number -- The Wrapper Classes -- Creating Wrapper Objects -- Common Wrapper Methods -- The Character Class -- Wrapping It Up -- Terms to Know -- Review Questions -- Chapter 10: The Collections Framework -- Defining a Framework -- The java.util.Collection Interface -- Understanding Lists -- The java.util.List Interface -- The java.util.ArrayList Class -- Summarizing Lists -- Understanding Sets -- The java.util.Set Interface -- The java.util.HashSet Class -- Summarizing Sets -- Understanding Maps -- The java.util.Map Interface -- The java.util.HashMap Class -- Summarizing Maps -- Working with Iterators -- The java.util.Iterator Interface -- The java.util.ListIterator Interface -- Iterators and Maps -- Terms to Know -- Review Questions -- Appendix A: Answers to Review Questions -- Chapter 1 -- Chapter 2 -- Chapter 3 -- Chapter 4 -- Chapter 5 -- Chapter 6 -- Chapter 7 -- Chapter 8 -- Chapter 9 -- Chapter 10 -- Glossary -- Index -- A -- B -- C -- D -- E -- F -- G -- H -- I -- J -- K -- L -- M -- N -- O -- P -- Q -- R -- S -- T -- U -- V -- W.
Abstract:
The world of IT is always evolving, but in every area there are stable, core concepts that anyone just setting out needed to know last year, needs to know this year, and will still need to know next year. The purpose of the Foundations series is to identify these concepts and present them in a way that gives you the strongest possible starting point, no matter what your endeavor. Java Foundations provides essential knowledge about what has arguably become the world's most important programming language. What you learn here will benefit you in the short term, as you acquire and practice your skills, and in the long term, as you use them. Topics covered include: The history of Java Java fundamentals Keywords and operators Flow control Arrays Basic and advanced concepts in object-oriented programming Exception handling Standard Java API classes The collections framework.
Local Note:
Electronic reproduction. Ann Arbor, Michigan : ProQuest Ebook Central, 2017. Available via World Wide Web. Access may be limited to ProQuest Ebook Central affiliated libraries.
Genre:
Electronic Access:
Click to View