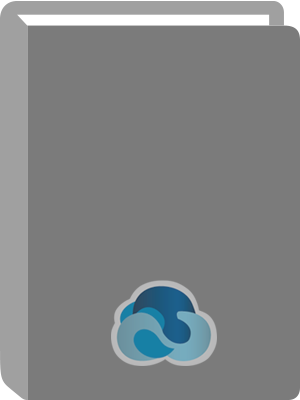
Implementing SSL / TLS Using Cryptography and PKI.
Title:
Implementing SSL / TLS Using Cryptography and PKI.
Author:
Davies, Joshua.
ISBN:
9781118038758
Personal Author:
Edition:
1st ed.
Physical Description:
1 online resource (697 pages)
Contents:
Implementing SSL/TLS Using Cryptography and PKI -- Contents -- Introduction -- Chapter 1: Understanding Internet Security -- What Are Secure Sockets? -- "Insecure" Communications: Understanding the HTTP Protocol -- Implementing an HTTP Client -- Adding Support for HTTP Proxies -- Reliable Transmission of Binary Data with Base64 Encoding -- Implementing an HTTP Server -- Roadmap for the Rest of This Book -- Chapter 2: Protecting Against Eavesdroppers with Symmetric Cryptography -- Understanding Block Cipher Cryptography Algorithms -- Implementing the Data Encryption Standard (DES) Algorithm -- DES Initial Permutation -- DES Key Schedule -- DES Expansion Function -- DES Decryption -- Padding and Chaining in Block Cipher Algorithms -- Using the Triple-DES Encryption Algorithm to Increase Key Length -- Faster Encryption with the Advanced Encryption Standard (AES) Algorithm -- AES Key Schedule Computation -- AES Encryption -- Other Block Cipher Algorithms -- Understanding Stream Cipher Algorithms -- Understanding and Implementing the RC4 Algorithm -- Converting a Block Cipher to a Stream Cipher: The OFB and COUNTER Block-Chaining Modes -- Chapter 3: Secure Key Exchange over an Insecure Medium with Public Key Cryptography -- Understanding the Theory Behind the RSA Algorithm -- Performing Arbitrary Precision Binary Math to Implement Public-Key Cryptography -- Implementing Large-Number Addition -- Implementing Large-Number Subtraction -- Implementing Large-Number Division -- Comparing Large Numbers -- Optimizing for Modulo Arithmetic -- Using Modulus Operations to Efficiently Compute Discrete Logarithms in a Finite Field -- Encryption and Decryption with RSA -- Encrypting with RSA -- Decrypting with RSA -- Encrypting a Plaintext Message -- Decrypting an RSA-Encrypted Message -- Testing RSA Encryption and Decryption.
Getting More Security per Key Bit: Elliptic Curve Cryptography -- How Elliptic Curve Cryptography Relies on Modular Inversions -- Using the Euclidean Algorithm to compute Greatest Common Denominators -- Computing Modular Inversions with the Extended Euclidean Algorithm -- Adding Negative Number Support to the Huge Number Library -- Supporting Negative Remainders -- Making ECC Work with Whole Integers: Elliptic-Curve Cryptography over Fp -- Reimplementing Diffie-Hellman to Use ECC Primitives -- Why Elliptic-Curve Cryptography? -- Chapter 4: Authenticating Communications Using Digital Signatures -- Using Message Digests to Create Secure Document Surrogates -- Implementing the MD5 Digest Algorithm -- Understanding MD5 -- A Secure Hashing Example -- Securely Hashing a Single Block of Data -- MD5 Vulnerabilities -- Increasing Collision Resistance with the SHA-1 Digest Algorithm -- Understanding SHA-1 Block Computation -- Understanding the SHA-1 Input Processing Function -- Understanding SHA-1 Finalization -- Even More Collision Resistance with the SHA-256 Digest Algorithm -- Preventing Replay Attacks with the HMAC Keyed-Hash Algorithm -- Implementing a Secure HMAC Algorithm -- Completing the HMAC Operation -- Creating Updateable Hash Functions -- Defining a Digest Structure -- Appending the Length to the Last Block -- Computing the MD5 Hash of an Entire File -- Where Does All of This Fit into SSL? -- Understanding Digital Signature Algorithm (DSA) Signatures -- Implementing Sender-Side DSA Signature Generation -- Implementing Receiver-Side DSA Signature Verification -- How to Make DSA Efficient -- Getting More Security per Bit: Elliptic Curve DSA -- Rewriting the Elliptic-Curve Math Functions to Support Large Numbers -- Implementing ECDSA -- Generating ECC Keypairs -- Chapter 5: Creating a Network of Trust Using X.509 Certificates.
Putting It Together: The Secure Channel Protocol -- Encoding with ASN.1 -- Understanding Signed Certificate Structure -- Version -- serialNumber -- signature -- issuer -- validity -- subject -- subjectPublicKeyInfo -- extensions -- Signed Certificates -- Summary of X.509 Certificates -- Transmitting Certificates with ASN.1 Distinguished Encoding Rules (DER) -- Encoded Values -- Strings and Dates -- Bit Strings -- Sequences and Sets: Grouping and Nesting ASN.1 Values -- ASN.1 Explicit Tags -- A Real-World Certificate Example -- Using OpenSSL to Generate an RSA KeyPair and Certificate -- Using OpenSSL to Generate a DSA KeyPair and Certificate -- Developing an ASN.1 Parser -- Converting a Byte Stream into an ASN.1 Structure -- The asn1parse Code in Action -- Turning a Parsed ASN.1 Structure into X.509 Certificate Components -- Joining the X.509 Components into a Completed X.509 Certificate Structure -- Parsing Object Identifiers (OIDs) -- Parsing Distinguished Names -- Parsing Certificate Extensions -- Signature Verification -- Validating PKCS #7-Formatted RSA Signatures -- Verifying a Self-Signed Certificate -- Adding DSA Support to the Certificate Parser -- Managing Certificates -- How Authorities Handle Certificate Signing Requests (CSRs) -- Correlating Public and Private Keys Using PKCS #12 Formatting -- Blacklisting Compromised Certificates Using Certificate Revocation Lists (CRLs) -- Keeping Certificate Blacklists Up-to-Date with the Online Certificate Status Protocol (OCSP) -- Other Problems with Certificates -- Chapter 6: A Usable, Secure Communications Protocol: Client-Side TLS -- Implementing the TLS 1.0 Handshake (Client Perspective) -- Adding TLS Support to the HTTP Client -- Understanding the TLS Handshake Procedure -- TLS Client Hello -- Tracking the Handshake State in the TLSParameters Structure -- Describing Cipher Suites.
Flattening and Sending the Client Hello Structure -- TLS Server Hello -- Adding a Receive Loop -- Sending Alerts -- Parsing the Server Hello Structure -- Reporting Server Alerts -- TLS Certificate -- TLS Server Hello Done -- TLS Client Key Exchange -- Sharing Secrets Using TLS PRF (Pseudo-Random Function) -- Creating Reproducible, Unpredictable Symmetric Keys with Master Secret Computation -- RSA Key Exchange -- Diffie-Hellman Key Exchange -- TLS Change Cipher Spec -- TLS Finished -- Computing the Verify Message -- Correctly Receiving the Finished Message -- Secure Data Transfer with TLS -- Assigning Sequence Numbers -- Supporting Outgoing Encryption -- Adding Support for Stream Ciphers -- Updating Each Invocation of send_message -- Decrypting and Authenticating -- TLS Send -- TLS Receive -- Implementing TLS Shutdown -- Examining HTTPS End-to-end Examples (TLS 1.0) -- Dissecting the Client Hello Request -- Dissecting the Server Response Messages -- Dissecting the Key Exchange Message -- Decrypting the Encrypted Exchange -- Exchanging Application Data -- Differences Between SSL 3.0 and TLS 1.0 -- Differences Between TLS 1.0 and TLS 1.1 -- Chapter 7: Adding Server-Side TLS 1.0 Support -- Implementing the TLS 1.0 Handshake from the Server's Perspective -- TLS Client Hello -- TLS Server Hello -- TLS Certificate -- TLS Server Hello Done -- TLS Client Key Exchange -- RSA Key Exchange and Private Key Location -- Supporting Encrypted Private Key Files -- Checking That Decryption was Successful -- Completing the Key Exchange -- TLS Change Cipher Spec -- TLS Finished -- Avoiding Common Pitfalls When Adding HTTPS Support to a Server -- When a Browser Displays Errors: Browser Trust Issues -- Chapter 8: Advanced SSL Topics -- Passing Additional Information with Client Hello Extensions -- Safely Reusing Key Material with Session Resumption.
Adding Session Resumption on the Client Side -- Requesting Session Resumption -- Adding Session Resumption Logic to the Client -- Restoring the Previous Session's Master Secret -- Testing Session Resumption -- Viewing a Resumed Session -- Adding Session Resumption on the Server Side -- Assigning a Unique Session ID to Each Session -- Adding Session ID Storage -- Modifying parse_client_hello to Recognize Session Resumption Requests -- Drawbacks of This Implementation -- Avoiding Fixed Parameters with Ephemeral Key Exchange -- Supporting the TLS Server Key Exchange Message -- Authenticating the Server Key Exchange Message -- Examining an Ephemeral Key Exchange Handshake -- Verifying Identity with Client Authentication -- Supporting the CertificateRequest Message -- Adding Certificate Request Parsing Capability for the Client -- Handling the Certificate Request -- Supporting the Certificate Verify Message -- Refactoring rsa_encrypt to Support Signing -- Testing Client Authentication -- Viewing a Mutually-Authenticated TLS Handshake -- Dealing with Legacy Implementations: Exportable Ciphers -- Export-Grade Key Calculation -- Step-up Cryptography -- Discarding Key Material Through Session Renegotiation -- Supporting the Hello Request -- Renegotiation Pitfalls and the Client Hello Extension 0xFF01 -- Defending Against the Renegotiation Attack -- Implementing Secure Renegotiation -- Chapter 9: Adding TLS 1.2 Support to Your TLS Library -- Supporting TLS 1.2 When You Use RSA for the Key Exchange -- TLS 1.2 Modifications to the PRF -- TLS 1.2 Modifications to the Finished Messages Verify Data -- Impact to Diffie-Hellman Key Exchange -- Parsing Signature Types -- Adding Support for AEAD Mode Ciphers -- Maximizing Throughput with Counter Mode -- Reusing Existing Functionality for Secure Hashes with CBC-MAC -- Combining CTR and CBC-MAC into AES-CCM.
Maximizing MAC Throughput with Galois-Field Authentication.
Abstract:
Hands-on, practical guide to implementing SSL and TLS protocols for Internet security If you are a network professional who knows C programming, this practical book is for you. Focused on how to implement Secure Socket Layer (SSL) and Transport Layer Security (TLS), this book guides you through all necessary steps, whether or not you have a working knowledge of cryptography. The book covers SSLv2, TLS 1.0, and TLS 1.2, including implementations of the relevant cryptographic protocols, secure hashing, certificate parsing, certificate generation, and more. Coverage includes: Understanding Internet Security Protecting against Eavesdroppers with Symmetric Cryptography Secure Key Exchange over an Insecure Medium with Public Key Cryptography Authenticating Communications Using Digital Signatures Creating a Network of Trust Using X.509 Certificates A Usable, Secure Communications Protocol: Client-Side TLS Adding Server-Side TLS 1.0 Support Advanced SSL Topics Adding TLS 1.2 Support to Your TLS Library Other Applications of SSL A Binary Representation of Integers: A Primer Installing TCPDump and OpenSSL Understanding the Pitfalls of SSLv2 Set up and launch a working implementation of SSL with this practical guide.
Local Note:
Electronic reproduction. Ann Arbor, Michigan : ProQuest Ebook Central, 2017. Available via World Wide Web. Access may be limited to ProQuest Ebook Central affiliated libraries.
Genre:
Electronic Access:
Click to View