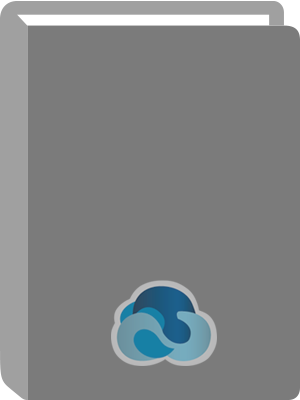
Programming Role Playing Games with DirectX.
Title:
Programming Role Playing Games with DirectX.
Author:
Adams, Jim.
ISBN:
9781592002139
Personal Author:
Physical Description:
1 online resource (1102 pages)
Contents:
Title -- Copyright -- Dedication -- Acknowledgments -- About the Author -- Contents at a Glance -- Contents -- Foreword -- Introduction -- What This Book Is About -- Who Should Read This Book -- How This Book Is Organized -- What's on the CD -- Conventions Used in This Book -- What You Need to Begin -- Part One An Introduction to Role-Playing Games -- CHAPTER 1 A World of Role-Playing -- A Story of Role-Playing -- The Concepts of Role-Playing -- The Basic Concepts -- Playing Traditional Pen-and-Paper Games -- Going Live with Role-Playing Games -- Role-Playing on the Computer -- The Evolution of Gaming -- Wrapping Up with a Look into the Future -- Part Two Role-Playing Game Design -- CHAPTER 2 Exploring RPG Design Elements -- General Game Design Issues -- The Importance of Design Documents -- Creating Your Design Document -- Starting with the Table of Contents -- Adding the Topics -- The Perfect Design Document -- RPG Design Aspects -- Turning to the Technical Side -- Knowing Your Role -- Wrapping Up Design -- CHAPTER 3 Story-Writing Essentials -- The Art of Telling Stories -- The Five Components of a Story -- The Story Ladder and the Three Acts -- Act 1: The Beginning Story Flow -- Act 2: The Middle -- Act 3: The End -- Characters -- Know Your Roles -- Building Three-Dimensional Characters -- Dialogue -- Setting Mood and Atmosphere -- The Point of View -- Your Narrative Voice -- Plots, Subplots, and Twists -- Plot Twists -- Subplots -- The Writing Process -- Eight Rules to Writing -- Six Steps to Writing -- Thought and Planning -- Shaping Your Thoughts -- Writing a Draft -- Revising the Story -- Editing -- Proofreading -- Writing the Three Drafts -- The Rough Draft -- The Revision Draft -- The Cut-and-Polish Draft -- Tips for Better Stories -- Back-Stories -- Flashbacks and Cut-Scenes -- Foreshadowing -- Don't Say It-Experience It.
Harnessing Emotion -- Studying the Greats -- Applying Stories to Games -- Enveloping the Player -- Breaking Up the Plot -- Linear and Nonlinear Story Lines -- Dialogue -- Involving the Design Document -- Wrapping Up Stories -- Part Three Programming Basics -- CHAPTER 4 Starting with C++ -- Introducing C++ -- Moving from C to C++ -- Working with Functions -- Function Prototyping -- Default Function Argument Values -- Function Overloading -- Inline Functions -- Working with Variables -- Variable Declaration -- Scope and Precedence -- Static Variables -- Protecting with Const -- New Keywords and Enhancements -- Memory Allocation -- NULL and Enum -- Classes -- Class Visibility -- Class Variables and Functions -- Using Static Variables and Functions -- The Constructor and Destructor -- Operator Functions -- Using the this Keyword -- Class Friends -- Derived Classes -- Virtual Functions -- Using Const with Classes -- Advanced Structures -- Wrapping Up C++ -- CHAPTER 5 Programming with Windows and Application Basics -- Programming with Windows -- Coding Conventions -- Hungarian Notation -- Win32 Data Types -- Function Naming -- Working Inside a Window -- Including the Headers -- The WinMain Function -- Events and Messages -- Registering a Windows Class -- Creating a Window -- The Message Pump -- The Window Message Procedure -- Common Messages -- An Application Shell -- Advanced Features -- Using Message Boxes -- Dialog Boxes -- Resources -- Attaching Resources to an Application -- Retrieving Resource Data -- Threads and Multithreading -- Critical Sections -- Using COM -- Initializing COM -- IUnknown -- Initializing and Releasing Objects -- Querying Interfaces -- DirectX -- Downloading and Installing DirectX -- Including DirectX in Your Project -- Understanding the Program Flow -- Modular Programming -- States and Processes -- Application States -- Processes.
Handling Application Data -- Using Data Packaging -- Testing the Data Package System -- Building an Application Framework -- Structuring a Project -- Debugging Your Program -- Wrapping Up Windows and Application Basics -- CHAPTER 6 Drawing with DirectX Graphics -- The Heart of 3-D Graphics -- Coordinate Systems -- Constructing Objects -- Lists, Strips, and Fans -- Vertex Ordering -- Coloring Polygons -- Transformations -- Getting Started with DirectX Graphics -- Direct3D Components -- Initializing the System -- Obtaining the Direct3D Interface -- Selecting a Display Mode -- Setting the Presentation Method -- Creating the Device Interface and Initializing the Display -- Losing the Device -- Introducing D3DX -- The Math of 3-D -- Matrix Math -- Matrix Construction -- Combining Matrices -- The Steps from Local to View Coordinates -- Getting Down to Drawing -- Using Vertices -- Flexible Vertex Format -- Using Vertex Buffers -- Creating a Vertex Buffer -- Locking the Vertex Buffer -- Stuffing in Vertex Data -- Vertex Streams -- Vertex Shaders -- Transformations -- The World Transformation -- The View Transformation -- The Projection Transformation -- Materials and Colors -- Clearing the Viewport -- Beginning and Ending a Scene -- Rendering Polygons -- Presenting the Scene -- Using Texture Maps -- Using Texture-Mapping with Direct3D -- Loading a Texture -- Setting the Texture -- Using Texture Filters -- Rendering Textured Objects -- Alpha Blending -- Enabling Alpha Blending -- Drawing with Alpha Blending -- Transparent Blitting with Alpha Testing -- Loading Textures with Color Keying -- Enabling Alpha Testing -- A Transparent Blitting Example -- Lighting -- Using Point Lights -- Using Spotlights -- Using Directional Lights -- Ambient Light -- Setting the Light -- Using Normals -- Let There Be Light! -- Using Fonts -- Creating the Font -- Drawing with Fonts.
Billboards -- Particles -- Depth Sorting and Z-Buffering -- Working with Viewports -- Working with Meshes -- The .X Files -- The .X File Format -- Templates Galore -- Using a Frame Hierarchy -- Creating .X Meshes -- Parsing .X Files -- Meshes with D3DX -- The ID3DXBuffer Object -- Standard Meshes -- Rendering Meshes -- Skinned Meshes -- Loading Skinned Meshes -- Updating and Rendering a Skinned Mesh -- Using 3-D Animation .X Style -- Key Frame Techniques -- Animation in .X -- Wrapping Up Graphics -- CHAPTER 7 Interacting with DirectInput -- Introducing Input Devices -- Interacting via the Keyboard -- Dealing with the Keyboard in Windows -- Playing with the Mouse -- Jammin' with the Joystick -- Using DirectInput -- Presenting DirectInput Basics -- Initializing DirectInput -- Employing DirectInput Devices -- Obtaining a Device GUID -- Creating the Device COM Object -- Setting the Data Format -- Setting the Cooperative Level -- Setting Special Properties -- Acquiring the Device -- Polling the Device -- Reading In Data -- Using DirectInput with the Keyboard -- Using DirectInput with the Mouse -- Using DirectInput with Joysticks -- Wrapping Up Input -- CHAPTER 8 Playing Sound with DirectX Audio -- Sound Basics -- Recording Digital Sounds -- Musical Madness -- Midi -- DirectMusic -- Understanding DirectX Audio -- Using DirectSound -- Initializing DirectSound -- Setting the Cooperative Level -- Setting the Playback Format -- Creating the Primary Sound Buffer Object -- Setting the Format -- Jump-Starting the Primary Sound Buffer -- Using Secondary Sound Buffers -- Lock and Load-Loading Sound Data into the Buffer -- Playing the Sound Buffer -- Altering Volume, Panning, and Frequency Settings -- Volume Control -- Panning -- Frequency Changes -- Losing Focus -- Using Notifications -- Using Threads for Events -- Loading Sounds into the Buffers -- Streaming Sound.
Working with DirectMusic -- Starting with DirectMusic -- Creating the Performance Object -- Creating the Loader Object -- Working with Music Segments -- Loading Music Segments -- Loading Instruments -- Configuring for Midi -- Setting Up the Instruments -- Using Loops and Repeats -- Playing and Stopping the Segment -- Unloading Segment Data -- Altering Music -- Volume Settings -- Tempo Changes -- Grabbing an Audio Channel -- Finishing Up Sound -- CHAPTER 9 Networking with DirectPlay -- Understanding Networking -- Network Models -- Lobbies -- Latency and Lag -- Communication Protocols -- Addressing -- Introducing DirectPlay -- The Network Objects -- Working with Players -- Networking with Messages -- Asynchronous and Synchronous -- Security -- Guaranteed Delivery -- Throttling -- From Small Bytes to Big Words -- Identifying Applications with GUIDs -- Initializing a Network Object -- Using Addresses -- Initializing the Address Object -- Adding Components -- Setting the Service Provider -- Selecting a Port -- Assigning a Device -- Using Message Handlers -- Configuring Session Information -- Server Session Data -- Client Session Data -- Working with Servers -- Handling Players -- Dealing with Create-Player Messages -- Retrieving a Player's Name -- Destroying Players -- Receiving Data -- Sending Server Messages -- Ending the Host Session -- Working with Clients -- Sending and Receiving Messages -- Terminating the Client Session -- Wrapping Up Networking -- CHAPTER 10 Creating the Game Core -- Understanding the Core Concept -- The System Core -- Using the cApplication Core Object -- State Processing with cStateManager -- Processes and cProcessManager -- Managing Data with cDataPackage -- The Graphics Core -- The Graphics System with cGraphics -- Images with cTexture -- Colors and cMaterial -- Light It Up with cLight -- Text and Fonts Using cFont.
Vertices and cVertexBuffer.
Local Note:
Electronic reproduction. Ann Arbor, Michigan : ProQuest Ebook Central, 2017. Available via World Wide Web. Access may be limited to ProQuest Ebook Central affiliated libraries.
Genre:
Electronic Access:
Click to View