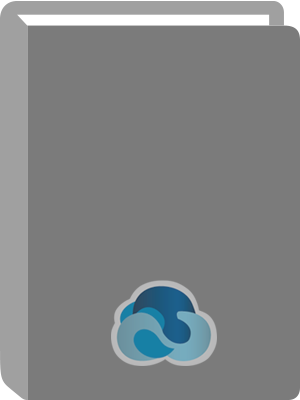
Advanced iOS 4 Programming : Developing Mobile Applications for Apple iPhone, iPad, and iPod Touch.
Title:
Advanced iOS 4 Programming : Developing Mobile Applications for Apple iPhone, iPad, and iPod Touch.
Author:
Ali, Maher.
ISBN:
9780470979549
Personal Author:
Edition:
1st ed.
Physical Description:
1 online resource (722 pages)
Contents:
Advanced iOS 4 Programming -- Contents -- Preface -- Publisher's Acknowledgments -- 1 Getting Started -- 1.1 iOS SDK and IDE Basics -- 1.1.1 Obtaining and installing the SDK -- 1.1.2 Creating a project -- 1.1.3 Familiarizing yourself with the IDE -- 1.1.4 Looking closely at the generated code -- 1.2 Creating Interfaces -- 1.2.1 Interface Builder -- 1.2.2 Revising the application -- 1.3 Using the Debugger -- 1.4 Getting More Information -- 1.5 Summary -- Exercises -- 2 Objective-C and Cocoa -- 2.1 Classes -- 2.1.1 Class declaration -- 2.1.2 How do I use other declarations? -- 2.1.3 Class definition -- 2.1.4 Method invocation and definition -- 2.1.5 Important types -- 2.1.6 Important Cocoa classes -- 2.2 Memory Management -- 2.2.1 Creating and deallocating objects -- 2.2.2 Preventing memory leaks -- 2.3 Protocols -- 2.4 Properties -- 2.4.1 Property declaration -- 2.4.2 Circular references -- 2.5 Categories -- 2.6 Posing -- 2.7 Exceptions and Errors -- 2.7.1 Exceptions -- 2.7.2 Errors -- 2.8 Key-Value Coding (KVC) -- 2.8.1 An example illustrating KVC -- 2.8.2 KVC in action -- 2.9 Multithreading -- 2.10 Notifications -- 2.11 Blocks -- 2.11.1 Declaration and definition -- 2.11.2 Block literal -- 2.11.3 Invocation -- 2.11.4 Variable binding -- 2.12 Grand Central Dispatch (GCD) -- 2.12.1 Queues -- 2.12.2 Scheduling a task -- 2.12.3 Putting it together -- 2.13 The Objective-C Runtime -- 2.13.1 Required header files -- 2.13.2 The NSObject class -- 2.13.3 Objective-C methods -- 2.13.4 Examples -- 2.14 Summary -- Exercises -- 3 Collections -- 3.1 Arrays -- 3.1.1 Immutable copy -- 3.1.2 Mutable copy -- 3.1.3 Deep copy -- 3.1.4 Sorting an array -- 3.2 Sets -- 3.2.1 Immutable sets -- 3.2.2 Mutable sets -- 3.2.3 Additional important methods for NSSet -- 3.3 Dictionaries -- 3.4 Summary -- Exercises -- 4 Anatomy of an iPhone Application.
4.1 Hello World Application -- 4.1.1 Create a main.m file -- 4.1.2 Create the application delegate class -- 4.1.3 Create the user interface subclasses -- 4.2 Building the Hello World Application -- 4.3 Summary -- Exercises -- 5 The View -- 5.1 View Geometry -- 5.1.1 Useful geometric type definitions -- 5.1.2 The UIScreen class -- 5.1.3 The frame and center properties -- 5.1.4 The bounds property -- 5.2 The View Hierarchy -- 5.3 The Multitouch Interface -- 5.3.1 The UITouch class -- 5.3.2 The UIEvent class -- 5.3.3 The UIResponder class -- 5.3.4 Handling a swipe -- 5.3.5 More advanced gesture recognition -- 5.4 Animation -- 5.4.1 Using the UIView class animation support -- 5.4.2 Sliding view -- 5.4.3 Flip animation -- 5.4.4 Transition animation -- 5.5 Drawing -- 5.5.1 Fundamentals -- 5.5.2 The Summary View application -- 5.6 Summary -- Exercises -- 6 Controls -- 6.1 The Foundation of All Controls -- 6.1.1 UIControl attributes -- 6.1.2 Target-action mechanism -- 6.2 The Text Field -- 6.2.1 Interacting with the keyboard -- 6.2.2 The delegate -- 6.2.3 Creating and working with a UITextField -- 6.3 Sliders -- 6.4 Switches -- 6.5 Buttons -- 6.6 Segmented Controls -- 6.7 Page Controls -- 6.8 Date Pickers -- 6.9 Summary -- Exercises -- 7 View Controllers -- 7.1 The Simplest View Controller -- 7.1.1 The view controller -- 7.1.2 The view -- 7.1.3 The application delegate -- 7.1.4 A simple MVC application -- 7.2 Tab-Bar Controllers -- 7.2.1 A detailed example of a tab-bar application -- 7.2.2 Some comments on tab-bar controllers -- 7.3 Navigation Controllers -- 7.3.1 A detailed example of a navigation controller -- 7.3.2 Customization -- 7.4 Modal View Controllers -- 7.5 Summary -- Exercises -- 8 Special-Purpose Views -- 8.1 Picker View -- 8.1.1 The delegate -- 8.1.2 An example of picker view -- 8.2 Progress View -- 8.3 Scroll View -- 8.4 Text View.
8.4.1 The delegate -- 8.4.2 An example of text view -- 8.5 Alert View -- 8.6 Action Sheet -- 8.7 Web View -- 8.7.1 A simple web view application -- 8.7.2 Viewing local files -- 8.7.3 Evaluating JavaScript -- 8.7.4 The web view delegate -- 8.8 Summary -- Exercises -- 9 Table View -- 9.1 Overview -- 9.2 The Simplest Table View Application -- 9.3 A Table View with Both Images and Text -- 9.4 A Table View with Section Headers and Footers -- 9.5 A Table View with the Ability to Delete Rows -- 9.6 A Table View with the Ability to Insert Rows -- 9.7 Reordering Table Rows -- 9.8 Presenting Hierarchical Information -- 9.8.1 Detailed example -- 9.9 Grouped Table Views -- 9.10 Indexed Table Views -- 9.11 Dynamic Table Views -- 9.12 Whitening Text in Custom Cells -- 9.13 Summary -- Exercises -- 10 File Management -- 10.1 The Home Directory -- 10.2 Enumerating a Directory -- 10.3 Creating and Deleting a Directory -- 10.4 Creating Files -- 10.5 Retrieving and Changing Attributes -- 10.5.1 Retrieving attributes -- 10.5.2 Changing attributes -- 10.6 Working with Resources and Low-Level File Access -- 10.7 Summary -- Exercises -- 11 Working with Databases -- 11.1 Basic Database Operations -- 11.1.1 Opening, creating, and closing databases -- 11.1.2 Table operations -- 11.2 Processing Row Results -- 11.3 Prepared Statements -- 11.3.1 Preparation -- 11.3.2 Execution -- 11.3.3 Finalization -- 11.3.4 Putting it together -- 11.4 User-Defined Functions -- 11.5 Storing BLOBs -- 11.6 Retrieving BLOBs -- 11.7 Summary -- Exercises -- 12 XML Processing -- 12.1 XML and RSS -- 12.1.1 XML -- 12.1.2 RSS -- 12.1.3 Configuring the XCode project -- 12.2 Document Object Model (DOM) -- 12.3 Simple API for XML (SAX) -- 12.4 An RSS Reader Application -- 12.5 Putting It Together -- 12.6 Summary -- Exercises -- 13 Location Awareness -- 13.1 The Core Location Framework.
13.1.1 The CLLocation class -- 13.2 A Simple Location-Aware Application -- 13.3 Google Maps API -- 13.4 A Tracking Application with Maps -- 13.5 Working with Zip Codes -- 13.6 Working with the Map Kit API -- 13.6.1 The MKMapView class -- 13.6.2 The MKCoordinateRegion structure -- 13.6.3 The MKAnnotation protocol -- 13.6.4 The MKAnnotationView class -- 13.6.5 The MKUserLocation class -- 13.6.6 The MKPinAnnotationView class -- 13.7 Summary -- Exercises -- 14 Working with Devices -- 14.1 Working with the Accelerometer -- 14.1.1 Basic accelerometer values -- 14.1.2 Accelerometer example -- 14.2 Working with Audio -- 14.2.1 Playing short audio files -- 14.2.2 Recording audio files -- 14.2.3 Playing audio files -- 14.2.4 Using the media picker controller -- 14.2.5 Searching the iPod Library -- 14.3 Playing Video -- 14.4 Accessing Device Information -- 14.5 Taking and Selecting Pictures -- 14.5.1 Overall approach to taking and selecting pictures -- 14.5.2 Detailed example of taking and selecting pictures -- 14.6 Monitoring the Device Battery -- 14.6.1 Battery level -- 14.6.2 Battery state -- 14.6.3 Battery state and level notifications -- 14.6.4 Putting it together -- 14.7 Accessing the Proximity Sensor -- 14.7.1 Enabling proximity monitoring -- 14.7.2 Subscribing to proximity change notification -- 14.7.3 Retrieving the proximity state -- 14.8 Summary -- Exercises -- 15 Internationalization -- 15.1 String Localization -- 15.2 Date Formatting -- 15.3 Number Formatting -- 15.4 Sorted List of Countries -- 15.5 Summary -- Exercises -- 16 Custom User Interface Components -- 16.1 Text Field Alert View -- 16.2 Table Alert View -- 16.3 Progress Alert View -- 16.4 Summary -- Exercises -- 17 Advanced Networking -- 17.1 Determining Network Connectivity -- 17.1.1 Determining network connectivity via EDGE or GPRS -- 17.1.2 Determining network connectivity in general.
17.1.3 Determining network connectivity via Wi-Fi -- 17.2 Uploading Multimedia Content -- 17.3 Computing MD5 Hash Value -- 17.4 Multithreaded Downloads -- 17.4.1 The Multithreaded Downloads application -- 17.4.2 Asynchronous networking -- 17.5 Push Notification -- 17.5.1 Configuring push notification on the server -- 17.5.2 Configuring the client -- 17.5.3 Coding the client -- 17.5.4 Coding the server -- 17.6 Local Notification -- 17.7 Large Downloads and Uploads -- 17.8 Sending Email -- 17.9 Summary -- Exercises -- 18 Working with the Address Book Database -- 18.1 Introduction -- 18.2 Property Types -- 18.3 Accessing Single-Value Properties -- 18.3.1 Retrieving single-value properties -- 18.3.2 Setting single-value properties -- 18.4 Accessing Multivalue Properties -- 18.4.1 Retrieving multivalue properties -- 18.4.2 Setting multivalue properties -- 18.5 Person and Group Records -- 18.6 Address Book -- 18.7 Multithreading and Identifiers -- 18.8 Person Photo Retriever Application -- 18.9 Using the ABUnknownPersonViewController Class -- 18.10 Using the ABPeoplePickerNavigationController Class -- 18.11 Using the ABPersonViewController Class -- 18.12 Using the ABNewPersonViewController Class -- 18.13 Summary -- Exercises -- 19 Core Data -- 19.1 Core Data Application Components -- 19.2 Key Players -- 19.2.1 Entity -- 19.2.2 Managed object model -- 19.2.3 Persistent store coordinator -- 19.2.4 Managed object context -- 19.2.5 Managed object -- 19.2.6 The Core Data wrapper class -- 19.3 Using the Modeling Tool -- 19.4 Create, Read, Update, and Delete (CRUD) -- 19.4.1 Create -- 19.4.2 Delete -- 19.4.3 Read and update -- 19.5 Working with Relationships -- 19.6 A Search Application -- 19.6.1 The UISearchDisplayController class -- 19.6.2 Main pieces -- 19.7 Summary -- Exercises -- 20 Undo Management -- 20.1 Understanding Undo Management -- 20.1.1 Basic idea.
20.1.2 Creating an undo manager.
Abstract:
With Advanced iOS 4 Programming, developers have the expert guidance they need to create amazing applications for Apple's iPhone, iPad, and iPod touch. Inside, veteran mobile developer Dr. Maher Ali begins with a foundation introduction to Objective C and Cocoa Touch programming, and then guides readers through building apps with Apple's iPhone SDK 4 - including coverage of the major categories of new APIs and building apps for the new Apple iPad. This book concentrates on illustrating GUI concepts programmatically, allowing readers to fully appreciate the complete picture of iOS 4 development without relying on Interface Builder. In addition, Interface Builder is covered in several chapters. Advanced iOS 4 Programming delves into more advanced topics going beyond the basics of iOS 4 development, providing comprehensive coverage that will help you get your apps to the App Store quicker. Key features include: Objective-C programming language and runtime Interface Builder Building advanced mobile user interfaces Collections Cocoa Touch Core Animation and Quartz 2D Model-view-controller (MVC) designs Developing for the iPad Grand Central Dispatch Parsing XML documents using SAX, DOM, and TouchXML Working with the Map Kit API Remote and Local Push Notification Blocks (closures) in Objective-C Building advanced location-based applications Developing database applications using the SQLite engine GameKit framework.
Local Note:
Electronic reproduction. Ann Arbor, Michigan : ProQuest Ebook Central, 2017. Available via World Wide Web. Access may be limited to ProQuest Ebook Central affiliated libraries.
Genre:
Electronic Access:
Click to View