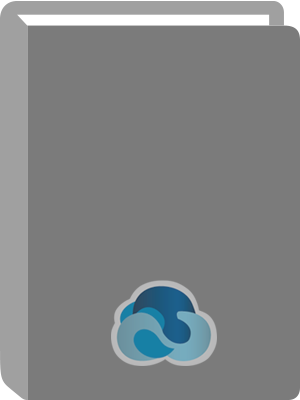
Mastering Visual C# .NET.
Title:
Mastering Visual C# .NET.
Author:
Price, Jason.
ISBN:
9780782152234
Personal Author:
Edition:
1st ed.
Physical Description:
1 online resource (1005 pages)
Contents:
Contents -- Introduction -- Part 1 Fundamental C# Programming -- Chapter 1 Introduction to C# -- Developing Your First C# Program -- Introducing Visual Studio .NET -- Using the .NET Documentation -- Summary -- Chapter 2 Basic C# Programming -- Using Statements, Whitespace, and Blocks -- Adding Comments -- Using Data Types, Variables, and Constants -- Introducing Strings -- Understanding Enumerations -- Handling Input and Output -- Summary -- Chapter 3 Expressions and Operators -- Understanding Expressions and Operators -- Operator Precedence -- Summary -- Chapter 4 Decisions, Loops, and Preprocessor Directives -- Using the if Statement -- Implementing the switch Statement -- Using Loop Statements -- Understanding Jump Statements -- Creating Preprocessor Directives -- Summary -- Chapter 5 Object-Oriented Programming -- Introducing Classes and Objects -- Declaring a Class -- Creating Objects -- Using Methods -- Using Access Modifiers -- Creating and Destroying Objects -- Introducing Structs -- Summary -- Chapter 6 More about Classes and Objects -- Introducing Static Members -- Using Readonly Fields -- Defining Properties -- Introducing the "Has a" Relationship -- Learning about Namespaces -- Summary -- Chapter 7 Derived Classes -- Introducing Inheritance -- Learning about Polymorphism -- Specifying Member Accessibility -- Hiding Members -- Versioning -- Using the System.Object Class -- Using Abstract Classes and Methods -- Declaring Sealed Classes and Methods -- Casting Objects -- Operator Overloading -- Summary -- Chapter 8 Interfaces -- Defining an Interface -- Implementing an Interface Using a Class -- Casting an Object to an Interface -- Using Derived Interfaces -- Understanding Explicit Interface Members -- Summary -- Chapter 9 Strings, Dates, Times, and Time Spans -- Using Strings -- Creating Dynamic Strings -- Representing Dates and Times.
Using Time Spans -- Summary -- Chapter 10 Arrays and Indexers -- Declaring and Creating Arrays -- Using Arrays -- Initializing Arrays -- Reading Command-Line Arguments -- Introducing Array Properties and Methods -- Using Multidimensional Arrays -- Creating Arrays of Objects -- Introducing Indexers -- Summary -- Chapter 11 Collections -- Introducing Array Lists -- Understanding Bit Arrays -- Understanding Hash Tables -- Understanding Sorted Lists -- Understanding Queues -- Understanding Stacks -- Summary -- Chapter 12 Delegates and Events -- Understanding Delegates -- Understanding Events -- Summary -- Chapter 13 Exceptions and Debugging -- Handling Exceptions -- Understanding Exception Objects -- Handling Specific Exceptions -- Exploring Exception Propagation -- Creating and Throwing Exception Objects -- Declaring Custom Exceptions -- Debugging -- Summary -- Part 2 Advanced C# Programming -- Chapter 14 Threads -- Understanding the .NET Framework Class Library -- Understanding Threads -- Managing Threads -- Dealing with Thread Problems -- Thread Pooling -- Summary -- Chapter 15 Streams and Input/Output -- Dealing with Files and Directories -- Exploring Streams and Backing Stores -- Using Readers and Writers -- Using Asynchronous I/O -- Introducing Serialization -- Summary -- Chapter 16 Assemblies -- Looking at the Big Picture -- What's in an Assembly? -- Building Assemblies -- Viewing Assembly Contents -- Understanding Strong Names and Signing -- Assembly Versioning -- Working with the Global Assembly Cache -- Finding an Assembly -- Summary -- Chapter 17 Attributes and Reflection -- Using Attributes -- Discovering Types at Run-Time -- Creating Types at Run-Time -- Summary -- Chapter 18 Remoting -- Understanding Application Domains -- Understanding Marshaling with Proxies -- Understanding Contexts -- Understanding Channels -- Using Remoting -- Summary.
Chapter 19 Security -- Using Code-Access Security -- Using Role-Based Security -- Using Encryption -- Summary -- Chapter 20 XML -- Understanding XML -- Reading and Writing XML -- Using the Document Object Model -- Transforming XML -- Summary -- Chapter 21 Other Classes in the Base Class Library -- Understanding the Graphics Classes -- Supporting Globalization -- Diagnostics and Debugging -- Using Advanced Facilities -- Summary -- Part 3 .NET Programming with C# -- Chapter 22 Introduction to Databases -- Introducing Databases -- Exploring the Northwind Database -- Using the Structured Query Language (SQL) -- Introducing Stored Procedures -- Accessing a Database Using Visual Studio .NET -- Summary -- Chapter 23 Active Data Objects: ADO.NET -- Overview of ADO.NET -- Overview of the ADO.NET Classes -- Performing a SQL SELECT Statement Using ADO.NET -- Connecting to a Microsoft Access Database -- Connecting to an Oracle Database -- Exploring the Details of the ADO.NET Classes -- Performing SQL INSERT, UPDATE, and DELETE Statements Using ADO.NET -- Modifying a DataTable Object and Synchronizing the Changes with the Database -- Using a Transaction in ADO.NET -- Using a DataView Object to Filter and Sort Rows -- Defining and Using a Relationship between Two DataTable Objects -- Running a SQL Server Stored Procedure Using ADO.NET -- Writing and Reading XML Files Using ADO.NET -- Summary -- Chapter 24 Introduction to Windows Applications -- Developing a Simple Windows Application -- Using Windows Controls -- Using a DataGrid Control to Access a Database -- Using the Data Form Wizard to Create a Windows Form -- Summary -- Chapter 25 Active Server Pages: ASP.NET -- Creating a Simple ASP.NET Web Application -- Using Web Form Controls -- Building a More Complex Application -- Using a DataGrid Control to Access a Database.
Using a DataList Control to Access a Database -- Summary -- Chapter 26 Web Services -- Exploring the Architecture of Web Services -- Building a Simple Web Service -- Watching the Conversation -- Looking Inside the Web Services Proxy -- Building a More Complex Web Service -- Building a Client the Easy Way -- Exploring Web Services Registries -- Summary -- Appendices -- Appendix A C# Keywords -- Appendix B: C# Compiler Options -- Appendix C Regular Expressions -- Concepts -- Metacharacters -- The Regular Expression Classes -- Regular Expression Examples -- Groups and Captures -- Index -- Symbols and Numbers -- A -- B -- C -- D -- E -- F -- G -- H -- I -- J -- K -- L -- M -- N -- O -- P -- Q -- R -- S -- T -- U -- V -- W -- X -- Y -- Z.
Abstract:
Get Everything You Can Out of Visual C# and the .NET Framework Mastering Visual C# .NET is the best resource for getting everything you can out of the new C# language and the .NET Framework. You'll master C# language essentials, quickly taking advantage of the many improvements it offers over C++ and see tons of examples that show you all the ways that .NET can make your programming more efficient and your applications more powerful. You'll learn how to create stand-alone applications, as well as build Windows, web, and database applications. You'll even see how to develop web services-a technology that holds great promise for the future of distributed application. Coverage includes: Mastering the fundamentals and advanced aspects of the C# language Using Visual Studio .NET for increased coding productivity and debugging Creating distributed applications with remoting and web services Understanding object-oriented concepts Delivering data across the Internet with web services Using XML to communicate with other applications Accessing databases with ADO.NET Building Windows applications Creating web applications using ASP.NET Reading and writing data from/to files or the Internet Using advanced data structures to store and manipulate information Using multi-threading for greater application efficiency Using reflection to manipulate running code Building distributed applications with remoting and web services Securing code and authenticating users Using built-in encryption facilities Making your applications world-ready Parsing strings with regular expressions Using delegates to handle runtime events Programming defensively with exception handling.
Local Note:
Electronic reproduction. Ann Arbor, Michigan : ProQuest Ebook Central, 2017. Available via World Wide Web. Access may be limited to ProQuest Ebook Central affiliated libraries.
Genre:
Electronic Access:
Click to View