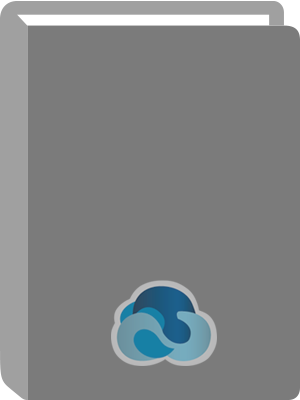
Python Programming for the Absolute Beginner.
Title:
Python Programming for the Absolute Beginner.
Author:
Dawson, Michael.
ISBN:
9781592002696
Personal Author:
Physical Description:
1 online resource (480 pages)
Contents:
Title -- Copyright -- Dedication -- Acknowledgments -- About the Author -- Contents at a Glance -- Contents -- Introduction -- CHAPTER 1 Getting Started: The Game Over Program -- Examining the Game Over Program -- Introducing Python -- Python Is Easy to Use -- Python Is Powerful -- Python Is Object-Oriented -- Python Is a "Glue" Language -- Python Runs Everywhere -- Python Has a Strong Community -- Python Is Free and Open Source -- Setting Up Python on Windows -- Installing Python on Windows -- Setting Up Python on Other Operating Systems -- Introducing the Python IDLE -- Programming in Interactive Mode -- Writing Your First Program -- Using the print Statement -- Learning the Jargon -- Generating an Error -- Understanding Color Coding -- Programming in Script Mode -- Writing Your First Program (Again) -- Saving and Running Your Program -- Back to the Game Over Program -- Using Comments -- Using Blank Lines -- Printing the String -- Waiting for the User -- Summary -- CHAPTER 2 Types, Variables, and Simple I/O: The Useless Trivia Program -- Introducing the Useless Trivia Program -- Using Quotes with Strings -- Introducing the Game Over 2.0 Program -- Using Quotes Inside Strings -- Continuing a Statement on the Next Line -- Creating Triple-Quoted Strings -- Using Escape Sequences with Strings -- Introducing the Fancy Credits Program -- Sounding the System Bell -- Moving Forward a Tab Stop -- Printing a Backslash -- Inserting a Newline -- Inserting a Quote -- Concatenating and Repeating Strings -- Introducing the Silly Strings Program -- Concatenating Strings -- Suppressing a Newline -- Repeating Strings -- Working with Numbers -- Introducing the Word Problems Program -- Understanding Numeric Types -- Using Mathematical Operators -- Understanding Variables -- Introducing the Greeter Program -- Creating Variables -- Using Variables.
Naming Variables -- Getting User Input -- Introducing the Personal Greeter Program -- Using the raw_input() Function -- Using String Methods -- Introducing the Quotation Manipulation Program -- Creating New Strings with String Methods -- Using the Right Types -- Introducing the Trust Fund Buddy-Bad Program -- Tracking Down Logical Errors -- Converting Values -- Introducing the Trust Fund Buddy-Good Program -- Converting Strings to Integers -- Using Augmented Assignment Operators -- Printing Strings and Numbers Together -- Back to the Useless Trivia Program -- Creating the Initial Comments -- Getting the User Input -- Printing Lowercase and Uppercase Versions of name -- Calculating dog_years -- Calculating seconds -- Printing name Five Times -- Calculating moon_weightand sun_weight -- Waiting for the User -- Summary -- CHAPTER 3 Branching, while Loops, and Program Planning: The Guess My Number Game -- Introducing the Guess My Number Game -- Generating Random Numbers -- Introducing the Craps Roller Program -- Using the import Statement -- Accessing randrange() -- Using randrange() -- Using the if Structure -- Introducing the Password Program -- Examining the if Structure -- Creating Conditions -- Understanding Comparison Operators -- Using Indentation to Create Blocks -- Building Your Own if Structure -- Using the if-else Structure -- Introducing the Granted or Denied Program -- Examining the else Statement -- Using the if-elif-else Structure -- Introducing the Mood Computer Program -- Examining the if-elif-else Structure -- Creating while Loops -- Introducing the Three-Year-Old Simulator Program -- Examining the while Structure -- Initializing the Sentry Variable -- Checking the Sentry Variable -- Updating the Sentry Variable -- Avoiding Infinite Loops -- Introducing the Losing Battle Program -- Tracing the Program.
Creating Conditions That Can Become False -- Treating Values as Conditions -- Introducing the Maitre D' Program -- Interpreting Any Value as True or False -- Creating Intentional Infinite Loops -- Introducing the Finicky Counter Program -- Understanding True and False -- Using the break Statement to Exit a Loop -- Using the continue Statement to Jump Back to the Top of a Loop -- Understanding When to Use break and continue -- Using Compound Conditions -- Introducing the Exclusive Network Program -- Understanding the not Logical Operator -- Understanding the and Logical Operator -- Understanding the or Logical Operator -- Planning Your Programs -- Creating Algorithms with Pseudocode -- Applying Stepwise Refinement to Your Algorithms -- Returning to the Guess My Number Game -- Planning the Program -- Creating the Initial Comment Block -- Importing the random Module -- Explaining the Game -- Setting the Initial Values -- Creating a Guessing Loop -- Congratulating the Player -- Waiting for the Player to Quit -- Summary -- CHAPTER 4 for Loops, Strings, and Tuples: The Word Jumble Game -- Introducing the Word Jumble Game -- Using for Loops -- Introducing the Loopy String Program -- Understanding for Loops -- Creating a for Loop -- Counting with a for Loop -- Introducing the Counter Program -- Counting Forwards -- Counting by Fives -- Counting Backwards -- Using Sequence Operators and Functions with Strings -- Introducing the Message Analyzer Program -- Using the len() Function -- Using the in Operator -- Indexing Strings -- Introducing the Random Access Program -- Working with Positive Position Numbers -- Working with Negative Position Numbers -- Accessing a Random String Element -- Understanding String Immutability -- Building a New String -- Introducing the No Vowels Program -- Creating Constants -- Creating New Strings from Existing Ones.
Slicing Strings -- Introducing the Pizza Slicer Program -- Introducing None -- Understanding Slicing -- Creating Slices -- Using Slicing Shorthand -- Creating Tuples -- Introducing the Hero's Inventory Program -- Creating an Empty Tuple -- Treating a Tuple as a Condition -- Creating a Tuple with Elements -- Printing a Tuple -- Looping Through a Tuple's Elements -- Using Tuples -- Introducing the Hero's Inventory 2.0 -- Setting Up the Program -- Using the len() Function with Tuples -- Using the in Operator with Tuples -- Indexing Tuples -- Slicing Tuples -- Understanding Tuple Immutability -- Concatenating Tuples -- Back to the Word Jumble Game -- Setting Up the Program -- Planning the Jumble Creation Section -- Creating an Empty Jumble String -- Setting Up the Loop -- Generating a Random Position in word -- Creating a New Version of jumble -- Creating a New Version of word -- Welcoming the Player -- Getting the Player's Guess -- Congratulating the Player -- Ending the Game -- Summary -- CHAPTER 5 Lists and Dictionaries: The Hangman Game -- Introducing the Hangman Game -- Using Lists -- Introducing the Hero's Inventory 3.0 Program -- Creating a List -- Using the len() Function with Lists -- Using the in Operator with Lists -- Indexing Lists -- Slicing Lists -- Concatenating Lists -- Understanding List Mutability -- Assigning a New List Element by Index -- Assigning a New List Slice -- Deleting a List Element -- Deleting a List Slice -- Using List Methods -- Introducing the High Scores Program -- Setting Up the Program -- Displaying the Menu -- Exiting the Program -- Displaying the Scores -- Adding a Score with the append() Function -- Removing a Score with the remove() Function -- Sorting the Scores with the sort() Function -- Reversing the Scores with the reverse() Function -- Dealing with an Invalid Choice -- Waiting for the User.
Understanding When to Use Tuples Instead of Lists -- Using Nested Sequences -- Introducing the High Scores 2.0 Program -- Creating Nested Sequences -- Accessing Nested Elements -- Unpacking a Sequence -- Setting Up the Program -- Displaying the Scores by Accessing Nested Tuples -- Adding a Score by Appending a Nested Tuple -- Dealing with an Invalid Choice -- Waiting for the User -- Understanding Shared References -- Using Dictionaries -- Introducing the Geek Translator Program -- Creating Dictionaries -- Accessing Dictionary Values -- Using a Key to Retrieve a Value -- Testing for a Key with the in Operator Before Retrieving a Value -- Using the get() Method to Retrieve a Value -- Setting Up the Program -- Getting a Value -- Adding a Key-Value Pair -- Replacing a Key-Value Pair -- Deleting a Key-Value Pair -- Wrapping Up the Program -- Understanding Dictionary Requirements -- Back to the Hangman Game -- Setting Up the Program -- Creating Constants -- Initializing the Variables -- Creating the Main Loop -- Getting the Player's Guess -- Checking the Guess -- Ending the Game -- Summary -- CHAPTER 6 Functions: The Tic-Tac-Toe Game -- Introducing the Tic-Tac-Toe Game -- Creating Functions -- Introducing the Instructions Program -- Defining a Function -- Documenting a Function -- Calling a Programmer-Created Function -- Understanding Abstraction -- Using Parameters and Return Values -- Introducing the Receive and Return Program -- Receiving Information through Parameters -- Returning Information through Return Values -- Understanding Encapsulation -- Receiving and Returning Values in the Same Function -- Understanding Software Reuse -- Using Keyword Arguments and Default Parameter Values -- Introducing the Birthday Wishes Program -- Using Positional Parameters and Positional Arguments -- Using Positional Parameters and Keyword Arguments.
Using Default Parameter Values.
Local Note:
Electronic reproduction. Ann Arbor, Michigan : ProQuest Ebook Central, 2017. Available via World Wide Web. Access may be limited to ProQuest Ebook Central affiliated libraries.
Genre:
Electronic Access:
Click to View