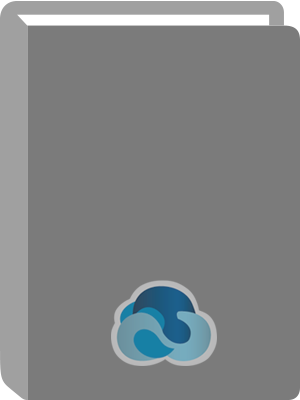
Beginning C++ Through Game Programming (3rd Edition).
Title:
Beginning C++ Through Game Programming (3rd Edition).
Author:
Dawson, Michael.
ISBN:
9781435457430
Personal Author:
Edition:
3rd ed.
Physical Description:
1 online resource (433 pages)
Contents:
Contents -- Introduction -- Chapter 1 Types, Variables, and Standard I/O: Lost Fortune -- Introducing C++ -- Using C++ for Games -- Creating an Executable File -- Dealing with Errors -- Understanding the ISO Standard -- Writing Your First C++ Program -- Introducing the Game Over Program -- Commenting Code -- Using Whitespace -- Including Other Files -- Defining the main() Function -- Displaying Text through the Standard Output -- Terminating Statements -- Returning a Value from main() -- Working with the std Namespace -- Introducing the Game Over 2.0 Program -- Employing a using Directive -- Introducing the Game Over 3.0 Program -- Employing using Declarations -- Understanding When to Employ using -- Using Arithmetic Operators -- Introducing the Expensive Calculator Program -- Adding, Subtracting, and Multiplying -- Understanding Integer and Floating Point Division -- Using the Modulus Operator -- Understanding Order of Operations -- Declaring and Initializing Variables -- Introducing the Game Stats Program -- Understanding Fundamental Types -- Understanding Type Modifiers -- Declaring Variables -- Naming Variables -- Assigning Values to Variables -- Initializing Variables -- Displaying Variable Values -- Getting User Input -- Defining New Names for Types -- Understanding Which Types to Use -- Performing Arithmetic Operations with Variables -- Introducing the Game Stats 2.0 Program -- Altering the Value of a Variable -- Using Combined Assignment Operators -- Using Increment and Decrement Operators -- Dealing with Integer Wrap Around -- Working with Constants -- Introducing the Game Stats 3.0 Program -- Using Constants -- Using Enumerations -- Introducing Lost Fortune -- Setting Up the Program -- Getting Information from the Player -- Telling the Story -- Summary -- Questions and Answers -- Discussion Questions -- Exercises.
Chapter 2 Truth, Branching, and the Game Loop: Guess My Number -- Understanding Truth -- Using the if Statement -- Introducing the Score Rater Program -- Testing true and false -- Interpreting a Value as true or false -- Using Relational Operators -- Nesting if Statements -- Using the else Clause -- Introducing the Score Rater 2.0 Program -- Creating Two Ways to Branch -- Using a Sequence of if Statements with else Clauses -- Introducing the Score Rater 3.0 Program -- Creating a Sequence of if Statements with else Clauses -- Using the switch Statement -- Introducing the Menu Chooser Program -- Creating Multiple Ways to Branch -- Using while Loops -- Introducing the Play Again Program -- Looping with a while Loop -- Using do Loops -- Introducing the Play Again 2.0 Program -- Looping with a do Loop -- Using break and continue Statements -- Introducing the Finicky Counter Program -- Creating a while (true) Loop -- Using the break Statement to Exit a Loop -- Using the continue Statement to Jump Back to the Top of a Loop -- Understanding When to Use break and continue -- Using Logical Operators -- Introducing the Designers Network Program -- Using the Logical AND Operator -- Using the Logical OR Operator -- Using the Logical NOT Operator -- Understanding Order of Operations -- Generating Random Numbers -- Introducing the Die Roller Program -- Calling the rand() Function -- Seeding the Random Number Generator -- Calculating a Number within a Range -- Understanding the Game Loop -- Introducing Guess My Number -- Applying the Game Loop -- Setting Up the Game -- Creating the Game Loop -- Wrapping Up the Game -- Summary -- Questions and Answers -- Discussion Questions -- Exercises -- Chapter 3 For Loops, Strings, and Arrays: Word Jumble -- Using for Loops -- Introducing the Counter Program -- Counting with for Loops -- Using Empty Statements in for Loops.
Nesting for Loops -- Understanding Objects -- Using String Objects -- Introducing the String Tester Program -- Creating string Objects -- Concatenating string Objects -- Using the size() Member Function -- Indexing a string Object -- Iterating through string Objects -- Using the find() Member Function -- Using the erase() Member Function -- Using the empty() Member Function -- Using Arrays -- Introducing the Hero's Inventory Program -- Creating Arrays -- Indexing Arrays -- Accessing Member Functions of an Array Element -- Being Aware of Array Bounds -- Understanding C-Style Strings -- Using Multidimensional Arrays -- Introducing the Tic-Tac-Toe Board Program -- Creating Multidimensional Arrays -- Indexing Multidimensional Arrays -- Introducing Word Jumble -- Setting Up the Program -- Picking a Word to Jumble -- Jumbling the Word -- Welcoming the Player -- Entering the Game Loop -- Saying Goodbye -- Summary -- Questions and Answers -- Discussion Questions -- Exercises -- Chapter 4 The Standard Template Library: Hangman -- Introducing the Standard Template Library -- Using Vectors -- Introducing the Hero's Inventory 2.0 Program -- Preparing to Use Vectors -- Declaring a Vector -- Using the push_back() Member Function -- Using the size() Member Function -- Indexing Vectors -- Calling Member Functions of an Element -- Using the pop_back() Member Function -- Using the clear() Member Function -- Using the empty() Member Function -- Using Iterators -- Introducing the Hero's Inventory 3.0 Program -- Declaring Iterators -- Looping through a Vector -- Changing the Value of a Vector Element -- Accessing Member Functions of a Vector Element -- Using the insert() Vector Member Function -- Using the erase() Vector Member Function -- Using Algorithms -- Introducing the High Scores Program -- Preparing to Use Algorithms -- Using the find() Algorithm.
Using the random_shuffle() Algorithm -- Using the sort() Algorithm -- Understanding Vector Performance -- Examining Vector Growth -- Examining Element Insertion and Deletion -- Examining Other STL Containers -- Planning Your Programs -- Using Pseudocode -- Using Stepwise Refinement -- Introducing Hangman -- Planning the Game -- Setting Up the Program -- Initializing Variables and Constants -- Entering the Main Loop -- Getting the Player's Guess -- Ending the Game -- Summary -- Questions and Answers -- Discussion Questions -- Exercises -- Chapter 5 Functions: Mad Lib -- Creating Functions -- Introducing the Instructions Program -- Declaring Functions -- Defining Functions -- Calling Functions -- Understanding Abstraction -- Using Parameters and Return Values -- Introducing the Yes or No Program -- Returning a Value -- Accepting Values into Parameters -- Understanding Encapsulation -- Understanding Software Reuse -- Working with Scopes -- Introducing the Scoping Program -- Working with Separate Scopes -- Working with Nested Scopes -- Using Global Variables -- Introducing the Global Reach Program -- Declaring Global Variables -- Accessing Global Variables -- Hiding Global Variables -- Altering Global Variables -- Minimizing the Use of Global Variables -- Using Global Constants -- Using Default Arguments -- Introducing the Give Me a Number Program -- Specifying Default Arguments -- Assigning Default Arguments to Parameters -- Overriding Default Arguments -- Overloading Functions -- Introducing the Triple Program -- Creating Overloaded Functions -- Calling Overloaded Functions -- Inlining Functions -- Introducing the Taking Damage Program -- Specifying Functions for Inlining -- Calling Inlined Functions -- Introducing the Mad Lib Game -- Setting Up the Program -- The main() Function -- The askText() Function -- The askNumber() Function.
The tellStory() Function -- Summary -- Questions and Answers -- Discussion Questions -- Exercises -- Chapter 6 References: Tic-Tac-Toe -- Using References -- Introducing the Referencing Program -- Creating References -- Accessing Referenced Values -- Altering Referenced Values -- Passing References to Alter Arguments -- Introducing the Swap Program -- Passing by Value -- Passing by Reference -- Passing References for Efficiency -- Introducing the Inventory Displayer Program -- Understanding the Pitfalls of Reference Passing -- Declaring Parameters as Constant References -- Passing a Constant Reference -- Deciding How to Pass Arguments -- Returning References -- Introducing the Inventory Referencer Program -- Returning a Reference -- Displaying the Value of a Returned Reference -- Assigning a Returned Reference to a Reference -- Assigning a Returned Reference to a Variable -- Altering an Object through a Returned Reference -- Introducing the Tic-Tac-Toe Game -- Planning the Game -- Setting Up the Program -- The main() Function -- The instructions() Function -- The askYesNo() Function -- The askNumber() Function -- The humanPiece() Function -- The opponent() Function -- The displayBoard() Function -- The winner() Function -- The isLegal() Function -- The humanMove() Function -- The computerMove() Function -- The announceWinner() Function -- Summary -- Questions and Answers -- Discussion Questions -- Exercises -- Chapter 7 Pointers: Tic-Tac-Toe 2.0 -- Understanding Pointer Basics -- Introducing the Pointing Program -- Declaring Pointers -- Initializing Pointers -- Assigning Addresses to Pointers -- Dereferencing Pointers -- Reassigning Pointers -- Using Pointers to Objects -- Understanding Pointers and Constants -- Using a Constant Pointer -- Using a Pointer to a Constant -- Using a Constant Pointer to a Constant -- Summarizing Constants and Pointers.
Passing Pointers.
Local Note:
Electronic reproduction. Ann Arbor, Michigan : ProQuest Ebook Central, 2017. Available via World Wide Web. Access may be limited to ProQuest Ebook Central affiliated libraries.
Genre:
Electronic Access:
Click to View