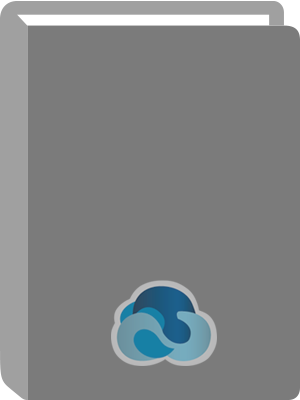
Computer Programming for Teens.
Title:
Computer Programming for Teens.
Author:
Farrell, Mary.
ISBN:
9781598636536
Personal Author:
Physical Description:
1 online resource (353 pages)
Contents:
Contents -- Introduction -- Chapter 1 First Things First -- In This Chapter -- Introduction -- Hardware and Software -- The Keyboard, Mouse, and Printer -- Hard Drive vs. External Drive -- RAM: Random Access Memory -- ROM: Read-Only Memory -- CPU: Central Processing Unit -- The ALU: Arithmetic/Logic Unit -- The Control Unit -- Digitization: Using Numbers to Represent Information -- The Computer: An Electronic Machine -- Electricity: On or Off -- Computer Languages -- Levels of Language: High and Low -- Language Helpers: Translators -- The Algorithm: The Basis for All Designs to Solutions of Programming Problems -- The Three Parts of Any Algorithm -- Examples of Algorithms in the Real World -- Programming -- Summary -- Chapter 2 Variables: The Holders of Information -- In This Chapter -- A Place to Put Data -- Examples Using Variables -- Comparison of Two Variables -- Different Types of Variables -- The Integer Type -- The Real Type -- The Character Type -- The String Type -- Introducing a Variable for the First Time in a Program -- An Analogy: Telling the Audience Who Is Who -- A Word about Statements -- Termination of Statements -- Must Statements Fit on One Line? -- Putting a Value into a Variable -- The Programmer -- The User -- How the Programmer Assigns Variables -- Assigning One Variable to Another Variable -- How the User Assigns Variables -- Input Streams -- Variables Are Assigned Their Values from This Stream -- A Stream Used for Input: cin -- Assigning Two Variables at Once -- Summary -- Chapter 3 Everything You Ever Wanted to Know About Operators -- In This Chapter -- What Are Operators Anyway? -- The Order of Operations -- Examples -- PEMDAS (Please Excuse My Dear Aunt Sally) -- Parentheses Still Rule -- Operators: Are They Binary or Unary? -- Binary Operators -- Unary Operators -- Arithmetic Operators -- Division: a Special Case.
Examples -- Examples with Variables -- The Relational Operators -- Examples -- Logic Operators -- Logic Operators in the Real World -- Logic Operators in the Computer Language Environment -- A Special Logic Operator: The Not Operator -- Examples -- A Powerful Operator for Any Computer Language: Mod -- How Is the Mod Operator Used in Programming? -- Summary -- Chapter 4 Programming: It's Now or Never -- In This Chapter -- Putting a Program Together -- Declare, Assign, and Manipulate -- Time for an Output Statement -- Cout -- How to Execute a Line Feed: endl -- Comments -- Compiler Directives -- The Include Directive -- The Main Section of a Program -- The Main Section -- The Return Statement -- Building a Program Outline -- Some Short Programs -- Example 1 -- Example 2 -- Example 3 -- Example 4 -- Summary -- Chapter 5 Design: It's Best to Start Here -- In This Chapter -- What Is Top-Down Design? -- Real-World Problems and Design -- Example 1 -- Example 2 -- Example 3 -- Example 4 -- Computer Problems and Design -- Example 1 -- Example 2 -- Summary -- Chapter 6 True or False: The Basis for All Decisions -- In This Chapter -- The Boolean Type -- What Does a Decision Involve? -- Developing a Model for Making a Decision -- Applying the Decision Model -- Controlling Where the Compiler Goes -- Program Flow -- Control Statements -- The If Statement -- The Boolean Condition -- The Conclusion -- Examples of If Statements in Everyday Circumstances -- Examples of If Statements for a Computer -- Examples Using the Relational Operators -- A Block of Code -- The If. . .Else Statement: The Two-Outcome Decision -- Examples -- Some Examples in Code -- Using a Boolean Condition to Determine Whether a Number Is Even or Odd -- The Switch/Case Statement -- Summary -- Chapter 7 Loops, or How to Spin Effectively! -- In This Chapter -- What Is a Loop? -- Loops in Programs.
Another Control Statement -- Repeating Steps in a Loop -- A Counter Statement -- Using the Counter Statement -- Two Different Kinds of Loops -- Fixed Iterative Loops vs. Conditional Loops -- The For Loop -- The Control Variable -- The Initial Statement -- The Boolean Expression -- The Counter Statement -- The Conditional Loop -- The While Loop -- The Do. . .While Loop -- Two Examples from a Programming Perspective -- Example 1 -- Example 2 -- Using a Conditional Loop with a Counter Statement -- Summary -- Chapter 8 Function Calls: That's What It's All About-Get Somebody Else to Do the Work -- In This Chapter -- What Is a Function and Why Use One? -- Example 1 -- Example 2 -- What Is a Function and What Does It Do? -- Example 1 -- Example 2 -- How Functions Interact with the Main -- Function Name and Parameter List -- Return Values -- How to Write a Function Heading -- Parameters: Two Different Types -- Value (Copy) Parameters -- Variable (Reference) Parameters -- The Symbol for a Variable (Reference) Parameter -- How to Call a Function -- Where the Calling Begins: Inside the Main Function -- The Receiving End: Inside the Called Function Find_Average -- Summary -- Chapter 9 Using Functions in Graphics -- In This Chapter -- Graphics: How Did You Do That? -- Vector Graphics -- Drawing Lines and Points -- Example 1 -- Example 2 -- Some Helpful Hints When Drawing -- Using the ''Pen'' to Draw -- Background vs. Foreground -- How to Make an Object Move -- Delaying the Computer -- Examples -- Using Functions to Draw -- Drawing the Sun By Using the Oval Commands -- Summary -- Chapter 10 Running Out of Holders? It's Time for the Array -- In This Chapter -- An Analogy for the Array Holder -- An Array Is Used for a Collection -- Why Is the Array Used? -- Array Syntax -- Different Types of Arrays -- Assigning Values to an Array -- How to Use Loops with Arrays.
Designing a For Loop with an Array in Mind -- Some Short Examples Using the Array -- Initializing an Array -- Printing an Array -- Counting the Number of Negative Values in an Array -- Copying One Array to Another -- Summary -- Chapter 11 All About Strings -- In This Chapter -- The String as an Array -- String Functions -- Length and Substring -- Find and CharAt -- The Brace Set Operator -- Summary -- Chapter 12 The Matrix-Before the Movie -- In This Chapter -- The Matrix as a Grid -- The Matrix -- How Does Storage Work? -- Loading One Row at a Time -- Nested For Loops -- The Bicycle Gear Analogy -- Applying the Loops -- How to Store Data in a Matrix -- How to Retrieve Data from a Matrix -- Manipulating a Matrix -- The Diagonal -- How to Change an Individual Row or Column of a Matrix -- Summary -- Chapter 13 Debugging: More Important Than You Think -- In This Chapter -- Three Types of Errors -- Syntax Errors -- Run-Time Errors -- Logic Errors -- What Are Debuggers? -- Tracing Through a Loop -- Tracing Through a Function -- Summary -- Chapter 14 But What If Things Are Different? Structures, Records, and Fields -- In This Chapter -- Beyond the Array: The Record -- How to Design a Record -- Defining a Record -- Reserved Words -- Declaring One or More Records -- Distinguishing Among the Record's Type, the Record Variable, and the Fields -- How to Assign Record Variables -- Using a Delimiter -- Letting the User Assign a Record -- Examples of Different Records -- An Array of Records -- Defining an Array of Records -- Where to Place the Record Definition -- Global Variables, Local Variables, and Their Scope -- Summary -- Chapter 15 Objects and Classes: Being Organized Is Better Than Not -- In This Chapter -- The Object -- Example -- Object-Oriented Programming -- Privacy of the Object -- The Class -- Constructors -- Accessors -- Modifiers.
How an Object Calls Its Methods -- Calling from Outside the Class -- Calling from Inside the Class -- Summary -- Chapter 16 Dealing with the Outside World: Files -- In This Chapter -- The Outside World from a Program's Perspective -- Creating a Text File -- Organizing a File Through Markers -- The End of Line Marker -- The End of File Marker -- Reading from a Text File -- Streams Used with Files -- How to Access the Values in a Text File -- Accessing the Contents and Displaying Them on the Screen at the Same Time -- Some Elementary Commands Used with Files -- How to ''Rewind'' a File -- Writing Values to a File -- Adding Values to the End of a File -- Closing a File -- Summary -- Chapter 17 Pointers: Who Is Looking at Whom? -- In This Chapter -- Static vs. Dynamic Variables -- Static and Dynamic Variables-Their Role in Memory -- The Pointer Variable: A Dynamic Variable -- How a Pointer (Variable) Works -- Operations Associated with Pointers -- Declaring a Pointer -- Allocating Memory for a Pointer -- Assigning the Variable at Which the Pointer ''Looks'' -- Distinguishing Between the Pointer and the Referred Variable -- How to Assign the Pointer Another Pointer -- De-allocating Memory -- Summary -- Chapter 18 Searching: Did You Find It Yet? -- In This Chapter -- Searching -- Searching for a Value in the Array -- Searching for a Value Not in the Array -- Developing the Linear Search Algorithm -- How to Examine a Member -- Writing a Loop to Examine Each Member -- Developing an If Statement with the Relational Expression -- The Binary Search -- Ascending Order -- What Is a Binary Search? -- Understanding the Binary Search -- Examining the Middle of a List -- Moving to the Right or to the Left -- How the Binary Search Works with an Array -- Finding the Middle of the Array -- How to ''Eliminate'' Half of the Array -- Summary.
Chapter 19 Let's Put Things in Order: Sorting.
Local Note:
Electronic reproduction. Ann Arbor, Michigan : ProQuest Ebook Central, 2017. Available via World Wide Web. Access may be limited to ProQuest Ebook Central affiliated libraries.
Genre:
Electronic Access:
Click to View