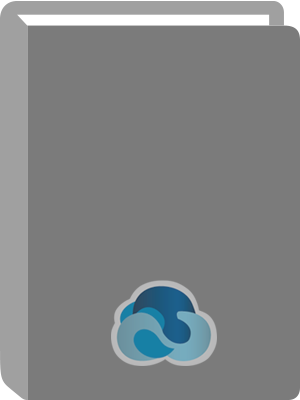
.NET 4.5 Parallel Extensions.
Title:
.NET 4.5 Parallel Extensions.
Author:
Freeman, Bryan.
ISBN:
9781849690232
Personal Author:
Edition:
1st ed.
Physical Description:
1 online resource (366 pages)
Contents:
.NET 4.5 Parallel Extensions Cookbook -- Table of Contents -- .NET 4.5 Parallel Extensions Cookbook -- Credits -- About the Author -- About the Reviewers -- www.PacktPub.com -- Support files, eBooks, discount offers and more -- Why Subscribe? -- Free Access for Packt account holders -- Preface -- What this book covers -- What you need for this book -- Who this book is for -- Conventions -- Reader feedback -- Customer support -- Downloading the example code -- Errata -- Piracy -- Questions -- 1. Getting Started with Task Parallel Library -- Introduction -- Creating a task -- How to do it… -- How it works… -- Waiting for tasks to finish -- How to do it… -- How it works… -- Returning results from a task -- How to do it… -- How it works… -- Passing data to a task -- How to do it… -- How it works… -- Creating a child task -- How to do it… -- How it works… -- Lazy task execution -- How to do it… -- How it works… -- Handling task exceptions using try/catch block -- Getting ready… -- How to do it… -- How it works… -- Handling task exceptions with AggregateException.Handle -- Getting ready… -- How to do it… -- How it works… -- Cancelling a task -- How to do it… -- How it works… -- There's more… -- Cancelling one of many tasks -- How to do it… -- How it works… -- 2. Implementing Continuations -- Introduction -- Continuing a task -- How to do it… -- How it works… -- Passing task results to a continuation -- How to do it… -- How it works… -- Continue "WhenAny" and "WhenAll" -- How to do it… -- How it works… -- Specifying when a continuation will run -- How to do it… -- How it works… -- There's more… -- Using a continuation for exception handling -- Getting Ready -- How to do it… -- How it works… -- Cancelling a continuation -- Getting Ready -- How to do it… -- How it works… -- Using a continuation to chain multiple tasks -- How to do it… -- How it works….
Using a continuation to update a UI -- How to do it… -- How it works… -- 3. Learning Concurrency with Parallel Loops -- Introduction -- Creating a basic parallel for loop -- How to do it… -- How it works… -- Creating a basic parallel foreach loop -- How to do it… -- How it works… -- Breaking a parallel loop -- How to do it… -- How it works… -- Stopping a parallel loop -- How to do it… -- How it works… -- Cancelling a parallel loop -- How to do it… -- How it works… -- Handling exceptions in a parallel loop -- Getting ready… -- How to do it… -- How it works… -- Controlling the degree of parallelism in a loop -- How to do it… -- How it works… -- Partitioning data in a parallel loop -- How to do it… -- How it works… -- Using Thread Local Storage -- How to do it… -- How it works… -- 4. Parallel LINQ -- Introduction -- Creating a basic parallel query -- How to do it… -- How it works… -- Preserving order in parallel LINQ -- How to do it… -- How it works… -- Forcing parallel execution -- How to do it… -- How it works… -- Limiting parallelism in a query -- How to do it… -- How it works… -- Processing query results -- How to do it… -- How it works… -- Specifying merge options -- How to do it… -- How it works… -- Range projection with parallel LINQ -- How to do it… -- How it works… -- Handling exceptions in parallel LINQ -- Getting ready… -- How to do it… -- How it works… -- Cancelling a parallel LINQ query -- Getting ready… -- How to do it… -- How it works… -- Performing reduction operations -- How to do it… -- How it works… -- Creating a custom partitioner -- How to do it… -- How it works… -- 5. Concurrent Collections -- Introduction -- Adding and removing items to BlockingCollection -- How to do it… -- How it works… -- Iterating a BlockingCollection with GetConsumingEnumerable -- How to do it… -- How it works….
Performing LIFO operations with ConcurrentStack -- How to do it… -- How it works… -- Thread safe data lookups with ConcurrentDictionary -- How to do it… -- How it works… -- Cancelling an operation in a concurrent collection -- Getting ready… -- How to do it… -- How it works… -- Working with multiple producers and consumers -- How to do it… -- How it works… -- Creating object pool with ConcurrentStack -- How to do it… -- How it works… -- Adding blocking and bounding with IProducerConsumerCollection -- How to do it… -- How it works… -- Using multiple concurrent collections to create a pipeline -- How to do it… -- How it works… -- 6. Synchronization Primitives -- Introduction -- Using monitor -- How to do it… -- How it works… -- Using a mutual exclusion lock -- How to do it… -- How it works… -- Using SpinLock for synchronization -- How to do it… -- How it works… -- Interlocked operations -- How to do it… -- How it works… -- Synchronizing multiple tasks with a Barrier -- How to do it… -- How it works… -- Using ReaderWriterLockSlim -- How to do it… -- How it works… -- Using WaitHandles with Mutex -- How to do it… -- How it works… -- Waiting for multiple threads with CountdownEvent -- How to do it… -- How it works… -- Using ManualResetEventSlim to spin and wait -- How to do it… -- How it works… -- Using SemaphoreSlim to limit access -- How to do it… -- How it works… -- 7. Profiling and Debugging -- Introduction -- Using the Threads and Call Stack windows -- Getting ready… -- How to do it… -- Using the Parallel Stacks window -- How to do it… -- Watching values in a thread with Parallel Watch window -- How to do it… -- Detecting deadlocks with the Parallel Tasks window -- Getting ready… -- How to do it… -- Measure CPU utilization with Concurrency Visualizer -- Getting ready… -- How to do it… -- Using Concurrency Visualizer Threads view -- Getting ready….
How to do it… -- Using Concurrency Visualizer Cores view -- Getting ready… -- How to do it… -- 8. Async -- Introduction -- Creating an async method -- How to do it… -- How it works… -- Handling Exceptions in asynchronous code -- How to do it… -- How it works… -- Cancelling an asynchronous operation -- How to do it… -- How it works… -- Cancelling async operation after timeout period -- How to do it… -- How it works… -- Processing multiple async tasks as they complete -- How to do it… -- How it works… -- Improving performance of async solution with Task.WhenAll -- How to do it… -- How it works… -- Using async for file access -- How to do it… -- How it works… -- Checking the progress of an asynchronous task -- How to do it… -- How it works… -- 9. Dataflow Library -- Introduction -- Reading from and writing to a dataflow block synchronously -- Getting ready… -- How to do it… -- How it works… -- Reading from and writing to a dataflow block asynchronously -- How to do it… -- How it works… -- Implementing a producer-consumer dataflow pattern -- How to do it… -- How it works… -- Creating a dataflow pipeline -- How to do it… -- How it works… -- Cancelling a dataflow block -- How to do it… -- How it works… -- Specifying the degree of parallelism -- How to do it… -- How it works… -- Unlink dataflow blocks -- How to do it… -- How it works… -- Using JoinBlock to read from multiple data sources -- How to do it… -- How it works… -- Index.
Abstract:
This book contains practical recipes on everything you will need to create task-based parallel programs using C#, .NET 4.5, and Visual Studio. The book is packed with illustrated code examples to create scalable programs.This book is intended to help experienced C# developers write applications that leverage the power of modern multicore processors. It provides the necessary knowledge for an experienced C# developer to work with .NET parallelism APIs. Previous experience of writing multithreaded applications is not necessary.
Local Note:
Electronic reproduction. Ann Arbor, Michigan : ProQuest Ebook Central, 2017. Available via World Wide Web. Access may be limited to ProQuest Ebook Central affiliated libraries.
Genre:
Electronic Access:
Click to View