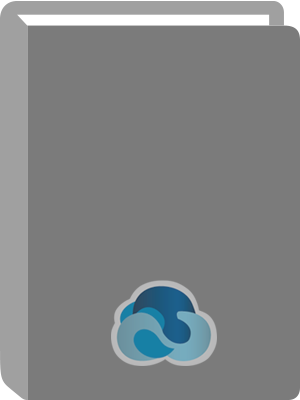
Professional C# 2012 and .NET 4.5.
Title:
Professional C# 2012 and .NET 4.5.
Author:
Nagel, Christian.
ISBN:
9781118332122
Personal Author:
Edition:
1st ed.
Physical Description:
1 online resource (1882 pages)
Contents:
Professional C# 2012 and .NET 4.5 -- Copyright -- About the Authors -- About the Technical Editors -- Credits -- Acknowledgments -- Contents -- Introduction -- The Significance of .NET and C# -- Advantages of .NET -- What's New in the .NET Framework 4.5 -- Asynchronous Programming -- Windows Store Apps and the Windows Runtime -- Enhancements with Data Access -- Enhancements with WPF -- ASP.NET MVC -- Where C# Fits in -- What You Need To Write and Run C# Code -- What this Book Covers -- Part I: The C# Language -- Part II: Visual Studio -- Part III: Foundation -- Part IV: Data -- Part V: Presentation -- Part VI: Communication -- Conventions -- Source Code -- Errata -- P2P.Wrox.Com -- Part I: The C# Language -- Chapter 1: .NET Architecture -- The Relationship of C# to .NET -- The Common Language Runtime -- Platform Independence -- Performance Improvement -- Language Interoperability -- A Closer Look at Intermediate Language -- Support for Object Orientation and Interfaces -- Distinct Value and Reference Types -- Strong Data Typing -- Error Handling with Exceptions -- Use of Attributes -- Assemblies -- Private Assemblies -- Shared Assemblies -- Reflection -- Parallel Programming -- Asynchronous Programming -- .NET Framework Classes -- Namespaces -- Creating .NET Applications Using C# -- Creating ASP.NET Applications -- Windows Presentation Foundation (WPF) -- Windows 8 Apps -- Windows Services -- Windows Communication Foundation -- Windows Workflow Foundation -- The Role of C# in the .NET Enterprise Architecture -- Summary -- Chapter 2: Core C# -- Fundamental C# -- Your First C# Program -- The Code -- Compiling and Running the Program -- A Closer Look -- Variables -- Initialization of Variables -- Type Inference -- Variable Scope -- Constants -- Predefined Data Types -- Value Types and Reference Types -- CTS Types -- Predefined Value Types.
Predefined Reference Types -- Flow Control -- Conditional Statements -- Loops -- Jump Statements -- Enumerations -- Namespaces -- The using Directive -- Namespace Aliases -- The Main() Method -- Multiple Main() Methods -- Passing Arguments to Main() -- More on Compiling C# Files -- Console I/O -- Using Comments -- Internal Comments within the Source Files -- XML Documentation -- The C# Preprocessor Directives -- #define and #undef -- #if, #elif, #else, and #endif -- #warning and #error -- #region and #endregion -- #line -- #pragma -- C# Programming Guidelines -- Rules for Identifiers -- Usage Conventions -- Summary -- Chapter 3: Objects and Types -- Creating and Using Classes -- Classes and Structs -- Classes -- Data Members -- Function Members -- readonly Fields -- Anonymous Types -- Structs -- Structs Are Value Types -- Structs and Inheritance -- Constructors for Structs -- Weak References -- Partial Classes -- Static Classes -- The Object Class -- System.Object Methods -- The ToString() Method -- Extension Methods -- Summary -- Chapter 4: Inheritance -- Inheritance -- Types of Inheritance -- Implementation Versus Interface Inheritance -- Multiple Inheritance -- Structs and Classes -- Implementation Inheritance -- Virtual Methods -- Hiding Methods -- Calling Base Versions of Functions -- Abstract Classes and Functions -- Sealed Classes and Methods -- Constructors of Derived Classes -- Modifiers -- Visibility Modifiers -- Other Modifiers -- Interfaces -- Defining and Implementing Interfaces -- Derived Interfaces -- Summary -- Chapter 5: Generics -- Generics Overview -- Performance -- Type Safety -- Binary Code Reuse -- Code Bloat -- Naming Guidelines -- Creating Generic Classes -- Generics Features -- Default Values -- Constraints -- Inheritance -- Static Members -- Generic Interfaces -- Covariance and Contra-variance.
Covariance with Generic Interfaces -- Contra-Variance with Generic Interfaces -- Generic Structs -- Generic Methods -- Generic Methods Example -- Generic Methods with Constraints -- Generic Methods with Delegates -- Generic Methods Specialization -- Summary -- Chapter 6: Arrays and Tuples -- Multiple Objects of the Same and Different Types -- Simple Arrays -- Array Declaration -- Array Initialization -- Accessing Array Elements -- Using Reference Types -- Multidimensional Arrays -- Jagged Arrays -- Array Class -- Creating Arrays -- Copying Arrays -- Sorting -- Arrays as Parameters -- Array Covariance -- ArraySegment -- Enumerations -- IEnumerator Interface -- foreach Statement -- yield Statement -- Tuples -- Structural Comparison -- Summary -- Chapter 7: Operators and Casts -- Operators and Casts -- Operators -- Operator Shortcuts -- Operator Precedence -- Type Safety -- Type Conversions -- Boxing and Unboxing -- Comparing Objects for Equality -- Comparing Reference Types for Equality -- Comparing Value Types for Equality -- Operator Overloading -- How Operators Work -- Operator Overloading Example: The Vector Struct -- Which Operators Can You Overload? -- User-Defined Casts -- Implementing User-Defined Casts -- Multiple Casting -- Summary -- Chapter 8: Delegates, Lambdas, and Events -- Referencing Methods -- Delegates -- Declaring Delegates -- Using Delegates -- Simple Delegate Example -- Action and Func Delegates -- BubbleSorter Example -- Multicast Delegates -- Anonymous Methods -- Lambda Expressions -- Parameters -- Multiple Code Lines -- Closures -- Closures with Foreach Statements -- Events -- Event Publisher -- Event Listener -- Weak Events -- Summary -- Chapter 9: Strings and Regular Expressions -- Examining System.String -- Building Strings -- StringBuilder Members -- Format Strings -- Regular Expressions.
Introduction to Regular Expressions -- The RegularExpressionsPlayaround Example -- Displaying Results -- Matches, Groups, and Captures -- Summary -- Chapter 10: Collections -- Overview -- Collection Interfaces and Types -- Lists -- Creating Lists -- Read-Only Collections -- Queues -- Stacks -- Linked Lists -- Sorted List -- Dictionaries -- Key Type -- Dictionary Example -- Lookups -- Sorted Dictionaries -- Sets -- Observable Collections -- Bit Arrays -- BitArray -- BitVector32 -- Concurrent Collections -- Creating Pipelines -- Using BlockingCollection -- Using ConcurrentDictionary -- Completing the Pipeline -- Performance -- Summary -- Chapter 11: Language Integrated Query -- LINQ Overview -- Lists and Entities -- LINQ Query -- Extension Methods -- Deferred Query Execution -- Standard Query Operators -- Filtering -- Filtering with Index -- Type Filtering -- Compound from -- Sorting -- Grouping -- Grouping with Nested Objects -- Inner Join -- Left Outer Join -- Group Join -- Set Operations -- Zip -- Partitioning -- Aggregate Operators -- Conversion Operators -- Generation Operators -- Parallel LINQ -- Parallel Queries -- Partitioners -- Cancellation -- Expression Trees -- LINQ Providers -- Summary -- Chapter 12: Dynamic Language Extensions -- Dynamic Language Runtime -- The Dynamic Type -- Dynamic Behind the Scenes -- Hosting the DLR ScriptRuntime -- DynamicObject and ExpandoObject -- DynamicObject -- ExpandoObject -- Summary -- Chapter 13: Asynchronous Programming -- Why Asynchronous Programming Is Important -- Asynchronous Patterns -- Synchronous Call -- Asynchronous Pattern -- Event-Based Asynchronous Pattern -- Task-Based Asynchronous Pattern -- Foundation of Asynchronous Programming -- Creating Tasks -- Calling an Asynchronous Method -- Continuation with Tasks -- Synchronization Context -- Using Multiple Asynchronous Methods.
Converting the Asynchronous Pattern -- Error Handling -- Handling Exceptions with Asynchronous Methods -- Exceptions with Multiple Asynchronous Methods -- Using AggregateException Information -- Cancellation -- Starting a Cancellation -- Cancellation with Framework Features -- Cancellation with Custom Tasks -- Summary -- Chapter 14: Memory Management and Pointers -- Memory Management -- Memory Management Under the Hood -- Value Data Types -- Reference Data Types -- Garbage Collection -- Freeing Unmanaged Resources -- Destructors -- The IDisposable Interface -- Implementing IDisposable and a Destructor -- Unsafe Code -- Accessing Memory Directly with Pointers -- Pointer Example: PointerPlayground -- Using Pointers to Optimize Performance -- Summary -- Chapter 15: Reflection -- Manipulating and Inspecting Code at Runtime -- Custom Attributes -- Writing Custom Attributes -- Custom Attribute Example: WhatsNewAttributes -- Using Reflection -- The System.Type Class -- The TypeView Example -- The Assembly Class -- Completing the WhatsNewAttributes Example -- Summary -- Chapter 16: Errors and Exceptions -- Introduction -- Exception Classes -- Catching Exceptions -- Implementing Multiple Catch Blocks -- Catching Exceptions from Other Code -- System.Exception Properties -- What Happens If an Exception Isn't Handled? -- Nested try Blocks -- User-Defined Exception Classes -- Catching the User-Defined Exceptions -- Throwing the User-Defined Exceptions -- Defining the User-Defined Exception Classes -- Caller Information -- Summary -- Part II: Visual Studio -- Chapter 17: Visual Studio 2012 -- Working with Visual Studio 2012 -- Project File Changes -- Visual Studio Editions -- Visual Studio Settings -- Creating a Project -- Multi-Targeting the .NET Framework -- Selecting a Project Type -- Exploring and Coding a Project -- Solution Explorer.
Working with the Code Editor.
Abstract:
Intermediate to advanced technique coverage, updated for C# 2012 and .NET 4.5 This guide is geared towards experienced programmers looking to update and enhance their skills in writing Windows applications, web apps, and Metro apps with C# and .NET 4.5. Packed with information about intermediate and advanced features, this book includes everything professional developers need to know about C# and putting it to work. Covers challenging .NET features including Language Integrated Query (LINQ), LINQ to SQL, LINQ to XML, WCF, WPF, Workflow, and Generics Puts the new Async keyword to work and features refreshers on .NET architecture, objects, types, inheritance, arrays, operators, casts, delegates, events, strings, regular expressions, collections, and memory management Explores new options and interfaces presented by Windows 8 development, WinRT, and Metro style apps Includes traditional Windows forms programming, ASP.NET web programming with C#, and working in Visual Studio 2012 with C# Professional C# 2012 and .NET 4.5 is a comprehensive guide for experienced programmers wanting to maximize these technologies.
Local Note:
Electronic reproduction. Ann Arbor, Michigan : ProQuest Ebook Central, 2017. Available via World Wide Web. Access may be limited to ProQuest Ebook Central affiliated libraries.
Genre:
Electronic Access:
Click to View