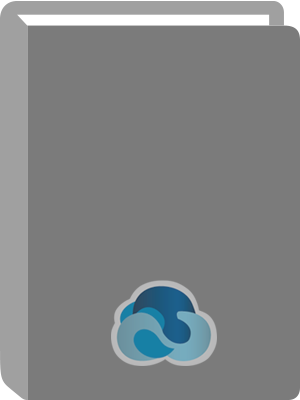
Professional ASP. NET MVC 5.
Title:
Professional ASP. NET MVC 5.
Author:
Galloway, Jon.
ISBN:
9781118794722
Personal Author:
Edition:
1st ed.
Physical Description:
1 online resource (622 pages)
Contents:
Cover -- Title Page -- Copyright -- Contents -- Chapter 1 Getting Started -- A Quick Introduction to ASP.NET MVC -- How ASP.NET MVC Fits in with ASP.NET -- The MVC Pattern -- MVC as Applied to Web Frameworks -- The Road to MVC 5 -- MVC 4 Overview -- Open-Source Release -- ASP.NET MVC 5 Overview -- One ASP.NET -- New Web Project Experience -- ASP.NET Identity -- Bootstrap Templates -- Attribute Routing -- ASP.NET Scaffolding -- Authentication Filters -- Filter Overrides -- Installing MVC 5 and Creating Applications -- Software Requirements for ASP.NET MVC 5 -- Installing ASP.NET MVC 5 -- Creating an ASP.NET MVC 5 Application -- The New ASP.NET Project Dialog -- The MVC Application Structure -- ASP.NET MVC and Conventions -- Convention over Configuration -- Conventions Simplify Communication -- Summary -- Chapter 2 Controllers -- The Controller's Role -- A Sample Application: The MVC Music Store -- Controller Basics -- A Simple Example: The Home Controller -- Writing Your First Controller -- Parameters in Controller Actions -- Summary -- Chapter 3 Views -- The Purpose of Views -- View Basics -- Understanding View Conventions -- Strongly Typed Views -- How ViewBag Falls Short -- Understanding ViewBag, ViewData, and ViewDataDictionary -- View Models -- Adding a View -- The Razor View Engine -- What Is Razor? -- Code Expressions -- HTML Encoding -- Code Blocks -- Razor Syntax Samples -- Layouts -- ViewStart -- Specifying a Partial View -- Summary -- Chapter 4 Models -- Modeling the Music Store -- Scaffolding a Store Manager -- What Is Scaffolding? -- Scaffolding and the Entity Framework -- Executing the Scaffolding Template -- Executing the Scaffolded Code -- Editing an Album -- Building a Resource to Edit an Album -- Responding to the Edit POST Request -- Model Binding -- The DefaultModelBinder -- Explicit Model Binding -- Summary.
Chapter 5 Forms and HTML Helpers -- Using Forms -- The Action and the Method -- To GET or to POST? -- HTML Helpers -- Automatic Encoding -- Making Helpers Do Your Bidding -- Inside HTML Helpers -- Setting Up the Album Edit Form -- Adding Inputs -- Helpers, Models, and View Data -- Strongly Typed Helpers -- Helpers and Model Metadata -- Templated Helpers -- Helpers and ModelState -- Other Input Helpers -- Html.Hidden -- Html.Password -- Html.RadioButton -- Html.CheckBox -- Rendering Helpers -- Html.ActionLink and Html.RouteLink -- URL Helpers -- Html.Partial and Html.RenderPartial -- Html.Action and Html.RenderAction -- Summary -- Chapter 6 Data Annotations and Validation -- Annotating Orders for Validation -- Using Validation Annotations -- Custom Error Messages and Localization -- Looking Behind the Annotation Curtain -- Controller Actions and Validation Errors -- Custom Validation Logic -- Custom Annotations -- IValidatableObject -- Display and Edit Annotations -- Display -- ScaffoldColumn -- DisplayFormat -- ReadOnly -- DataType -- UIHint -- HiddenInput -- Summary -- Chapter 7 Membership, Authorization, and Security -- Security: Not fun, But Incredibly Important -- Using the Authorize Attribute to Require Login -- Securing Controller Actions -- How AuthorizeAttribute Works with Forms Authentication and the AccountController -- Windows Authentication -- Using AuthorizeAttribute to Require Role Membership -- Extending User Identity -- Storing additional user profile data -- Persistance control -- Managing users and roles -- External Login via OAuth and OpenID -- Registering External Login Providers -- Configuring OpenID Providers -- Configuring OAuth Providers -- Security Implications of External Logins -- Understanding the Security Vectors in a Web Application -- Threat: Cross-Site Scripting.
Threat: Cross-Site Request Forgery -- Threat: Cookie Stealing -- Threat: Over-Posting -- Threat: Open Redirection -- Proper Error Reporting and the Stack Trace -- Using Configuration Transforms -- Using Retail Deployment Configuration in Production -- Using a Dedicated Error Logging System -- Security Recap and Helpful Resources -- Summary -- Chapter 8 Ajax -- jQuery -- jQuery Features -- Unobtrusive JavaScript -- Using jQuery -- Ajax Helpers -- Adding the Unobtrusive Ajax Script to Your Project -- Ajax ActionLinks -- HTML 5 Attributes -- Ajax Forms -- Client Validation -- jQuery Validation -- Custom Validation -- Beyond Helpers -- jQuery UI -- Autocomplete with jQuery UI -- JSON and Client-Side Templates -- Bootstrap Plugins -- Improving Ajax Performance -- Using Content Delivery Networks -- Script Optimizations -- Bundling and Minification -- Summary -- Chapter 9 Routing -- Uniform Resource Locators -- Introduction to Routing -- Comparing Routing to URL Rewriting -- Routing Approaches -- Defining Attribute Routes -- Defining Traditional Routes -- Choosing Attribute Routes or Traditional Routes -- Named Routes -- MVC Areas -- Catch-All Parameter -- Multiple Route Parameters in a Segment -- StopRoutingHandler and IgnoreRoute -- Debugging Routes -- Inside Routing: How Routes Generate URLs -- High-Level View of URL Generation -- A Detailed Look at URL Generation -- Ambient Route Values -- More Examples of URL Generation with the Route Class -- Inside Routing: How Routes Tie Your URL to an Action -- The High-Level Request Routing Pipeline -- RouteData -- Custom Route Constraints -- Using Routing with Web Forms -- Summary -- Chapter 10 NuGet -- Introduction to NuGet -- Adding a Library as a Package -- Finding Packages -- Installing a Package -- Updating a Package -- Package Restore.
Using the Package Manager Console -- Creating Packages -- Packaging a Project -- Packaging a Folder -- Configuration File and Source Code Transformations -- NuSpec File -- Metadata -- Dependencies -- Specifying Files to Include -- Tools -- Framework and Profile Targeting -- Prerelease Packages -- Publishing Packages -- Publishing to NuGet.org -- Using NuGet.exe -- Using the Package Explorer -- Summary -- Chapter 11 ASP.NET Web API -- Defining ASP.NET Web API -- Getting Started with Web API -- Writing an API Controller -- Examining the Sample ValuesController -- Async by Design: IHttpController -- Incoming Action Parameters -- Action Return Values, Errors, and Asynchrony -- Configuring Web API -- Configuration in Web-Hosted Web API -- Configuration in Self-Hosted Web API -- Adding Routes to Your Web API -- Binding Parameters -- Filtering Requests -- Enabling Dependency Injection -- Exploring APIs Programmatically -- Tracing the Application -- Web API Example: ProductsController -- Summary -- Chapter 12 Single Page Applications with AngularJS -- Understanding and Setting Up AngularJS -- What's AngularJS? -- Your Goal in This Chapter -- Getting Started -- Adding AngularJS to the Site -- Setting Up the Database -- Building the Web API -- Building Applications and Modules -- Creating Controllers, Models, and Views -- Services -- Routing -- Details View -- A Custom MovieService -- Deleting Movies -- Editing and Creating Movies -- Summary -- Chapter 13 Dependency Injection -- Software Design Patterns -- Design Pattern: Inversion of Control -- Design Pattern: Service Locator -- Design Pattern: Dependency Injection -- Dependency Resolution in MVC -- Singly Registered Services in MVC -- Multiply Registered Services in MVC -- Arbitrary Objects in MVC -- Dependency Resolution in Web API.
Singly Registered Services in Web API -- Multiply Registered Services in Web API -- Arbitrary Objects in Web API -- Dependency Resolvers in MVC vs. Web API -- Summary -- Chapter 14 Unit Testing -- Understanding Unit Testing and Test-Driven Development -- Defining Unit Testing -- Defining Test-Driven Development -- Building a Unit Test Project -- Examining the Default Unit Tests -- Test Only the Code You Write -- Advice for Unit Testing Your ASP.NET MVC and ASP.NET Web API Applications -- Testing Controllers -- Testing Routes -- Testing Validators -- Summary -- Chapter 15 Extending MVC -- Extending Models -- Turning Request Data into Models -- Describing Models with Metadata -- Validating Models -- Extending Views -- Customizing View Engines -- Writing HTML Helpers -- Writing Razor Helpers -- Extending Controllers -- Selecting Actions -- Filters -- Providing Custom Results -- Summary -- Chapter 16 Advanced Topics -- Mobile Support -- Adaptive Rendering -- Display Modes -- Advanced Razor -- Templated Razor Delegates -- View Compilation -- Advanced View Engines -- Configuring a View Engine -- Finding a View -- The View Itself -- Alternative View Engines -- New View Engine or New ActionResult? -- Advanced Scaffolding -- Introducing ASP.NET Scaffolding -- Customizing Scaffold Templates -- Custom Scaffolders -- Advanced Routing -- RouteMagic -- Editable Routes -- Advanced Templates -- The Default Templates -- Custom Templates -- Advanced Controllers -- Defining the Controller: The IController Interface -- The ControllerBase Abstract Base Class -- The Controller Class and Actions -- Action Methods -- The ActionResult -- Action Invoker -- Using Asynchronous Controller Actions -- Summary -- Chapter 17 Real-World ASP.NET MVC: Building the NuGet.org Website -- May the Source Be with You -- WebActivator -- ASP.NET Dynamic Data.
Exception Logging.
Abstract:
ASP.NET MVC insiders cover the latest updates to the technology in this popular Wrox reference MVC 5 is the newest update to the popular Microsoft technology that enables you to build dynamic, data-driven websites. Like previous versions, this guide shows you step-by-step techniques on using MVC to best advantage, with plenty of practical tutorials to illustrate the concepts. It covers controllers, views, and models; forms and HTML helpers; data annotation and validation; membership, authorization, and security. MVC 5, the latest version of MVC, adds sophisticated features such as single page applications, mobile optimization, and adaptive rendering A team of top Microsoft MVP experts, along with visionaries in the field, provide practical advice on basic and advanced MVC topics Covers controllers, views, models, forms, data annotations, authorization and security, Ajax, routing, ASP.NET web API, dependency injection, unit testing, real-world application, and much more Professional ASP.NET MVC 5 is the comprehensive resource you need to make the best use of the updated Model-View-Controller technology.
Local Note:
Electronic reproduction. Ann Arbor, Michigan : ProQuest Ebook Central, 2017. Available via World Wide Web. Access may be limited to ProQuest Ebook Central affiliated libraries.
Genre:
Electronic Access:
Click to View