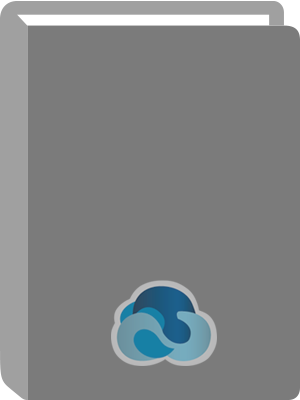
Game Coding Complete.
Title:
Game Coding Complete.
Author:
McShaffry, Mike.
ISBN:
9781133776581
Personal Author:
Edition:
4th ed.
Physical Description:
1 online resource (959 pages)
Contents:
Cover -- Contents -- Introduction -- Chapter 1 What Is Game Programming Really Like? -- The Good -- The Job -- The Gamers -- Your Coworkers -- The Tools-Software Development Kits (SDKs) -- The Hardware -- The Platforms -- The Show -- The Hard Work -- Game Programming Is Freaking Hard -- Bits and Pieces -- That's Not a Bug-That's a Feature -- The Tools -- The Dark Side -- Hitting a Moving Target -- Crunch Mode (and Crunch Meals) -- Bah Humbug -- Operating System Hell -- Fluid Nature of Employment -- It's All Worth It, Right? -- Chapter 2 What's in a Game? -- Game Architecture -- Applying the Game Architecture -- Application Layer -- Reading Input -- File Systems and Resource Caching -- Managing Memory -- Initialization, the Main Loop, and Shutdown -- Other Application Layer Code -- Game Logic -- Game State and Data Structures -- Physics and Collision -- Events -- Process Manager -- Command Interpreter -- Game View for the Human Player -- Graphics Display -- Audio -- User Interface Presentation -- Process Manager -- Options -- Multiplayer Games -- Game Views for AI Agents -- Networked Game Architecture -- Remote Game View -- Remote Game Logic -- Do I Have to Use DirectX? -- Design Philosophy of DirectX -- Direct3D or OpenGL -- DirectSound or What? -- DirectInput or Roll Your Own -- Other Bits and Pieces -- Further Reading -- Chapter 3 Coding Tidbits and Style That Saved Me -- General Coding Styles -- Bracing -- Consistency -- Smart Code Design Practices -- Avoiding Hidden Code and Nontrivial Operations -- Class Hierarchies: Keep Them Flat -- Inheritance Versus Composition -- Virtual Functions Gone Bad -- Use Interface Classes -- Consider Using Factories -- Encapsulate Components That Change -- Use Streams to Initialize Objects -- Smart Pointers and Naked Pointers -- Reference Counting -- C++'s shared_ptr -- Using Memory Correctly.
Understanding the Different Kinds of Memory -- Optimizing Memory Access -- Memory Alignment -- Virtual Memory -- Writing Your Own Memory Manager -- Grab Bag of Useful Stuff -- An Excellent Random Number Generator -- Pseudo-Random Traversal of a Set -- Memory Pools -- Developing the Style That's Right for You -- Further Reading -- Chapter 4 Building Your Game -- A Little Motivation -- Creating a Project -- Build Configurations -- Create a Bullet-Proof Directory Structure -- Where to Put Your Game Engine and Tools -- Setting Visual Studio Build Options -- Multiplatform Projects -- Source Code Repositories and Version Control -- A Little History-Visual SourceSafe from Microsoft -- Subversion and TortoiseSVN -- Perforce by Perforce Software -- AlienBrain from Avid -- Using Source Control Branches -- Building the Game: A Black Art? -- Automate Your Builds -- The Build Machine -- Automated Build Scripts -- Creating Build Scripts -- Normal Build -- Milestone Build -- Multiple Projects and Shared Code -- Some Parting Advice -- Chapter 5 Game Initialization and Shutdown -- Initialization 101 -- Some C++ Initialization Pitfalls -- The Game's Application Layer -- WinMain: The Windows Entry Point -- The Application Layer: GameCodeApp -- InitInstance(): Checking System Resources -- Checking for Multiple Instances of Your Game -- Checking Hard Drive Space -- Checking Memory -- Calculating CPU Speed -- Do You Have a Dirtbag on Your Hands? -- Initialize Your Resource Cache -- Loading Text Strings -- Your Script Manager and the Events System -- Initialize DirectX and Create Your Window -- Create Your Game Logic and Game View -- Set Your Save Game Directory -- Preload Selected Resources from the Cache -- Stick the Landing: A Nice Clean Exit -- How Do I Get Out of Here? -- Forcing Modal Dialog Boxes to Close -- Shutting Down the Game -- What About Consoles?.
Getting In and Getting Out -- Chapter 6 Game Actors and Component Architecture -- A First Attempt at Building Game Actors -- Component Architecture -- Creating Actors and Components -- Defining Actors and Components -- Storing and Accessing Actors -- Putting It All Together -- Data Sharing -- Direct Access -- Events -- The Best of Both Worlds -- Chapter 7 Controlling the Main Loop -- Organizing the Main Loop -- Hard-Coded Updates -- Multithreaded Main Loops -- A Hybrid Technique -- A Simple Cooperative Multitasker -- Very Simple Process Example: DelayProcess -- More Uses of Process Derivatives -- Playing Nicely with the OS -- Using the DirectX 11 Framework -- Rendering and Presenting the Display -- Your Callback Functions for Updating and Rendering -- Can I Make a Game Yet? -- Chapter 8 Loading and Caching Game Data -- Game Resources: Formats and Storage Requirements -- 3D Object Meshes and Environments -- Animation Data -- Map/Level Data -- Texture Data -- Bitmap Color Depth -- Sound and Music Data -- Video and Prerendered Cinematics -- Resource Files -- Packaging Resources into a Single File -- Other Benefits of Packaging Resources -- Data Compression and Performance -- Zlib: Open Source Compression -- The Resource Cache -- IResourceFile Interface -- ResHandle: Tracking Loaded Resources -- IResourceLoader Interface and the DefaultResourceLoader -- ResCache: A Simple Resource Cache -- Caching Resources into DirectX et al. -- World Design and Cache Prediction -- I'm Out of Cache -- Chapter 9 Programming Input Devices -- Getting the Device State -- Using XInput or DirectInput -- A Few Safety Tips -- Working with Two-Axis Controls -- Capturing the Mouse on Desktops -- Making a Mouse Drag Work -- Working with a Game Controller -- Dead Zones -- Normalizing Input -- One Stick, Two Stick, Red Stick, Blue Stick -- Ramping Control Values.
Working with the Keyboard -- Mike's Keyboard Snooper -- GetAsyncKeyState( ) and Other Evils -- Handling the Alt Key Under Windows -- What, No Dance Pad? -- Chapter 10 User Interface Programming -- DirectX's Text Helper and Dialog Resource Manager -- The Human's Game View -- A WASD Movement Controller -- Screen Elements -- A Custom MessageBox Dialog -- Modal Dialog Boxes -- Controls -- Control Identification -- Hit Testing and Focus Order -- Control State -- More Control Properties -- Hot Keys -- Tooltips -- Context-Sensitive Help -- Dragging -- Sounds and Animation -- Some Final User Interface Tips -- Chapter 11 Game Event Management -- Game Events -- Events and Event Data -- The Event Listener Delegates -- The Event Manager -- Example: Bringing It All Together -- What Game Events Are Important? -- Distinguishing Events from Processes -- Further Reading -- Chapter 12 Scripting with Lua -- A Brief History of Game Programming Languages -- Assembly Language -- C/C++ -- Scripting Languages -- Using a Scripting Language -- Rapid Prototyping -- Design Focused -- Speed and Memory Costs -- Where's the Line? -- Scripting Language Integration Strategies -- Writing Your Own -- Using an Existing Language -- Choosing a Scripting Language -- Python -- Lua -- A Crash Course in Lua -- Comments -- Variables -- Functions -- Tables -- Flow Control -- Operators -- What's Next? -- Object-Oriented Programming in Lua -- Metatables -- Creating a Simple Class Abstraction -- Memory Management -- Binding Lua to C++ -- The Lua C API -- tolua++ -- luabind -- LuaPlus -- A Crash Course in LuaPlus -- LuaState -- LuaObject -- Tables -- Globals -- Functions -- Calling C++ Functions from Lua -- Bringing It All Together -- Managing the Lua State -- Script Exports -- Process System -- Event System -- Script Component -- Lua Development and Debugging -- Final Thoughts -- Further Reading.
Chapter 13 Game Audio -- How Sound Works -- Digital Recording and Reproduction -- Sound Files -- A Quick Word About Threads and Synchronization -- Game Sound System Architecture -- Sound Resources and Handles -- IAudioBuffer Interface and AudioBuffer Class -- IAudio Interface and Audio Class -- DirectSound Implementations -- Sound Processes -- Launching Sound Effects -- Other Technical Hurdles -- Sounds and Game Objects -- Timing and Synchronization -- Mixing Issues -- Some Random Notes -- Data-Driven Sound Settings -- Background Ambient Sounds and Music -- Speech -- The Last Dance -- Chapter 14 3D Graphics Basics -- 3D Graphics Pipeline -- 3D Math 101 -- Coordinates and Coordinate Systems -- Vector Mathematics -- C++ Math Classes -- Vector Classes -- Matrix Mathematics -- Quaternion Mathematics -- Transformations -- Geometry -- Lighting, Normals, and Color -- Materials -- Textured Vertices -- Texturing -- Subsampling -- Mip-Mapping -- Introducing ID3D11Device and ID3D11DeviceContext -- Loading Textures in D3D11 -- Triangle Meshes -- Still with Me? -- Chapter 15 3D Vertex and Pixel Shaders -- The Vertex Shader and Shader Syntax -- Compiling the Vertex Shader -- C++ Helper Class for the Vertex Shader -- The Pixel Shader -- C++ Helper Class for the Pixel Shader -- Rendering with the Shader Helper Classes -- Shaders-It's Just the Beginning -- Further Reading -- Chapter 16 3D Scenes -- Scene Graph Basics -- ISceneNode Interface Class -- SceneNodeProperties and RenderPass -- SceneNode-It All Starts Here -- The Scene Class -- Special Scene Graph Nodes -- Implementing Separate Render Passes -- A Simple Camera -- Putting Lights in Your Scene -- Rendering the Sky -- Using Meshes in Your Scene -- What's Missing? -- Still Hungry? -- Further Reading -- Chapter 17 Collision and Simple Physics -- Mathematics for Physics Refresher.
Meters, Feet, Cubits, or Kellicams?.
Local Note:
Electronic reproduction. Ann Arbor, Michigan : ProQuest Ebook Central, 2017. Available via World Wide Web. Access may be limited to ProQuest Ebook Central affiliated libraries.
Genre:
Electronic Access:
Click to View