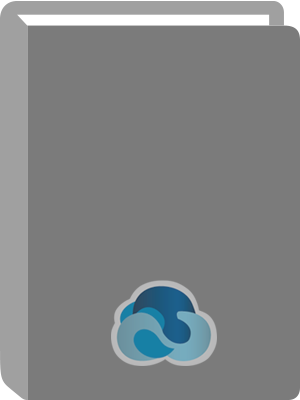
Professional Android Sensor Programming.
Title:
Professional Android Sensor Programming.
Author:
Milette, Greg.
ISBN:
9781118227459
Personal Author:
Edition:
1st ed.
Physical Description:
1 online resource (556 pages)
Contents:
Professional Android™ Sensor Programming -- Contents -- Introduction -- Part I: Location Services -- Chapter 1: Introducing the Android Location Service -- Methods Used to Determine Location -- GPS Provider -- How It Works -- GPS Improvements -- Limitations -- Controlling GPS -- Network Provider -- Using Wireless Network Access Points -- Using Cell IDs -- Summary -- Chapter 2: Determining a Device's Current Location -- Know Your Tools -- LocationManager -- LocationProvider -- Location -- Criteria -- LocationListener -- Setting up the Android Manifest -- Determining the Appropriate Location Provider -- GPS Location Provider -- Network Location Provider -- Passive Location Provider -- Accuracy versus Battery Life -- Receiving Location Updates -- Receiving Location Updates with a LocationListener -- Receiving Location Updates with a Broadcast Intent -- Implementing the Example App -- Implementing LocationListener -- onLocationChanged() -- onProviderDisabled() and onProviderEnabled() -- onStatusChanged() -- Obtaining a Handle to LocationManager -- Requesting Location Updates -- Cleaning up After Yourself -- Launching the Location Settings Activity -- Summary -- Chapter 3: Tracking Device Movement -- Collecting Location Data -- Receiving Location Updates with a Broadcast Receiver -- Extending BroadcastReceiver -- Registering the BroadcastReceiver with Android -- Requesting Location Updates with a PendingIntent -- One Intent, Multiple Receivers -- Why Not Use a Service? -- Viewing the Tracking Data -- Google Map Library Components -- MapView -- OverlayItem -- ItemizedOverlay -- MapActivity -- Filtering Location Data -- Continuous Location Tracking and Battery Life -- Reducing Location Update Frequency -- Limiting Location Providers -- Summary -- Chapter 4: Proximity Alerts -- App Structure -- Geocoding.
android.location.Geocoder -- Reading the Geocoded Response -- Setting a Proximity Alert -- Responding to a Proximity Alert -- Proximity Alert Limitations -- Battery Life -- Permissions -- More Efficient Proximity Alert -- ProximityAlertService -- Summary -- Part II: Inferring Information from Physical Sensors -- Chapter 5: Overview of Physical Sensors -- Definitions -- Android Sensor API -- SensorManager -- Sensor -- Sensor Rates -- Sensor Range and Resolution -- SensorEventListener -- SensorEvent -- Sensor List -- The Manifest File -- SensorListActivity -- SensorSelectorFragment -- SensorDisplayFragment -- Sensing the Environment -- Sensor.TYPE_LIGHT -- Sensor.TYPE_PROXIMITY -- Sensor.TYPE_PRESSURE -- Absolute Altitude -- Relative Altitude -- Mean Sea-Level Pressure (MSLP) -- Where to Find MSLP -- Sensor Units -- Sensor Range -- Common Use Cases -- Sensor.TYPE_RELATIVE_HUMIDITY -- Sensor.TYPE_AMBIENT_TEMPERATURE -- Sensor.TYPE_TEMPERATURE -- Sensing Device Orientation and Movement -- Coordinate Systems -- Global Coordinate System -- Device Coordinate System -- Angles -- Sensor.TYPE_ACCELEROMETER, .TYPE_GRAVITY, and .TYPE_LINEAR_ACCELERATION -- Sensor Units and Resolution -- Sensor.TYPE_GYROSCOPE -- Sensor Units -- Sensor Range -- Sensor.TYPE_MAGNETIC_FIELD -- Sensor Units, Range, and Resolution -- Sensor.TYPE_ROTATION_VECTOR -- SensorManager.getOrientation() -- SensorManager.getInclination() -- Sensor Fusion Schemes -- Summary -- Chapter 6: Errors and Sensor Signal Processing -- Definitions -- Accuracy and Precision -- Types of Errors -- Human Error, Systematic Error, and Random Error -- Noise -- Drift -- Zero Offset (or "Offset," or "Bias") -- Time Delays and Dropped Data -- Integration Error -- Techniques to Address Error -- Re-zeroing -- Filters -- Sensor Fusion -- Filters -- Low-Pass -- Weighted Smoothing.
Simple Moving Average (SMA) -- Choosing the Smoothing Parameter -- Averaging: Smoothness vs. Response Time -- Simple Moving Median (SMM) -- High-Pass -- Inverse Low-Pass Filter -- Bandpass -- Introducing Kalman Filters -- A Better Determination of Orientation by Using Sensor Fusion -- Sensor Fusion: Simple vs. Proprietary -- Proprietary Sensor Fusion -- Simple Sensor Fusion: The Balance Filter -- Summary -- Chapter 7: Determining Device Orientation -- Previewing the Example App -- Determining Device Orientation -- Gravity Sensor -- Accelerometer and Magnetometer -- Gravity Sensor and Magnetometer -- Rotation Vector -- Implementation Details -- Processing Gravity Sensor Data -- Processing Accelerometer and Magnetic Field Data -- Processing Rotation Vector Data -- Notifying the User of Orientation Changes -- NorthFinder -- Summary -- Chapter 8: Detecting Movement -- Acceleration Data -- Accelerometer Data -- Linear Acceleration Sensor Data -- Data While Device Is in Motion -- Total Acceleration -- Implementation -- DetermineMovementActivity -- AccelerationEventListener -- Summary -- Chapter 9: Sensing the Environment -- Barometer vs. GPS for Altitude Data -- Example App Overview -- Implementation Details -- GPS-Based Altitude -- Barometric Pressure-Based Altitude -- Relative Altitude -- Summary -- Chapter 10: Android Open Accessory -- A Short History of AOA -- USB Host Versus USB Accessory -- Electrical Power Requirements -- Supported Android Devices -- The Android Development Kit (ADK) -- Hardware Components -- Software Components -- AOA Sensors versus Native Device Sensors -- AOA Beyond Sensors -- AOA Limitations -- AOA and Sensing Temperature -- Implementation -- Requirements -- Getting Started with the Arduino Software -- Arduino Sketch -- Android Code -- Communication between Arduino and Android.
Taking an Android Accessory to the Consumer Market -- Summary -- Part III: Sensing the Augmented, Pattern-Rich External World -- Chapter 11: Near Field Communication (NFC) -- What Is RFID? -- What Is NFC? -- The NDEF Data Format -- How and Where to Buy NFC Tags -- NDEF-compatible NFC Tags -- Storage Size versus Price versus Security Trade-off -- Write Protection -- Form Factor -- Retailers -- General Advantages and Disadvantages of NFC -- Low Power and Proximity Based -- Small, Short Data Bursts -- Singular Scanning -- Security -- Card Emulation -- Android-specific Advantage: Intents -- Required Hardware -- Building an Inventory Tracking System -- The Scenario -- The NFC Inventory Demonstration App -- Enabling NFC in the Settings -- Debugging Your Tags with Apps -- Android APIs -- In Your AndroidManifest.xml File -- Permissions and Minimum API Level -- Intent Filters -- Custom MIME Type Intent Filters -- URI-based Intent Filters -- In Your Main Activity Class -- NfcManager -- NfcAdapter -- Foreground Dispatching -- Foreground NDEF Push -- Reacting to an NDEF Tag -- NdefMessage and NdefRecord -- Parsing and Reading NDEF Tags -- Getting Ready to Write to a Tag -- Writing to the Tag -- Putting it All Together -- Future Considerations -- NFC N-Mark -- Peer-to-Peer NFC Sharing -- Peer-to-Peer Android APIs -- Go Forth and NFC! -- Summary -- Chapter 12: Using the Camera -- Using the Camera Activity -- Controlling the Camera with Your Own Activity -- Claiming and Releasing a Camera -- The Preview View -- Controlling the Camera -- Orientation -- Zoom -- Focus -- Switching Cameras -- Flash -- Other Camera Parameters -- Creating a Simple Barcode Reader -- Understanding Barcodes -- Parity and Implied First Digit -- The Check Digit -- Right Half of the Barcode -- Autofocus.
Using the Camera Preview Image and Detecting the Barcode -- Debugging Image Processing Programs on Android -- Detecting the Barcode -- Summary -- Chapter 13: Image-Processing Techniques -- The Structure of Image-Processing Programs -- The Image-Processing Pipeline -- Common Image-Processing Operations -- Image-to-Image Operations -- Image-to-Object Operations -- Jon's Java Imaging Library (JJIL) -- Image -- PipelineStage -- Sequence -- Ladder -- JJIL and Detecting the Android Logo -- Choose the Right Image Size -- Improving Reliability in Image Processing -- Detecting Faces -- Image-Processing Resources -- Summary -- Chapter 14: Using the Microphone -- Introducing the Android Clapper -- Using MediaRecorder to Analyze Maximum Amplitude -- Recording Maximum Amplitude -- Asynchronous Audio Recording -- Implementing a Clapper -- Analyzing Raw Audio -- Setting Audio Input Parameters -- Preparing AudioRecord -- Recording Audio -- Using OnRecordPositionUpdateListener -- Using Loud Noise Detection -- Using Consistent Frequency Detection -- Estimating Frequency -- Implementing the Singing Clapper -- Summary -- Part IV: Speaking to Android -- Chapter 15: Designing a Speech-enabled App -- Know Your Tools -- User Interface Screen Flow -- Voice Action Types -- Voice User Interface (VUI) Design -- Deciding Appropriate Tasks for Voice Actions -- Designing What the App and Users Will Say -- Constrain Speech Input to Increase Accuracy -- Train Users to Know What They Can Say -- Prompt the Users so They Know What to Say -- Confirm Success and Help Users Recover from Errors -- Help Users Recover from Accidental Speech Activation -- Teach Users Proper Speech Hygiene -- Use Menus Cautiously -- After the Design -- Testing Your Design -- Summary -- References.
Chapter 16: Using Speech Recognition and Text-to-Speech APIs.
Abstract:
Learn to build human-interactive Android apps, starting with device sensors This book shows Android developers how to exploit the rich set of device sensors-locational, physical (temperature, pressure, light, acceleration, etc.), cameras, microphones, and speech recognition-in order to build fully human-interactive Android applications. Whether providing hands-free directions or checking your blood pressure, Professional Android Sensor Programming shows how to turn possibility into reality. The authors provide techniques that bridge the gap between accessing sensors and putting them to meaningful use in real-world situations. They not only show you how to use the sensor related APIs effectively, they also describe how to use supporting Android OS components to build complete systems. Along the way, they provide solutions to problems that commonly occur when using Android's sensors, with tested, real-world examples. Ultimately, this invaluable resource provides in-depth, runnable code examples that you can then adapt for your own applications. Shows experienced Android developers how to exploit the rich set of Android smartphone sensors to build human-interactive Android apps Explores Android locational and physical sensors (including temperature, pressure, light, acceleration, etc.), as well as cameras, microphones, and speech recognition Helps programmers use the Android sensor APIs, use Android OS components to build complete systems, and solve common problems Includes detailed, functional code that you can adapt and use for your own applications Shows you how to successfully implement real-world solutions using each class of sensors for determining location, interpreting physical sensors, handling images and audio, and recognizing and acting on speech Learn how to write programs for this fascinating aspect of mobile app development with
Professional Android Sensor Programming.
Local Note:
Electronic reproduction. Ann Arbor, Michigan : ProQuest Ebook Central, 2017. Available via World Wide Web. Access may be limited to ProQuest Ebook Central affiliated libraries.
Genre:
Added Author:
Electronic Access:
Click to View