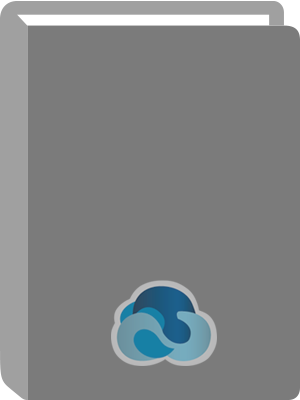
Game Programming All in One.
Title:
Game Programming All in One.
Author:
Sousa, Bruno Miguel Teixeira de.
Personal Author:
Physical Description:
1 online resource (992 pages)
Contents:
CONTENTS -- LETTER FROM THE SERIES EDITOR -- INTRODUCTION -- Part One: C++ Programming -- CHAPTER 1 INTRODUCTION TO C++ PROGRAMMING -- Why Use C++? -- Setting Up Visual C++ -- Your First Program: "Hello all you happy people" -- Structure of a C++ Program -- Commenting -- Catching Errors -- Warnings -- Summary -- Questions and Answers -- Exercises -- CHAPTER 2 VARIABLES AND OPERATORS -- Variables and Memory -- What Type of Variables Are There? -- Using Variables in Your Programs -- Variable Modifiers -- Variable Naming -- Redefining Types -- What Is an Operator? -- Bitwise Shift Operators -- Relational Operators -- Conditional Operator -- Logical Operators -- Operator Precedence -- Summary -- Questions and Answers -- Exercises -- CHAPTER 3 FUNCTIONS AND PROGRAM FLOW -- Functions: What Are They and What Are They Used For? -- Creating and Using Functions -- Default Parameters -- Variable Scope -- Recursion -- Things to Remember When Using Functions -- Program Flow -- Code Blocks and Statements -- if, else if, else Statements -- while, do ... while, and for Loops -- Breaking and Continuing -- Switching to switch -- Randomizing -- First Game: "Craps" -- Summary -- Questions and Answers -- Exercises -- CHAPTER 4 MULTIPLE FILES AND THE PREPROCESSOR -- Differences between Source and Header Files -- Handling Multiple Files -- What Is the Preprocessor? -- Avoiding Multiple Includes -- Macros -- Other Preprocessor Directives -- Summary -- Exercises -- CHAPTER 5 ARRAYS, POINTERS, AND STRINGS -- What Is an Array? -- Declaring and Using an Array -- Multi-Dimensional Arrays -- Pointers to What? -- Pointers and Variables -- Pointers and Arrays -- Declaring and Allocating Memory to a Pointer -- Pointer Operators -- Manipulating Memory -- Strings -- Summary -- Questions and Answers -- Exercises -- CHAPTER 6 CLASSES -- What Is a Class? -- Building Classes.
Using Classes -- Private, Protected, and Public Members -- Constructors and the Destructor -- Operator Overloading -- Putting It All Together-The String Class -- Basics of Inheritance and Polymorphism -- Enumerations -- Unions -- Static Members -- Useful Techniques Using Classes -- Summary -- Questions and Answers -- Exercises -- CHAPTER 7 DEVELOPING MONSTER -- ConLib -- Building Monster -- Summary -- CHAPTER 8 STREAMS -- What Is a Stream? -- Binary and Text Streams -- Input and Output -- File Streams -- Modifying Monster to Save and Load Games -- Summary -- Questions and Answers -- Exercises -- CHAPTER 9 BASIC SOFTWARE ARCHITECTURE -- The Importance of Software Design -- Design Approaches -- Some Basic Techniques -- Modules and Multiple Files -- Naming Conventions -- Where Common Sense Beats Design -- The Design Used in This Book -- Summary -- Questions and Answers -- Exercises -- Part Two: Windows Programming -- CHAPTER 10 DESIGNING YOUR GAME LIBRARY: MIRUS -- General Description -- Mirus Components -- Helper Component -- Window Component -- Graphics Component -- Sound Component -- Input Component -- Building the Help Component -- How to Use Mirus -- Summary -- Questions and Answers -- CHAPTER 11 BEGINNING WINDOWS PROGRAMMING -- History of Windows -- Introduction to Windows Programming -- Visual C++ and Windows Applications -- Building the Windows Application -- Creating a Real-Time Message Loop -- Making a Reusable Window Class -- Using the Mirus Window Framework -- Some Common Window Functions -- Show -- Summary -- Questions and Answers -- Exercises -- CHAPTER 12 INTRODUCTION TO DIRECTX -- What Is DirectX? -- Brief History of DirectX -- Why Use DirectX? -- DirectX Components -- How Does DirectX Work? -- How to Use DirectX with Visual C++ -- Summary -- Questions and Answers -- Exercises -- CHAPTER 13 DIRECTX GRAPHICS.
Interfaces You Will Be Using -- Using Direct3D: The Basics -- Surfaces, Buffers, and Swap Chains -- Rendering Surfaces -- Vertices, Polygons, and Textures -- Windows Bitmaps -- Full Screen and Other Bit Modes -- Color Theory and Color Keying -- Targa Files -- Animation and Template Sets -- Collision Detection -- 2D Image Manipulation -- 2D Primitives Revealed -- Developing Mirus -- Summary -- Questions and Answers -- Exercises -- CHAPTER 14 DIRECTINPUT -- Introduction to DirectInput -- mrInputManager -- mrKeyboard -- mrMouse -- mrJoystick -- Summary -- Questions and Answers -- Exercises -- CHAPTER 15 DIRECTSOUND -- Sound Theory -- DirectSound Basics -- mrSoundPlayer -- mrSound -- Media Control Interface -- mrCDPlayer -- Summary -- Questions and Answers -- Exercises -- Part Three: Hardcore Game Programming -- CHAPTER 16 INTRODUCTION TO GAME DESIGN -- What Is Game Design? -- The Dreadful Design Document -- Why the "It's in My Head" Technique Isn't Good -- The Two Types of Designs -- A Fill In Design Document Template -- A Sample Game Design: Space Invaders -- Summary -- Questions and Answers -- Exercises -- CHAPTER 17 DATA STRUCTURES AND ALGORITHMS -- The Importance of the Correct Data Structures and Algorithms -- Lists -- Trees -- General Trees -- Binary Search Trees -- Sorting Data -- Bubble Sort -- The Quick Sort -- Compression -- RLE Compression -- Summary -- Questions and Answers -- Exercises -- CHAPTER 18 THE MATHEMATICAL SIDE OF GAMES -- Trigonometry -- Vectors -- Matrices -- Probability -- Functions -- Summary -- Questions and Answers -- Exercises -- CHAPTER 19 INTRODUCTION TO ARTIFICIAL INTELLIGENCE -- The Various Fields of Artificial Intelligence -- Deterministic Algorithms -- Finite State Machines -- Fuzzy Logic -- A Simple Method for Memory -- Artificial Intelligence and Games -- Summary -- Questions and Answers -- Exercises.
CHAPTER 20 INTRODUCTION TO PHYSICS MODELING -- Introduction to Physics -- Building a Physics Engine -- Basic Physics Concepts -- Forces -- Gravitational Interaction -- Friction -- Handling Collisions -- Simulating -- Particle Systems -- Summary -- Questions and Answers -- Exercises -- CHAPTER 21 BUILDING BREAKING THROUGH -- Designing Breaking Through -- Building Breaking Through -- Conclusion -- CHAPTER 22 PUBLISHING YOUR GAME -- Is Your Game Worth Publishing? -- Whose Door to Knock On -- Learn to Knock Correctly -- Contracts -- Milestones -- No Publisher, Now What? -- Interviews -- Summary -- References -- Conclusion -- Part Four: Appendixes -- APPENDIX A: WHAT'S ON THE CD-ROM -- Source -- Microsoft DirectX 8.0 SDK -- Programs -- Games -- APPENDIX B: DEBUGGING USING MICROSOFT VISUAL C++ -- Breakpoints and Controlling Execution -- Modifying Variables During Runtime -- Watching Variables -- APPENDIX C: BINARY, HEXADECIMAL, AND DECIMAL SYSTEM -- Binary -- Hexadecimal -- Decimal -- APPENDIX D: A C PRIMER -- Standard Input and Output -- File Input and Output -- Structures: Say Bye-Bye to Classes -- Dynamic Memory -- APPENDIX E: ANSWERS TO THE EXERCISES -- Chapter 1 -- Chapter 2 -- Chapter 3 -- Chapter 4 -- Chapter 5 -- Chapter 6 -- Chapter 7 -- Chapter 8 -- Chapter 9 -- Chapter 10 -- Chapter 11 -- Chapter 12 -- Chapter 13 -- Chapter 14 -- Chapter 15 -- Chapter 16 -- Chapter 17 -- Chapter 18 -- Chapter 19 -- Chapter 20 -- APPENDIX F: C++ KEYWORDS -- APPENDIX G: USEFUL TABLES -- ASCII Table -- Integral Table -- Derivatives Table -- Inertia Equations Table -- APPENDIX H: MORE RESOURCES -- Game Development and Programming -- News, Reviews, and Download Sites -- Engines -- Independent Game Developers -- Industry -- Computer Humor -- Books -- INDEX -- A -- B -- C -- D -- E -- F -- G -- H -- I -- J -- K -- L -- M -- N -- O -- P -- Q -- R -- S -- T -- U -- V.
W -- X -- Z.
Local Note:
Electronic reproduction. Ann Arbor, Michigan : ProQuest Ebook Central, 2017. Available via World Wide Web. Access may be limited to ProQuest Ebook Central affiliated libraries.
Subject Term:
Genre:
Electronic Access:
Click to View