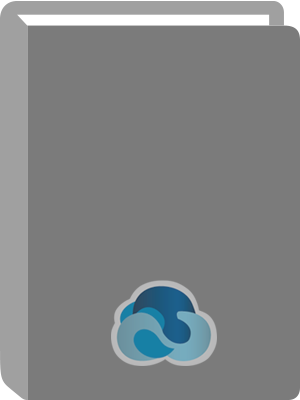
Game Engine Toolset Development.
Title:
Game Engine Toolset Development.
Author:
Staff, Course Technology Ptr.
Personal Author:
Physical Description:
1 online resource (710 pages)
Contents:
Contents -- Introduction -- PART I: TOOLSET DESIGN FUNDAMENTALS -- Chapter 1 What Is a Tool? What Is a Toolset? -- Stakeholders: Internal Versus External -- Who Builds the Tools? -- How Large Are Tools Teams? -- Chapter 2 Why Use C#? Why Use .NET? -- Overview of .NET -- Overview of C# -- Legacy Interoperability -- Benefits -- Chapter 3 Examples of Commercial Toolsets -- Case Study: BioWare Corporation -- Case Study: Artificial Studios -- Chapter 4 Everything Starts with a Plan -- Vision -- Stakeholders -- Reusability -- Architecture -- Requirements -- Design Standards -- Coding Standards -- Documentation -- Testing -- Defect Tracking -- Life Cycle -- Development Environment -- Staging Environment -- Production Environment -- Chapter 5 Development Phases of a Tool -- Phase: Planning -- Phase: Analysis -- Phase: Design -- Phase: Implementation -- Chapter 6 Measurement Metrics for Tool Quality -- Metric: Maintainability -- Metric: Traceability -- Metric: Performance -- Metric: Usability -- Metric: Testability -- Metric: Portability -- Metric: Reliability -- Metric: Efficiency -- Chapter 7 Fundamentals of User Interface Design -- Principle of Consistency -- Principle of Transparency -- Principle of Feedback -- Principle of Refinement -- Principle of Exploration -- Principle of Modality -- Principle of Self-Evidence -- Principle of Moderation -- Principle of Customization -- Chapter 8 Distributed Componential Architecture Design -- Architecture Overview -- Core Components -- Specific Tool Logic -- Console Entry Point -- Windows Entry Point -- Other Entry Points -- Architecture Example -- Alternate Architecture Structure -- Chapter 9 Solutions to Bridge Domain Gaps -- Compositional Friction -- Cause: Domain Coverage -- Cause: Design Intentions -- Cause: Framework Gap -- Cause: Entity Overlap -- Cause: Legacy Components -- Cause: Source Code Access.
Relevant Design Patterns -- Chapter 10 Unit Testing with NUnit -- Overview of Unit Testing -- Introducing NUnit -- Creating an NUnit Project -- Attribute Overview -- Expected Outcome Assertion -- A Simple Example -- Running Tests -- Debugging with Visual Studio -- Chapter 11 Code Documentation with NDoc and XML -- Configuring the Project -- Supported XML Markup -- Commenting Example -- Generating the Documentation -- Chapter 12 Microsoft Coding Conventions -- Styles of Capitalization -- Naming Classes -- Naming Interfaces -- Naming Namespaces -- Naming Attributes -- Naming Enumerations -- Naming Static Fields -- Naming Parameters -- Naming Methods -- Naming Properties -- Naming Events -- Abbreviations -- Chapter 13 Enforcing Coding Policies with FxCop -- Installing FxCop -- Creating an FxCop Project -- Configuring Built-In Rules -- Analyzing Your Project -- Building Custom Rules -- Chapter 14 Best Practices for Robust Exception Handling -- External Data Is Evil -- Creating Custom Exceptions -- Throwing Exceptions -- Structured Exception Handlers -- Logging Exception Information -- Mechanisms for Cleanup -- Unhandled and Thread Exception Events -- PART II: TECHNIQUES FOR ARBITRARY TOOLS -- Chapter 15 Compressing Data to Reduce Memory Footprint -- Types of Compression -- GZipStream Compression in .NET 2.0 -- Implementation for Arbitrary Data -- Implementation for Serializable Objects -- Chapter 16 Protecting Sensitive Data with Encryption -- Encryption Rudiments -- Selecting a Cipher -- ICryptoTransform Interface -- Chapter 17 Generic Batch File Processing Framework -- Goals -- Proposed Solution -- Implementation -- Chapter 18 Ensuring a Single Instance of an Application -- Early Solutions -- Journey to the Dark Side -- The Solution -- Chapter 19 Implementing a Checksum to Protect Data Integrity -- Implementation -- Usage -- Alternative.
Chapter 20 Using the Property Grid Control with Late Binding -- Designing a Bindable Class -- Ordering Properties -- Using the PropertyGrid -- Chapter 21 Adding Printing Support for Arbitrary Data -- Printing Regular Text -- Supporting Printer Selection -- Supporting Page Setup -- Supporting Print Preview -- Chapter 22 Flexible CommandLine Tokenizer -- Formatting Styles -- Implementation -- Sample Usage -- Chapter 23 Layering Windows Forms on Console Applications -- Implementation -- Sample Usage -- Chapter 24 Overview of Database Access with ADO.NET -- Advantages of ADO.NET -- ADO.NET Object Model -- Working with a DataReader -- Working with a DataAdapter -- Working with XML -- Potion Database Editor -- PART III: TECHNIQUES FOR GRAPHICAL TOOLS -- Chapter 25 Using Direct3D Swap Chains with MDI Applications -- What Is a Swap Chain? -- Thoughts for SDI and MDI Applicability -- Common Pitfalls -- The Proposed Solution -- Chapter 26 Constructing an Aesthetic Texture Browser Control -- Swappable Loader Interface -- Windows GDI+ Loader -- Managed Direct3D Loader -- Storing Texture Information -- Building the Thumbnail Control -- Handling Custom User Events -- Building the Viewer Control -- Using the Control -- Loading Textures from a Directory -- Loading Textures from a MemoryStream -- Loading Textures from a Bitmap -- Texture Browser Demo -- Chapter 27 Converting from Screen Space to World Space -- Transforming Screen Coordinates -- Computing the Picking Ray -- Bounding Sphere Intersection Tests -- Improving Intersection Accuracy -- Using Built-In D3DX Functionality -- Chapter 28 Asynchronous Input Device Polling -- Asynchronous Mouse Polling -- Asynchronous Keyboard Polling -- Sample Usage -- PART IV: TECHNIQUES FOR NETWORK TOOLS -- Chapter 29 Downloading Network Files Asynchronously -- HttpWebRequest and HttpWebResponse -- The Request Object.
Maintaining Data State -- The Core System -- Sample Usage -- PART V: TECHNIQUES FOR LEGACY INTEROPERABILITY -- Chapter 30 Exchanging Data Between Applications -- What Microsoft.NET Provides -- What Microsoft.NET Should Provide -- Building a Wrapper Around WM_COPYDATA -- Chapter 31 Interacting with the Clipboard -- The Clipboard Class and IDataObject -- Storing Built-In Types -- Storing Custom Data Formats -- Querying Available Data Formats -- Complete Solution -- Chapter 32 Using .NET Assemblies as COM Objects -- COM Callable Wrappers (CCW) -- Applying Interop Attributes -- Registering with COM -- Accessing from Unmanaged Code -- Deployment Considerations -- Chapter 33 Managing Items in the Recent Documents List -- Implementation -- Example Usage -- PART VI: TECHNIQUES TO IMPROVE PERFORMANCE -- Chapter 34 Playing Nice with the Garbage Collector -- Overview of the Garbage Collector -- Collecting the Garbage -- Allocation Profile -- CLR Profiler and GC Monitoring -- Finalization and the Dispose Pattern -- Weak Referencing -- Explicit Control -- Chapter 35 Using Unsafe Code and Pointers -- Rudiments of Pointer Notation -- Using an Unsafe Context -- Pinning Memory with the Fixed Statement -- Disabling Arithmetic Overflow Checking -- Allocating High Performance Memory -- Getting Size of Data Types -- Example: Array Iteration and Value Assignment -- Example: Data Block Copying -- Example: Win32 API Access -- Chapter 36 Investigating Managed Code Performance -- Investigating Performance -- Avoid Manual Optimization -- String Comparison -- String Formatting -- String Reversal -- Compiling Regular Expressions -- Use the Most Specific Type -- Avoid Boxing and Unboxing -- Use Value Types Sensibly -- The Myth About Foreach Loops -- Use Asynchronous Calls -- Efficient IO Buffer Sizes -- Minimize the Working Set -- Perform Chunky Calls.
Minimize Exception Throwing -- Thoughts About NGen -- Chapter 37 Responsive UI During Intensive Processing -- Implementing the Worker Logic -- Reporting Operation Progress -- Supporting User Cancellation -- Executing the Worker Thread -- PART VII: TECHNIQUES TO ENHANCE USABILITY -- Chapter 38 Designing an Extensible Plugin-Based Architecture -- Designing a Common Interface -- Embedding Plugin Metadata Information -- Building a Proxy Wrapper -- Loading Plugins Through the Proxy -- Reloading Plugins During Runtime -- Runtime Compilation of Plugins -- Enforcing a Security Policy -- Chapter 39 Persisting Application Settings to Isolated Storage -- Concept of Isolated Storage -- Accessing Isolated Storage -- Levels of Isolation -- Management and Debugging -- Chapter 40 Designing a Reusable and Versatile Loading Screen -- Splash Dialog -- Go for the Gusto -- Concept of Loading Jobs -- Responsive Processing -- Simple Example -- Chapter 41 Writing Context Menu Shell Extensions -- Unmanaged Interfaces -- Reusable Framework -- Sample Usage-Standalone -- Sample Usage-Integrated -- Component Registration -- Debugging Techniques -- PART VIII: TECHNIQUES TO INCREASE PRODUCTIVITY -- Chapter 42 Automating Workflow Using Job Scheduling -- Benefits -- Solution Goals -- Implementation -- Chapter 43 MVC Object Model Automation with CodeDom -- Advantages of an Automatable Object Model -- Comparison with Model-View-Controller Pattern -- A Simple Object Model Architecture -- Plugin-Based Architectures -- Controlling an Object Model with Scripts -- Implementing a C# Command Window -- Simple Automation and MVC Example -- PART IX: TECHNIQUES FOR DEPLOYMENT AND SUPPORT -- Chapter 44 Deployment and Versioning with ClickOnce -- ClickOnce and MSI Comparison -- Creating the Application -- Publishing the Application -- Launching the Application -- Deployment Configuration.
Pushing Application Updates.
Local Note:
Electronic reproduction. Ann Arbor, Michigan : ProQuest Ebook Central, 2017. Available via World Wide Web. Access may be limited to ProQuest Ebook Central affiliated libraries.
Genre:
Electronic Access:
Click to View