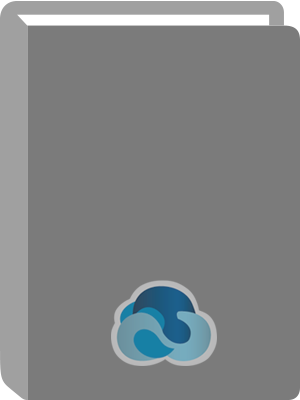
1001 Microsoft Visual C++ Programming Tips.
Title:
1001 Microsoft Visual C++ Programming Tips.
Author:
Wright, Charles.
Personal Author:
Physical Description:
1 online resource (1422 pages)
Contents:
Contents -- Programming Basics -- Understanding the Concept of Software -- Understanding How a Computer Runs a Program -- Understanding Low-Level Languages -- Understanding High-Level Languages -- Running an Interpreted Language Program -- Running a Compiled Language Program -- Where Does C++ Fit In -- Understanding Program Files in Visual -- Creating Source Code Files -- Understanding and Creating Header Files -- Structure and Syntax -- Understanding Computer Languages -- Understanding Syntax in a Computer Language -- Understanding Tokens -- How C and C++ Use White Space -- Understanding Variables -- Naming Variables -- Understanding Expressions -- Statements in C/C++ -- Understanding Program Flow -- Breaking the Program Flow: understanding the goto statement -- Breaking the Program Flow: Calling Functions -- Understanding Program Structure -- Understanding Functions in C/C++ -- Writing Reusable Code in Functions -- Using Library Functions -- The C Programming Language -- Understanding the Data Type -- Data Types in the C/C++ Language -- Defining Your Own Data Types -- Understanding Operators -- Assignment Operators -- Understanding Unary Operators -- Understanding Arithmetic Operators -- Other Operators -- Understanding How the Compiler Evaluates Expressions -- Using Parentheses to Group Operators -- Understanding C/C++ Keywords -- Compiler Directives -- Introduction to Structures and Unions -- Introducing the C++ Class -- How C and C++ Handle Structures and Unions -- C and C++ Concepts -- Understanding Naming Conventions -- The Hungarian Notation System -- Understanding the Difference Between a Declaration and a Definition -- Understanding Declarations: Declaring Variables -- Understanding Declarations: Declaring Variables in a Function Call -- Understanding Declarations: Prototyping Functions.
Understanding the #define Compiler Directive: Defining Constants -- Understanding the #define Compiler Directive: Defining Macros -- Understanding Variable Storage Classes: The Automatic Variable -- Understanding Variable Storage Classes: The Register Variable -- Understanding Variable Storage Classes: The Static Variable -- Understanding Variable Storage Classes: The Static Variable in a Class -- Introduction to Recursion -- Understanding Recursion: A Sorting Problem -- Understanding Recursion: A Math Problem -- Understanding Pointers -- Understanding Pointers: Indirection -- Understanding Arrays -- Understanding Strings as an Array of Type char -- Commenting Your Code -- Files-Basic Types -- File Types Used by the Visual C++ IDE -- Understanding Text Files and Document Files -- Creating and Using Source Files -- Creating and Using Header Files -- Using a Guardian to Protect Against Including a Header File More Than Once -- Understanding Makefiles -- Understanding Makefiles: Defining Environment Variables -- Understanding Makefiles: Setting Dependencies -- Using NMAKE.EXE -- Understanding Executable Files -- Understanding Characters -- Understanding Characters: The ASCII Character Set -- Understanding Characters: The Trans-ASCII or Extended Character Set -- C/C++ Escape Sequences: Embedding Unprintable Characters -- C/C++ Escape Sequences: Using the Backslash -- C/C++ Escape Sequences: Using the Percent Character to Format text -- Using printf: Formatting Output -- Using printf: Setting the Field Width -- Using printf: Setting the Precision -- Using printf: Zero and Space Filling the Field -- C++ I/O Streams: cout, cin, cerr -- Exploring the Visual C++ Environment -- Creating a Project -- Visual Studio Menus -- Visual Studio Menus: The File Menu -- Visual Studio Menus: The Edit Menu -- Visual Studio Menus: The View Menu.
Visual Studio Menus: The Insert Menu -- Visual Studio Menus: The Project Menu -- Visual Studio Menus: The Build and Tools Menus -- Customizing Visual Studio Menus -- Adding Commands to the Tools Menu -- The Visual Studio Pop-Up (Context) Menus -- Exploring Visual Studio Windows: The Editing Window -- Exploring Visual Studio Windows: The Output Window -- Exploring Visual Studio Windows: The Variables Window -- Exploring Visual Studio Windows: The Watch Window -- Exploring Visual Studio Windows: Hiding, Showing, and Docking Windows -- Exploring the Workspace Window: The ClassView Panel -- Exploring the Workspace Window: The ResourceView Panel -- Exploring the Workspace Window: The FileView Panel -- Exploring Visual Studio Toolbars -- Exploring Visual Studio Toolbars: What They Do -- Exploring Visual Studio Toolbars: Hiding and Showing Toolbars -- Exploring Visual Studio Toolbars: Customizing Toolbars -- Exploring Visual Studio Toolbars: Creating Your Own Toolbars -- Getting Help in Visual Studio -- Using the Find In Files Command -- Context Tracking in the Visual Studio -- Using the Full Screen Editor -- Using the Split Panel to View Two Locations in the Same File -- Using the Debug Windows -- Finishing the Program Editor -- Getting Started With Programming -- Running the Compiler From the Command Line -- Creating a Source File -- The main() Function -- Adding a Header File to the Program -- Using printf to Output Text -- "Hello, World": A Simple Command-Line C++ Program -- The Formatted Output Family -- Formatted Input -- The Three Standard Files: stdin, stdout, and stderr -- Using the C++ Streams cout, cin, and cerr -- Redirecting stdout to a File -- Arguments to main(): argc and argv -- Using an if Statement to Control Output -- Opening a File -- Using the File I/O Functions -- Adding a while Loop -- Adding a Function to the Program.
Using a for Loop -- Compiler Command-Line Options -- Adding a Second Source File -- Adding a Header File -- Creating a Makefile -- Running nmake.exe to Compile a Program -- C++ Operators -- Understanding Operator Types -- Understanding Unary Operators: The Indirection Operator (*) -- Understanding Unary Operators: The Address Operator (&) -- Understanding Unary Operators: The Increment and Decrement Operators (++ and - -) -- Understanding Unary Operators: The Negation and Complement Operators (! and ~) -- Understanding Unary Operators: The Plus and Minus Operators (+ and -) -- Understanding Arithmetic Operators: The Multiplicative Operators (*, /, and %) -- Understanding Arithmetic Operators: The Additive Operators (+ and -) -- Understanding Relational Operators: The Equality Operators (== and !=) -- Understanding Relational Operators: The Less Than and Greater Than Operators () -- Understanding Relational Operators: The Less Than or Equals and Greater Than or Equals Operators (=) -- Understanding Bitwise Operators: The Shift Operators (>) -- Understanding Bitwise Operators: The AND Operator (&) -- Understanding Bitwise Operators: The OR Operator (
Introduction to Flow Control -- Using the if Keyword -- Using the else Keyword -- Executing a Single Statement -- Executing Multiple Statements as the Object of an if operator -- Combining the if and else Keywords -- Using Multiple if Conditions -- Using the Conditional Operator -- Introduction to Scope -- Understanding Scope Within a Conditional -- Some Common Errors with Conditional Statements -- Program Loops -- Introduction to Loops -- Constructing a Loop with a Conditional Statement and a goto -- Introducing the while Loop -- Using the continue and break Statements -- Introducing the do while Loop -- Introducing the for Loop -- Understanding Scope Within a Loop -- The switch Statement -- Understanding the switch Statement -- Using case and default Statements -- Declaring Variables Within the Scope of a switch Statement -- Declaring Variables Within a case -- A Look at the C++ Language -- A Brief History of C -- Making the Transition to C++ -- Understanding Structures in C -- Introduction to the Class in C++ -- Understanding Similarities and Differences Between Classes and Structures -- Declaring Variables in C and C++ -- Understanding Function Prototyping -- Arrays in C/C++ -- Understanding Arrays -- Declaring Arrays of Variables -- Using the String Array -- Using Arrays of Objects -- Understanding Pointers to Arrays -- Accessing Array Elements -- Understanding Array Arithmetic -- Incrementing and Decrementing Array Variables -- Declaring Multidimensional Arrays -- Macros, Constants, and Preprocessor Commands: Defining and Using Constants -- Understanding Constants -- Using the #define Preprocessor Command -- Beware of the Semicolon in #define statements -- Declaring Constant Values -- Declaring Constants without Values -- Understanding String Constants -- Defining Numerical Constants -- Defining a Constant Using Variables.
Undefining a Constant.
Local Note:
Electronic reproduction. Ann Arbor, Michigan : ProQuest Ebook Central, 2017. Available via World Wide Web. Access may be limited to ProQuest Ebook Central affiliated libraries.
Genre:
Added Author:
Electronic Access:
Click to View