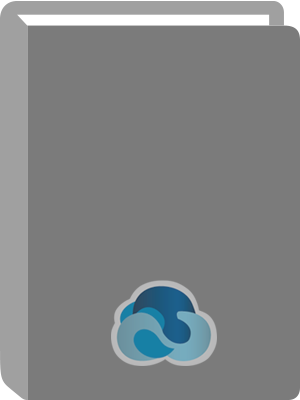
Expert Python Programming.
Title:
Expert Python Programming.
Author:
Ziade, Tarek.
ISBN:
9781847194954
Personal Author:
Edition:
1st ed.
Physical Description:
1 online resource (401 pages)
Contents:
Expert Python Programming -- Table of Contents -- Expert Python Programming -- Credits -- Foreword -- About the Author -- About the Reviewers -- Preface -- What This Book Covers -- What You Need for This Book -- Who This Book Is For -- Conventions -- Reader Feedback -- Customer Support -- Downloading the Example Code for the Book -- Errata -- Piracy -- Questions -- 1. Getting started -- Installing Python -- Python Implementations -- Jython -- IronPython -- PyPy -- Other Implementations -- Linux Installation -- Package Installation -- Compiling the Sources -- Windows Installation -- Installing Python -- Installing MinGW -- Installing MSYS -- Mac OS X Installation -- Package Installation -- Compiling the Source -- The Python Prompt -- Customizing the Interactive Prompt -- iPython: An Advanced Prompt -- Installing setuptools -- Understanding How It Works -- setuptools Installation Using EasyInstall -- Hooking MinGW into distutils -- Working Environment -- Using an Editor and Complementary Tools -- Code Editor -- Installing and Configuring Vim -- Using Another Editor -- Extra Binaries -- Using an Integrated Development Environment -- Installing Eclipse with PyDev -- Summary -- 2. Syntax Best Practices-Below the Class Level -- List Comprehensions -- Iterators and Generators -- Generators -- Coroutines -- Generator Expressions -- The itertools Module -- islice: The Window Iterator -- tee: The Back and Forth Iterator -- groupby: The uniq Iterator -- Other Functions -- Decorators -- How to Write a Decorator -- Argument checking -- Caching -- Proxy -- Context Provider -- with and contextlib -- The contextlib Module -- Context Example -- Summary -- 3. Syntax Best Practices-Above the Class Level -- Subclassing Built-in Types -- Accessing Methods from Superclasses -- Understanding Python's Method Resolution Order (MRO) -- super Pitfalls.
Mixing super and classic Calls -- Heterogeneous Arguments -- Best Practices -- Descriptors and Properties -- Descriptors -- Introspection Descriptor -- Meta-descriptor -- Properties -- Slots -- Meta-programming -- The__new__ Method -- __metaclass__ Method -- Summary -- 4. Choosing Good Names -- PEP 8 and Naming Best Practices -- Naming Styles -- Variables -- Constants -- Naming and Usage -- Public and Private Variables -- Functions and Methods -- The Private Controversy -- Special Methods -- Arguments -- Properties -- Classes -- Modules and Packages -- Naming Guide -- Use "has" or "is" Prefix for Boolean Elements -- Use Plural for Elements That Are Sequences -- Use Explicit Names for Dictionaries -- Avoid Generic Names -- Avoid Existing Names -- Best Practices for Arguments -- Build Arguments by Iterative Design -- Trust the Arguments and Your Tests -- Use *args and **kw Magic Arguments Carefully -- Class Names -- Module and Package Names -- Working on APIs -- Tracking Verbosity -- Building the Namespace Tree -- Splitting the Code -- Using Eggs -- Using a Deprecation Process -- Useful Tools -- Pylint -- CloneDigger -- Summary -- 5. Writing a Package -- A Common Pattern for All Packages -- setup.py, the Script That Controls Everything -- sdist -- The MANIFEST.in File -- build and bdist -- bdist_egg -- install -- How to Uninstall a Package -- develop -- test -- register and upload -- Python 2.6 .pypirc Format -- Creating a New Command -- setup.py Usage Summary -- The alias Command -- Other Important Metadata -- The Template-Based Approach -- Python Paste -- Creating Templates -- Creating the Package Template -- Development Cycle -- Summary -- 6. Writing an Application -- Atomisator: An Introduction -- Overall Picture -- Working Environment -- Adding a Test Runner -- Adding a packages Structure -- Writing the Packages -- atomisator.parser.
Creating the Initial Package -- Creating the Initial doctest -- Building the Test Environment -- Writing the Code -- atomisator.db -- SQLAlchemy -- Creating the Mappings -- Providing the APIs -- atomisator.feed -- atomisator.main -- Distributing Atomisator -- Dependencies between Packages -- Summary -- 7. Working with zc.buildout -- zc.buildout Philosophy -- Configuration File Structure -- Minimum Configuration File -- [buildout] Section Options -- The buildout Command -- Recipes -- Notable Recipes -- Creating Recipes -- Atomisator buildout Environment -- buildout Folder Structure -- Going Further -- Releasing and Distributing -- Releasing the Packages -- Adding a Release Configuration File -- Building and Releasing the Application -- Summary -- 8. Managing Code -- Version Control Systems -- Centralized Systems -- Distributed Systems -- Distributed Strategies -- Centralized or Distributed? -- Mercurial -- Project Management with Mercurial -- Setting Up a Dedicated Folder -- Configuring hgwebdir -- Configuring Apache -- Setting Up Authorizations -- Setting Up the Client Side -- Continuous Integration -- Buildbot -- Installing Buildbot -- Hooking Buildbot and Mercurial -- Hooking Apache and Buildbot -- Summary -- 9. Managing Life Cycle -- Different Approaches -- Waterfall Development Model -- Spiral Development Model -- Iterative Development Model -- Defining a Life Cycle -- Planning -- Development -- Global Debug -- Release -- Setting Up a Tracking System -- Trac -- Installation -- Apache Settings -- Permission Settings -- Project Life Cycle with Trac -- Planning -- Development -- Cleaning -- Release -- Summary -- 10. Documenting Your Project -- The Seven Rules of Technical Writing -- Write in Two Steps -- Target the Readership -- Use a Simple Style -- Limit the Scope of the Information -- Use Realistic Code Examples.
Use a Light but Sufficient Approach -- Use Templates -- A reStructuredText Primer -- Section Structure -- Lists -- Inline Markup -- Literal Block -- Links -- Building the Documentation -- Building the Portfolio -- Design -- Common Template -- Usage -- Recipe -- Tutorial -- Module Helper -- Operations -- Make Your Own Portfolio -- Building the Landscape -- Producer's Layout -- Consumer's Layout -- Working on the Index Pages -- Registering Module Helpers -- Adding Index Markers -- Cross-references -- Summary -- 11. Test-Driven Development -- I Don't Test -- Test-Driven Development Principles -- Preventing Software Regression -- Improving Code Quality -- Providing the Best Developer Documentation -- Producing Robust Code Faster -- What Kind of Tests? -- Acceptance Tests -- Unit Tests -- Python Standard Test Tools -- unittest -- doctest -- I Do Test -- Unittest Pitfalls -- Unittest Alternatives -- nose -- Test Runner -- Writing Tests -- Writing Test Fixtures -- Integration with setuptools and Plug-in System -- Wrap-Up -- py.test -- Disabling a Test Class -- Automated Distributed Tests -- Test Starts Immediately -- Wrap-Up -- Fakes and Mocks -- Building a Fake -- Using Mocks -- Document-Driven Development -- Writing a Story -- Summary -- 12. Optimization: General Principles and Profiling Techniques -- The Three Rules of Optimization -- Make It Work First -- Work from the User's Point of View -- Keep the Code Readable(and thus maintainable) -- Optimization Strategy -- Find Another Culprit -- Scale the Hardware -- Write a Speed Test -- Finding Bottlenecks -- Profiling CPU Usage -- Macro-Profiling -- Micro-Profiling -- Measuring Pystones -- Profiling Memory Usage -- How Python Deals with Memory -- Profiling Memory -- A Guppy-PE Primer -- Tracking Memory Usage with Heapy -- C Code Memory Leaks -- Profiling Network Usage -- Summary.
13. Optimization: Solutions -- Reducing the Complexity -- Measuring Cyclomatic Complexity -- Measuring the Big-O Notation -- Simplifying -- Searching in a List -- Using a Set Instead of a List -- Cut the External Calls, Reduce the Workload -- Using Collections -- deque -- defaultdict -- namedtuple -- Multithreading -- What is Multithreading? -- How Python Deals with Threads -- When Should Threading Be Used? -- Building Responsive Interfaces -- Delegating Work -- Multi-User Applications -- Simple Example -- Multiprocessing -- Pyprocessing -- Caching -- Deterministic Caching -- Non-Deterministic Caching -- Pro-Active Caching -- Memcached -- Summary -- 14. Useful Design Patterns -- Creational Patterns -- Singleton -- Structural Patterns -- Adapter -- Interfaces -- Proxy -- Facade -- Behavioral Patterns -- Observer -- Visitor -- Template -- Summary -- Index.
Abstract:
Best practices for designing, coding, and distributing your Python software.
Local Note:
Electronic reproduction. Ann Arbor, Michigan : ProQuest Ebook Central, 2017. Available via World Wide Web. Access may be limited to ProQuest Ebook Central affiliated libraries.
Genre:
Electronic Access:
Click to View