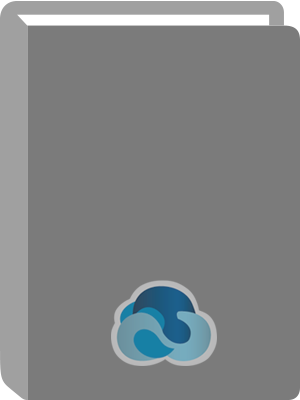
Object Oriented JavaScript.
Title:
Object Oriented JavaScript.
Author:
Stefanov, Stoyan.
ISBN:
9781849693134
Personal Author:
Edition:
2nd ed.
Physical Description:
1 online resource (426 pages)
Contents:
Object-Oriented JavaScript Second Edition -- Table of Contents -- Object-Oriented JavaScript Second Edition -- Credits -- About the Authors -- About the Reviewer -- www.PacktPub.com -- Support files, eBooks, discount offers and more -- Why Subscribe? -- Free Access for Packt account holders -- Preface -- What this book covers -- What you need for this book -- Who this book is for -- Conventions -- Reader feedback -- Customer support -- Errata -- Piracy -- Questions -- 1. Object-oriented JavaScript -- A bit of history -- Browser wars and renaissance -- The present -- The future -- ECMAScript 5 -- Object-oriented programming -- Objects -- Classes -- Encapsulation -- Aggregation -- Inheritance -- Polymorphism -- OOP summary -- Setting up your training environment -- WebKit's Web Inspector -- JavaScriptCore on a Mac -- More consoles -- Summary -- 2. Primitive Data Types, Arrays, Loops, and Conditions -- Variables -- Variables are case sensitive -- Operators -- Primitive data types -- Finding out the value type - the typeof operator -- Numbers -- Octal and hexadecimal numbers -- Exponent literals -- Infinity -- NaN -- Strings -- String conversions -- Special strings -- Booleans -- Logical operators -- Operator precedence -- Lazy evaluation -- Comparison -- Undefined and null -- Primitive data types recap -- Arrays -- Adding/updating array elements -- Deleting elements -- Arrays of arrays -- Conditions and loops -- The if condition -- The else clause -- Code blocks -- Checking if a variable exists -- Alternative if syntax -- Switch -- Loops -- While loops -- Do-while loops -- For loops -- For-in loops -- Comments -- Summary -- Exercises -- 3. Functions -- What is a function? -- Calling a function -- Parameters -- Predefined functions -- parseInt() -- parseFloat() -- isNaN() -- isFinite() -- Encode/decode URIs -- eval() -- A bonus - the alert() function.
Scope of variables -- Variable hoisting -- Functions are data -- Anonymous functions -- Callback functions -- Callback examples -- Immediate functions -- Inner (private) functions -- Functions that return functions -- Function, rewrite thyself! -- Closures -- Scope chain -- Breaking the chain with a closure -- Closure #1 -- Closure #2 -- A definition and closure #3 -- Closures in a loop -- Getter/setter -- Iterator -- Summary -- Exercises -- 4. Objects -- From arrays to objects -- Elements, properties, methods, and members -- Hashes and associative arrays -- Accessing an object's properties -- Calling an object's methods -- Altering properties/methods -- Using the this value -- Constructor functions -- The global object -- The constructor property -- The instanceof operator -- Functions that return objects -- Passing objects -- Comparing objects -- Objects in the WebKit console -- console.log -- Built-in objects -- Object -- Array -- A few array methods -- Function -- Properties of function objects -- Prototype -- Methods of function objects -- Call and apply -- The arguments object revisited -- Inferring object types -- Boolean -- Number -- String -- A few methods of string objects -- Math -- Date -- Methods to work with date objects -- Calculating birthdays -- RegExp -- Properties of RegExp objects -- Methods of RegExp objects -- String methods that accept regular expressions as arguments -- search() and match() -- replace() -- Replace callbacks -- split() -- Passing a string when a RegExp is expected -- Error objects -- Summary -- Exercises -- 5. Prototype -- The prototype property -- Adding methods and properties using the prototype -- Using the prototype's methods and properties -- Own properties versus prototype properties -- Overwriting a prototype's property with an own property -- Enumerating properties -- isPrototypeOf().
The secret __proto__ link -- Augmenting built-in objects -- Augmenting built-in objects - discussion -- Prototype gotchas -- Summary -- Exercises -- 6. Inheritance -- Prototype chaining -- Prototype chaining example -- Moving shared properties to the prototype -- Inheriting the prototype only -- A temporary constructor - new F() -- Uber - access to the parent from a child object -- Isolating the inheritance part into a function -- Copying properties -- Heads-up when copying by reference -- Objects inherit from objects -- Deep copy -- object() -- Using a mix of prototypal inheritance and copying properties -- Multiple inheritance -- Mixins -- Parasitic inheritance -- Borrowing a constructor -- Borrow a constructor and copy its prototype -- Summary -- Case study - drawing shapes -- Analysis -- Implementation -- Testing -- Exercises -- 7. The Browser Environment -- Including JavaScript in an HTML page -- BOM and DOM - an overview -- BOM -- The window object revisited -- window.navigator -- Your console is a cheat sheet -- window.location -- window.history -- window.frames -- window.screen -- window.open()/close() -- window.moveTo() and window.resizeTo() -- window.alert(), window.prompt(), and window.confirm() -- window.setTimeout() and window.setInterval() -- window.document -- DOM -- Core DOM and HTML DOM -- Accessing DOM nodes -- The document node -- documentElement -- Child nodes -- Attributes -- Accessing the content inside a tag -- DOM access shortcuts -- Siblings, body, first, and last child -- Walk the DOM -- Modifying DOM nodes -- Modifying styles -- Fun with forms -- Creating new nodes -- DOM-only method -- cloneNode() -- insertBefore() -- Removing nodes -- HTML-only DOM objects -- Primitive ways to access the document -- document.write() -- Cookies, title, referrer, domain -- Events -- Inline HTML attributes -- Element Properties.
DOM event listeners -- Capturing and bubbling -- Stop propagation -- Prevent default behavior -- Cross-browser event listeners -- Types of events -- XMLHttpRequest -- Sending the request -- Processing the response -- Creating XMLHttpRequest objects in IE prior to Version 7 -- A is for Asynchronous -- X is for XML -- An example -- Summary -- Exercises -- 8. Coding and Design Patterns -- Coding patterns -- Separating behavior -- Content -- Presentation -- Behavior -- Example of separating behavior -- Asynchronous JavaScript loading -- Namespaces -- An Object as a namespace -- Namespaced constructors -- A namespace() method -- Init-time branching -- Lazy definition -- Configuration object -- Private properties and methods -- Privileged methods -- Private functions as public methods -- Immediate functions -- Modules -- Chaining -- JSON -- Design patterns -- Singleton -- Singleton 2 -- Global variable -- Property of the Constructor -- In a private property -- Factory -- Decorator -- Decorating a Christmas tree -- Observer -- Summary -- A. Reserved Words -- Keywords -- Future reserved words -- Previously reserved words -- B. Built-in Functions -- C. Built-in Objects -- Object -- Members of the Object constructor -- The Object.prototype members -- ECMAScript 5 additions to Object -- Array -- The Array.prototype members -- ECMAScript 5 additions to Array -- Function -- The Function.prototype members -- ECMAScript 5 additions to a function -- Boolean -- Number -- Members of the Number constructor -- The Number.prototype members -- String -- Members of the String constructor -- The String.prototype members -- ECMAScript 5 additions to String -- Date -- Members of the Date constructor -- The Date.prototype members -- ECMAScript 5 additions to Date -- Math -- Members of the Math object -- RegExp -- The RegExp.prototype members -- Error objects.
The Error.prototype members -- JSON -- Members of the JSON object -- D. Regular Expressions -- Index.
Abstract:
You will first be introduced to object-oriented programming, then to the basics of objects in JavaScript. This book takes a do-it-yourself approach when it comes to writing code, because the best way to really learn a programming language is by writing code. You are encouraged to type code into Firebug's console, see how it works and then tweak it and play around with it. There are practice questions at the end of each chapter to help you review what you have learned.For new to intermediate JavaScript developer who wants to prepare themselves for web development problems solved by smart JavaScript!.
Local Note:
Electronic reproduction. Ann Arbor, Michigan : ProQuest Ebook Central, 2017. Available via World Wide Web. Access may be limited to ProQuest Ebook Central affiliated libraries.
Genre:
Added Author:
Electronic Access:
Click to View