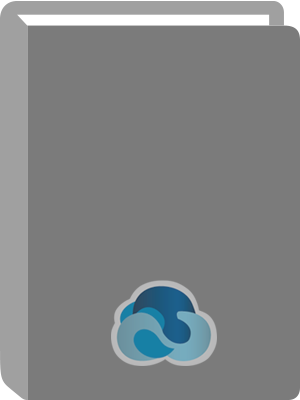
Mastering Concurrency in Go.
Title:
Mastering Concurrency in Go.
Author:
Kozyra, Nathan.
ISBN:
9781783983490
Personal Author:
Physical Description:
1 online resource (368 pages)
Contents:
Mastering Concurrency in Go -- Table of Contents -- Mastering Concurrency in Go -- Credits -- About the Author -- About the Reviewers -- www.PacktPub.com -- Support files, eBooks, discount offers, and more -- Why subscribe? -- Free access for Packt account holders -- Preface -- What this book covers -- What you need for this book -- Who this book is for -- Conventions -- Reader feedback -- Customer support -- Downloading the example code -- Errata -- Piracy -- Questions -- 1. An Introduction to Concurrency in Go -- Introducing goroutines -- A patient goroutine -- Implementing the defer control mechanism -- Using Go's scheduler -- Using system variables -- Understanding goroutines versus coroutines -- Implementing channels -- Channel-based sorting at the letter capitalization factory -- Cleaning up our goroutines -- Buffered or unbuffered channels -- Using the select statement -- Closures and goroutines -- Building a web spider using goroutines and channels -- Summary -- 2. Understanding the Concurrency Model -- Understanding the working of goroutines -- Synchronous versus asynchronous goroutines -- Designing the web server plan -- Visualizing concurrency -- RSS in action -- An RSS reader with self diagnostics -- Imposing a timeout -- A little bit about CSP -- The dining philosophers problem -- Go and the actor model -- Object orientation -- Demonstrating simple polymorphism in Go -- Using concurrency -- Managing threads -- Using sync and mutexes to lock data -- Summary -- 3. Developing a Concurrent Strategy -- Applying efficiency in complex concurrency -- Identifying race conditions with race detection -- Using mutual exclusions -- Exploring timeouts -- Importance of consistency -- Synchronizing our concurrent operations -- The project - multiuser appointment calendar -- Visualizing a concurrent pattern -- Developing our server requirements.
Web server -- The Gorilla toolkit -- Using templates -- Time -- Endpoints -- Custom structs -- A multiuser Appointments Calendar -- A note on style -- A note on immutability -- Summary -- 4. Data Integrity in an Application -- Getting deeper with mutexes and sync -- The cost of goroutines -- Working with files -- Getting low - implementing C -- Touching memory in cgo -- The structure of cgo -- The other way around -- Getting even lower - assembly in Go -- Distributed Go -- Some common consistency models -- Distributed shared memory -- First-in-first-out - PRAM -- Looking at the master-slave model -- The producer-consumer problem -- Looking at the leader-follower model -- Atomic consistency / mutual exclusion -- Release consistency -- Using memcached -- Circuit -- Summary -- 5. Locks, Blocks, and Better Channels -- Understanding blocking methods in Go -- Blocking method 1 - a listening, waiting channel -- Sending more data types via channels -- Creating a function channel -- Using an interface channel -- Using structs, interfaces, and more complex channels -- The net package - a chat server with interfaced channels -- Handling direct messages -- Examining our client -- Blocking method 2 - the select statement in a loop -- Cleaning up goroutines -- Blocking method 3 - network connections and reads -- Creating channels of channels -- Pprof - yet another awesome tool -- Handling deadlocks and errors -- Summary -- 6. C10K - A Non-blocking Web Server in Go -- Attacking the C10K problem -- Failing of servers at 10,000 concurrent connections -- Using concurrency to attack C10K -- Taking another approach -- Building our C10K web server -- Benchmarking against a blocking web server -- Handling requests -- Routing requests -- Serving pages -- Parsing our template -- External dependencies -- Connecting to MySQL -- Multithreading and leveraging multiple cores.
Exploring our web server -- Timing out and moving on -- Summary -- 7. Performance and Scalability -- High performance in Go -- Getting deeper into pprof -- Parallelism's and concurrency's impact on I/O pprof -- Using the App Engine -- Distributed Go -- Types of topologies -- Type 1 - star -- Type 2 - mesh -- The Publish and Subscribe model -- Serialized data -- Remote code execution -- Other topologies -- Message Passing Interface -- Some helpful libraries -- Nitro profiler -- Heka -- GoFlow -- Memory preservation -- Garbage collection in Go -- Summary -- 8. Concurrent Application Architecture -- Designing our concurrent application -- Identifying our requirements -- Using NoSQL as a data store in Go -- MongoDB -- Redis -- Tiedot -- CouchDB -- Cassandra -- Couchbase -- Setting up our data store -- Monitoring filesystem changes -- Managing logfiles -- Handling configuration files -- Detecting file changes -- Sending changes to clients -- Checking records against Couchbase -- Backing up our files -- Designing our web interface -- Reverting a file's history - command line -- Using Go in daemons and as a service -- Checking the health of our server -- Summary -- 9. Logging and Testing Concurrency in Go -- Handling errors and logging -- Breaking out goroutine logs -- Using the LiteIDE for richer and easier debugging -- Sending errors to screen -- Logging errors to file -- Logging errors to memory -- Using the log4go package for robust logging -- Panicking -- Recovering -- Logging our panics -- Catching stack traces with concurrent code -- Using the runtime package for granular stack traces -- Summary -- 10. Advanced Concurrency and Best Practices -- Going beyond the basics with channels -- Building workers -- Implementing nil channel blocks -- Using nil channels -- Implementing more granular control over goroutines with tomb -- Timing out with channels.
Building a load balancer with concurrent patterns -- Choosing unidirectional and bidirectional channels -- Using receive-only or send-only channels -- Using an indeterminate channel type -- Using Go with unit testing -- GoCheck -- Ginkgo and Gomega -- Using Google App Engine -- Utilizing best practices -- Structuring your code -- Documenting your code -- Making your code available via go get -- Keeping concurrency out of your packages -- Summary -- Index.
Abstract:
A practical approach covering everything you need to know to get up and running with Go, starting with the basics and imparting increasingly more detail as the examples and topics become more complicated. The book utilizes a casual, conversational style, rife with actual code and historical anecdotes for perspective, as well as usable and extensible example applications. This book is intended for systems developers and programmers with some experience in either Go and/or concurrent programming who wish to become fluent in building high-performance applications that scale by leveraging single-core, multicore, or distributed concurrency.
Local Note:
Electronic reproduction. Ann Arbor, Michigan : ProQuest Ebook Central, 2017. Available via World Wide Web. Access may be limited to ProQuest Ebook Central affiliated libraries.
Genre:
Electronic Access:
Click to View