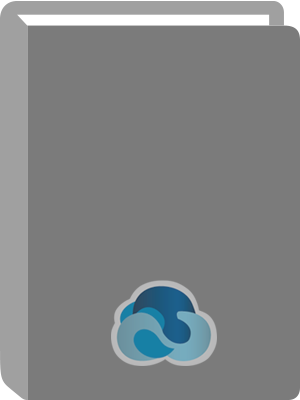
Professional Java for Web Applications.
Title:
Professional Java for Web Applications.
Author:
Williams, Nick.
ISBN:
9781118656518
Personal Author:
Edition:
1st ed.
Physical Description:
1 online resource (939 pages)
Contents:
Cover -- Title Page -- Copyright -- About the Author -- About the Technical Editors -- Credits -- Acknowledgments -- Contents -- Introduction -- Who This Book Is For -- Who This Book Is Not For -- What You Will Learn in This Book -- Part I: Creating Enterprise Applications -- Part II: Adding Spring Framework Into the Mix -- Part III: Persisting Data with JPA and Hibernate ORM -- Part IV: Securing Your Application with Spring Security -- What You Will Not Learn in This Book -- What Tools You Will Need -- Java Development Kit for Java SE 8 -- Integrated Development Environment -- NetBeans IDE 8.0 -- Eclipse Luna IDE 4.4 for Java EE Developers -- IntelliJ IDEA 13 Ultimate Edition -- Java EE 7 Web Container -- Conventions Used in This Book -- Code Examples -- Maven Dependencies -- Why Security Is at the End of the Book -- Errata -- Part 1: Creating Enterprise Applications -- Chapter 1: Introducing Java Platform, Enterprise Edition -- A Timeline of Java Platforms -- In the Beginning -- The Birth of Enterprise Java -- Java SE and Java EE Evolving Together -- Understanding the Most Recent Platform Features -- A Continuing Evolution -- Understanding the Basic Web Application Structure -- Servlets, Filters, Listeners, and JSPs -- Directory Structure and WAR Files -- The Deployment Descriptor -- Class Loader Architecture -- Enterprise Archives -- Summary -- Chapter 2: Using Web Containers -- Choosing a Web Container -- Apache Tomcat -- GlassFish -- JBoss and WildFly -- Other Containers and Application Servers -- Why You'll Use Tomcat in This Book -- Installing Tomcat on Your Machine -- Installing as a Windows Service -- Installing as a Command-Line Application -- Configuring a Custom JSP Compiler -- Deploying and Undeploying Applications in Tomcat -- Performing a Manual Deploy and Undeploy -- Using the Tomcat Manager -- Debugging Tomcat from Your IDE.
Using IntelliJ IDEA -- Using Eclipse -- Summary -- Chapter 3: Writing Your First Servlet -- Creating a Servlet Class -- What to Extend -- Using the Initializer and Destroyer -- Configuring a Servlet for Deployment -- Adding the Servlet to the Descriptor -- Mapping the Servlet to a URL -- Running and Debugging Your Servlet -- Understanding doGet(), doPost(), and Other Methods -- What Should Happen during the service Method Execution? -- Using HttpServletRequest -- Using HttpServletResponse -- Using Parameters and Accepting Form Submissions -- Configuring your Application Using Init Parameters -- Using Context Init Parameters -- Using Servlet Init Parameters -- Uploading Files from a Form -- Introducing the Customer Support Project -- Configuring the Servlet for File Uploads -- Accepting a File Upload -- Making Your Application Safe for Multithreading -- Understanding Requests, Threads, and Method Execution -- Protecting Shared Resources -- Summary -- Chapter:4 Using JSPs to Display Content -- Is Easier Than output.println("") -- Why JSPs Are Better -- What Happens to a JSP at Run Time -- Creating Your First JSP -- Understanding the File Structure -- Directives, Declarations, Scriptlets, and Expressions -- Commenting Your Code -- Adding Imports to Your JSP -- Using Directives -- Using the Tag -- Using Java within a JSP (and Why You Shouldn't!) -- Using the Implicit Variables in a JSP -- Why You Shouldn't Use Java in a JSP -- Combining Servlets and JSPs -- Configuring JSP Properties in the Deployment Descriptor -- Forwarding a Request from a Servlet to a JSP -- A Note about JSP Documents (JSPX) -- Summary -- Chapter 5: Maintaining State Using Sessions -- Understanding Why Sessions Are Necessary -- Maintaining State -- Remembering Users -- Enabling Application Workflow -- Using Session Cookies and URL Rewriting.
Understanding the Session Cookie -- Session IDs in the URL -- Session Vulnerabilities -- Storing Data in a Session -- Configuring Sessions in the Deployment Descriptor -- Storing and Retrieving Data -- Removing Data -- Storing More Complex Data in Sessions -- Applying Sessions Usefully -- Adding Login to the Customer Support Application -- Detecting Changes to Sessions Using Listeners -- Maintaining a List of Active Sessions -- Clustering an Application That Uses Sessions -- Using Session IDs in a Cluster -- Understand Session Replication and Failover -- Summary -- Chapter 6: Using the Expression Language in JSPs -- Understanding Expression Language -- What It's For -- Understanding the Base Syntax -- Placing EL Expressions -- Writing with the EL Syntax -- Reserved Keywords -- Operator Precedence -- Object Properties and Methods -- EL Functions -- Static Field and Method Access -- Enums -- Lambda Expressions -- Collections -- Using Scoped Variables in EL Expressions -- Using the Implicit EL Scope -- Using the Implicit EL Variables -- Accessing Collections with the Stream API -- Understanding Intermediate Operations -- Using Terminal Operations -- Putting the Stream API to Use -- Replacing Java Code with Expression Language -- Summary -- Chapter 7: Using the Java Standard Tag Library -- Introducing JSP Tags and the JSTL -- Working with Tags -- Using the Core Tag Library (C Namespace) -- -- -- -- , , and -- -- -- -- -- and -- Putting Core Library Tags to Use -- Using the Internationalization and Formatting Tag Library (FMT Namespace) -- Internationalization and Localization Components -- -- -- and -- -- and.
and -- and -- Putting i18n and Formatting Library Tags to Use -- Using the Database Access Tag Library (SQL Namespace) -- Using the XML Processing Tag Library (X Namespace) -- Replacing Java Code with JSP Tags -- Summary -- Chapter 8: Writing Custom Tag and Function Libraries -- Understanding TLDs, Tag Files, and Tag Handlers -- Reading the Java Standard Tag Library TLD -- Comparing JSP Directives and Tag File Directives -- Creating Your First Tag File to Serve as an HTML Template -- Creating a More Useful Date Formatting Tag Handler -- Creating an EL Function to Abbreviate Strings -- Replacing Java Code with Custom JSP Tags -- Summary -- Chapter 9: Improving Your Application Using Filters -- Understanding the Purpose of Filters -- Logging Filters -- Authentication Filters -- Compression and Encryption Filters -- Error Handling Filters -- Creating, Declaring, and Mapping Filters -- Understanding the Filter Chain -- Mapping to URL Patterns and Servlet Names -- Mapping to Different Request Dispatcher Types -- Using the Deployment Descriptor -- Using Annotations -- Using Programmatic Configuration -- Ordering Your Filters Properly -- URL Pattern Mapping versus Servlet Name Mapping -- Exploring Filter Order with a Simple Example -- Using Filters with Asynchronous Request Handling -- Investigating Practical Uses for Filters -- Adding a Simple Logging Filter -- Compressing Response Content Using a Filter -- Simplifying Authentication with a Filter -- Summary -- Chapter 10: Making Your Application Interactive with WebSockets -- Evolution: From Ajax to WebSockets -- Problem: Getting New Data from the Server to the Browser -- Solution 1: Frequent Polling -- Solution 2: Long Polling -- Solution 3: Chunked Encoding -- Solution 4: Applets and Adobe Flash.
WebSockets: The Solution Nobody Knew Kind of Already Existed -- Understanding the WebSocket APIS -- HTML5 (JavaScript) Client API -- Java WebSocket APIs -- Creating Multiplayer Games with WebSockets -- Implementing the Basic Tic-Tac-Toe Algorithm -- Creating the Server Endpoint -- Writing the JavaScript Game Console -- Playing WebSocket Tic-Tac-Toe -- Using WebSockets to Communicate in a Cluster -- Simulating a Simple Cluster Using Two Servlet Instances -- Transmitting and Receiving Binary Messages -- Testing the Simulated Cluster Application -- Adding "Chat with Support" to the Customer Support Application -- Using Encoders and Decoders to Translate Messages -- Creating the Chat Server Endpoint -- Writing the JavaScript Chat Application -- Summary -- Chapter 11: Using Logging to Monitor Your Application -- Understanding the Concepts of Logging -- Why You Should Log -- What Content You Might Want to See in Logs -- How Logs Are Written -- Using Logging Levels and Categories -- Why Are There Different Logging Levels? -- Logging Levels Defined -- How Logging Categories Work -- How Log Sifting Works -- Choosing a Logging Framework -- API versus Implementation -- Performance -- A Quick Look at Apache Commons Logging and SLF4J -- Introducing Log4j 2 -- Integrating Logging into Your Application -- Creating the Log4j 2 Configuration Files -- Utilizing Fish Tagging with a Web Filter -- Writing Logging Statements in Java Code -- Using the Log Tag Library in JSPs -- Logging in the Customer Support Application -- Summary -- Part 2: Adding Spring Framework Into the Mix -- Chapter 12: Introducing Spring Framework -- What Is Spring Framework? -- Inversion of Control and Dependency Injection -- Aspect-Oriented Programming -- Data Access and Transaction Management -- Application Messaging -- Model-View-Controller Pattern for Web Applications -- Why Spring Framework?.
Logical Code Groupings.
Abstract:
The comprehensive Wrox guide for creating Java web applications for the enterprise This guide shows Java software developers and software engineers how to build complex web applications in an enterprise environment. You'll begin with an introduction to the Java Enterprise Edition and the basic web application, then set up a development application server environment, learn about the tools used in the development process, and explore numerous Java technologies and practices. The book covers industry-standard tools and technologies, specific technologies, and underlying programming concepts. Java is an essential programming language used worldwide for both Android app development and enterprise-level corporate solutions As a step-by-step guide or a general reference, this book provides an all-in-one Java development solution Explains Java Enterprise Edition 7 and the basic web application, how to set up a development application server environment, which tools are needed during the development process, and how to apply various Java technologies Covers new language features in Java 8, such as Lambda Expressions, and the new Java 8 Date & Time API introduced as part of JSR 310, replacing the legacy Date and Calendar APIs Demonstrates the new, fully-duplex WebSocket web connection technology and its support in Java EE 7, allowing the reader to create rich, truly interactive web applications that can push updated data to the client automatically Instructs the reader in the configuration and use of Log4j 2.0, Spring Framework 4 (including Spring Web MVC), Hibernate Validator, RabbitMQ, Hibernate ORM, Spring Data, Hibernate Search, and Spring Security Covers application logging, JSR 340 Servlet API 3.1, JSR 245 JavaServer Pages (JSP) 2.3 (including custom tag libraries), JSR 341 Expression Language 3.0, JSR 356 WebSocket API 1.0, JSR 303/349 Bean
Validation 1.1, JSR 317/338 Java Persistence API (JPA) 2.1, full-text searching with JPA, RESTful and SOAP web services, Advanced Message Queuing Protocol (AMQP), and OAuth Professional Java for Web Applications is the complete Wrox guide for software developers who are familiar with Java and who are ready to build high-level enterprise Java web applications.
Local Note:
Electronic reproduction. Ann Arbor, Michigan : ProQuest Ebook Central, 2017. Available via World Wide Web. Access may be limited to ProQuest Ebook Central affiliated libraries.
Genre:
Added Author:
Electronic Access:
Click to View