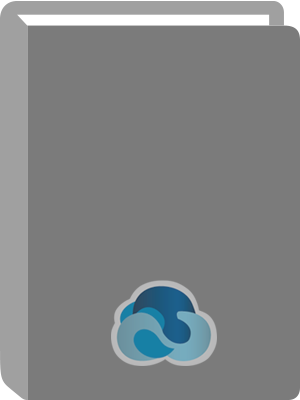
MUD Game Programming.
Başlık:
MUD Game Programming.
Yazar:
Penton, Ron.
ISBN:
9789781931840
Yazar Ek Girişi:
Fiziksel Tanımlama:
1 online resource (704 pages)
İçerik:
Title -- Copyright -- Dedication -- Acknowledgments -- About the Author -- Contents at a Glance -- Contents -- Introduction -- History -- MUD Design -- The SimpleMUD -- BetterMUD -- Expectations -- Book Layout -- Part One-The Basics -- Chapter 1-Introduction to Network Programming -- Chapter 2-Winsock/Berkeley Sockets Programming -- Chapter 3-Introduction to Multithreading -- Chapter 4-The Basic Library -- Chapter 5-The Socket Library -- Chapter 6-Telnet Protocol and a Simple Chat Server -- Part Two-Creating a SimpleMUD -- Chapter 7-Designing the SimpleMUD -- Chapter 8-Items and Players -- Chapter 9-Maps, Stores, and Training Rooms -- Chapter 10-Enemies, Combat, and the Game Loop -- Part Three-Creating a BetterMUD -- Chapter 11-The BetterMUD -- Chapter 12-Entities, Accessors, and Databases -- Chapter 13-Entities and Databases Continued -- Chapter 14-Scripts, Actions, Logic, and Commands -- Chapter 15-Game Logic -- Chapter 16-The Networking System -- Chapter 17-Python -- Chapter 18-Making the Game -- Appendixes -- Appendix A-Setting Up Your Compilers -- Appendix B-Socket Error Codes -- Appendix C-C++ Primer -- Appendix D-Template Primer -- Glossary -- Let's Get Ready to Rumble -- PART ONE The Basics -- CHAPTER 1 Introduction to Network Programming -- Why Learn the Basics? -- History of Communication Networks in a Nutshell -- Electric Communication-Telegraphs to Telephones -- Switched Communication -- Packet-Switched Networks -- Mechanics of Packets and the Internet Wonderland -- All About IPv4 -- IPv6: Bigger and Better -- IP Philosophy and Layered Hierarchy -- Network Layer -- Internet Layer -- Transport Layer -- Application Layer -- Other Layers -- Common Transport Protocols -- UDP -- TCP to the Rescue! -- Information on Networking Protocols -- Summary -- CHAPTER 2 Winsock/Berkeley Sockets Programming -- Byte Ordering -- What Is a Socket?.
Listening Sockets -- Data Sockets -- Connection Diagrams -- Sockets API -- Header Files -- Socket API Errors -- Initializing the API -- Creating a TCP Listening Socket -- Creating the Socket -- Binding the Socket -- Listening -- Accepting Connections -- Creating a TCP Data Socket -- Creating the Socket -- Connecting the Socket -- Sending Data -- Receiving Data -- Closing a Socket -- Miscellaneous Functions -- Converting IP Addresses to Strings and Back -- Getting Socket Information -- Domain Name System -- Performing a DNS Lookup -- Demo 2.1 Hello Internet Server -- Include Files -- Platform-Independent Defines -- The Rest of the Code -- Demo 2.2 Hello Internet Client -- Using select() to Avoid Multithreading -- Demo 2.3 Hello Internet Server v2 -- Demo 2.4 Hello Internet Client v2 -- Summary -- CHAPTER 3 Introduction to Multithreading -- What Is Multithreading? -- What Is a Thread? -- Synchronization -- Mutexes -- Semaphores -- Condition Objects -- Problems with Multithreading -- Threads Use More Memory -- Threads Require More Processing -- Deadlock -- Corruption -- Debugging -- Don't Let the Bedbugs Bite -- ThreadLib -- Headers and Typedefs -- Creating a New Thread -- ThreadLib::DummyData Structure -- ThreadLib::DummyRun Function -- ThreadLib::Create Function -- Getting the ID of a Thread -- Waiting for a Thread to Finish -- Killing a Thread -- Yielding a Thread -- Demo 3.1-Basic Threading -- Demo 3.2-Yielding -- ThreadLib Mutexes -- Data -- Constructor -- Destroying a Mutex -- Locking a Mutex -- Unlocking a Mutex -- Demo 3.3-Mutexes -- Summary -- CHAPTER 4 The Basic Library -- Big Numbers -- What Time Is It? -- Windows -- Getting the Frequency -- Getting the Time -- Linux -- Milliseconds -- What's the Frequency, Kenneth? -- Other Times -- Timestamps -- Timers -- Strings -- Creating Strings -- Using Strings -- Other String Functions.
My Own String Functions -- Changing Cases -- Trimming Whitespace -- Parsing -- Conversions -- Searching and Replacing -- Logging -- Decorators -- The Logger -- The Constructor -- The Destructor -- It's Log, Log-It's Big, It's Heavy, It's Wood! -- Using the Logger -- Summary -- CHAPTER 5 The Socket Library -- Sockets API Wrapper Classes and Functions -- Socket Wrapper -- Listening Sockets -- Data Sockets -- Using Sockets -- Socket Sets -- Function Wrappers -- Errors -- Error Codes -- Translating Errors -- The SocketLib::Exception Class -- The Winsock Initializer -- Connections, Managers, and Policies, Oh My! -- Protocol Policies -- Connections -- New Variables -- Protocol Object -- The Stack of Handlers -- A Sending Buffer -- Rates and Times -- Other Data -- New Functions -- The Constructors -- Receiving Data -- Sending Functions -- Closing Functions -- Handler Functions -- Other Functions -- Listening Manager -- Constructor and Destructor -- Adding New Sockets -- Listening for New Connections -- Connection Manager -- Variables -- Functions -- Constructor and Destructor -- Adding New Connections -- Closing Connections -- Listening for Data -- Sending Data -- Summary -- CHAPTER 6 Telnet Protocol and a Simple Chat Server -- Telnet -- Simple Telnet Example -- Demo 6.1-Very Simple Telnet Server -- Running Demo 6.1 -- Processing Codes -- Telnet Options -- VT100 Terminal Codes -- ConnectionHandler Class -- Creating a Telnet Protocol Class -- Data -- Functions -- Codes -- Demo 6.2-SimpleChat -- Database -- Users -- Database Data -- Iterator Functions -- Database Functions -- Valid Usernames -- Logon Handler -- No Room -- New Connections -- Handling Commands -- Other Functions -- Chat Handler -- New Connections -- Exiting Connections -- Handling Commands -- The SendAll Function -- Closing Function -- Other Functions -- Tying It All Together -- Summary.
PART TWO Creating a SimpleMUD -- CHAPTER 7 Designing the SimpleMUD -- Choosing Game Characteristics -- Setting -- Players -- Attributes -- Core Attributes -- Life Attributes -- Accuracy and Dodging Attributes -- Damage Attributes -- Bases -- Experience and Leveling -- Inventory -- Other Data -- Items -- Weapons of Mass Destruction -- Your Knight in Shining Armor -- Heal, Brother! -- Here There Be Dragons! -- It's a Small World, After All -- Give Me Some Room Here -- Come and See What's in Store -- Mortal Combat -- I Command Thee -- Player Commands -- God and Admin Commands -- Summary -- CHAPTER 8 Items and Players -- Groundwork -- Entities -- Accessors -- Full Matching -- Partial Matching -- Entity Function Listing -- Entity Functors -- Entity Database Classes -- Map-Based Database -- Class Definition and Data -- Iterator Inner Class -- Searching the Database -- ID-Based Lookups -- Name-Based Lookups -- EntityDatabase Function Listing -- Vector-Based Database -- Class Definition and Data -- Functions -- Database Pointers -- Before Templates, There Were Macros -- Conversion Operators -- Dereference Operators -- Stream Operators -- Defining the Macros -- Logs -- Attributes -- Attribute Class -- Attribute Sets -- Items -- Item Class -- Item Types -- Money: It's What I Want -- Item Attributes -- Writing and Reading Items from Disk -- Item Function Listing -- Item Database -- File Storage -- Class Definition -- Loading the Database -- Item Database Pointers -- Populating Your Realm with Players -- Player Class -- Player Variables -- Player Ranks -- Directly Modifiable Attributes -- Level Functions -- Informational Level Functions -- Training -- Attribute Functions -- Recalculating Stats -- Hitpoint Functions -- Attribute Functions -- Other Attribute Functions -- Item Functions -- Accessors -- Helpers -- Inventory Modification.
Weapon and Armor Modification -- Item Searching -- Constructor -- Communication -- Functors -- File Functions -- Player Function Listing -- Disk Storage -- PlayerDatabase -- Class Declaration -- Loading the Database -- Saving the Database -- Saving a Single Player -- Adding New Players -- Searching the Database -- Logging Out -- Database Pointers -- Handler Design -- Logon Handler -- Logon States -- Logon Data -- Logon Functions -- Hanging Up, Flooding, and No Room -- Leaving and Entering -- Handling Commands -- GotoGame Function -- Training Handler -- Class Skeleton -- Leaving and Entering -- Closing Connections -- Handling Training Commands -- Printing Stats -- Game Handler -- Game Data -- Game Functions -- Handler Functions -- New Connections -- Leaving the Handler -- Closed Connections -- Handling Commands -- Sending Functions -- Sending to the Game and Sending Globally -- Helpers -- Whispering -- Status Printers -- Item Functions -- Using an Item -- Disarming an Item -- Helpers -- Demo 8.1-The SimpleMUD Baseline: The Core, Players, and Items -- Summary -- CHAPTER 9 Maps, Stores, and Training Rooms -- Adding New Features to the Baseline -- Rooms -- Room Data -- Template Data -- Volatile Data -- Temporary Volatile Data -- Room Functions -- Accessors -- Player Functions -- Item Functions -- File Functions -- The Room Database -- Loading Templates -- Loading Data -- Saving Data -- Room Database Pointers -- Stores -- Helper Functions -- Stream Extraction Function -- The Store Database -- Ch-Ch-Ch-Changes -- Entering and Leaving the Realm -- Entering -- Leaving -- New Room-Related Commands -- New Room Functions -- Printing Room Descriptions -- Moving Between Rooms -- Getting Items -- Dropping Items -- Sending Text to a Room -- Commands Related to New Store -- The StoreList Function -- Buying Items -- Selling Items -- New Training Room Commands.
Database Reloading.
Notlar:
Electronic reproduction. Ann Arbor, Michigan : ProQuest Ebook Central, 2017. Available via World Wide Web. Access may be limited to ProQuest Ebook Central affiliated libraries.
Tür:
Elektronik Erişim:
Click to View