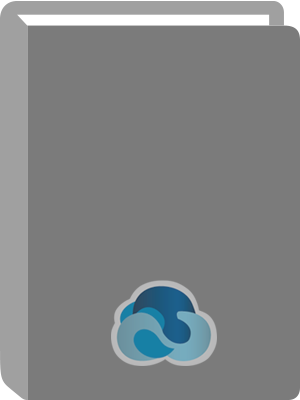
Linux Game Programming.
Başlık:
Linux Game Programming.
Yazar:
Baker, Steve.
ISBN:
9781592003785
Yazar Ek Girişi:
Fiziksel Tanımlama:
1 online resource (368 pages)
İçerik:
Title -- Copyright -- Contents at a Glance -- Contents -- Acknowledgments -- Author Bio -- Introduction -- My Joys with Linux -- CHAPTER 1 Introduction to Game Development -- What Makes a Game? -- Different Game Genres -- Shoot-em-ups -- Strategy Games -- Simulations -- Platform Games -- Puzzle Games -- Adventure Games -- Role-Play Games -- Card Games -- Sports Games -- What Makes a Successful Game? -- The Game-Development Process -- The Design Document -- Development -- Testing -- Releasing Your Game -- OpenSource Games -- Commercial Games -- Shareware Games -- CHAPTER 2 Linux Development Tools -- Development Tools -- Compiler -- Debugging Tools -- Development Environments -- Libraries -- Simple DirectMedia Layer -- OpenGL -- libX11 -- Other Libraries -- CHAPTER 3 The Structure of a Game -- The Parts of a Game -- A Game Framework -- CHAPTER 4 2D Graphics Under Linux -- The SDL API -- Starting Up SDL -- Creating a Window -- Creating a Surface -- Drawing a Bitmap Image -- Drawing Directly to the Screen -- Pixel Formats -- Plotting Pixels -- Using Palettes -- Cleaning Up -- The Xlib API -- Creating a Window -- Drawing to the Screen -- Plotting a Pixel -- Drawing Images -- Double Buffering -- Installing Color Maps -- Cleaning Up -- The SVGAlib API -- Switching to a Graphics Mode -- Drawing to the Screen -- Displaying Images -- Understanding the PCX Format -- Displaying the Image -- Using Color Palettes -- Cleaning Up -- A Mini-Project -- CHAPTER 5 Input with SDL -- Understanding Input Devices -- Keyboard -- Mouse -- Joystick -- Handling Methods -- Event Queues -- Polling -- Using the SDL Event Queue -- The SDL_Event Union -- Layout -- Interpreting Event Types -- Reading from the Event Queue -- Keyboard Events -- Mouse Events -- Joystick Events -- Polling with SDL -- Polling the Keyboard -- Polling the Mouse -- Configuring the Control System.
CHAPTER 6 3D Graphics for Linux Games -- Some History: Mesa and OpenGL -- What Is OpenGL? -- Where Can I Get OpenGL? -- Buffers, Hidden Surface Elimination, and Animation -- Getting Started -- Compiling and Linking with OpenGL -- Let's Draw Something! -- Drawing Polygons, Lines, and Points -- Drawing in 3D: Perspective and Movement -- Perspective and the Projection Matrix -- Drawing in Orthographic or Perspective Mode -- Code That Moves the Object -- Texturing and Other "State" Elements -- The GL_CULL_FACE Option -- The GL_LIGHTING Option -- The GL_TEXTURE_2D Option -- The GL_COLOR_MATERIAL Option -- The GL_BLEND Option -- The GL_ALPHA_TEST Option -- The GL_DEPTH_TEST Option -- Lighting -- Specifying the Lighting Qualities of the Object -- Establishing the Light Source -- Setting Up Surface Normals -- Texturing the Model -- Loading the Texture Image -- MIP Mapping the Image -- Applying the Texture -- Transparency and the Alpha Component -- Solving Transparency Problems -- Fogging -- CHAPTER 7 Using OpenGL in Games -- Understanding Display Lists -- Understanding Vertex Arrays -- Drawing with Triangle Strips and Fans -- Adding Text -- Understanding Matrices -- Working with Plane Equations, Line Equations, and Distances in 3D -- Using Billboards to Simulate 3D -- Working with Particles -- Detecting Collisions -- Simplify the Shapes You Test -- If the Objects Are Close, Test Again -- Follow Surface Contours -- The Next Layer: Scene Graph APIs -- Field-of-View Culling -- State Management -- Scene Graphs -- Moving the Camera -- Advanced Lighting -- Putting It All Together -- CHAPTER 8 Sound Under Linux -- OpenAL -- A Simple OpenAL Application -- Initializing and Loading Samples -- Creating Listeners -- Loading a Sound File -- Creating Sound Sources -- Playing Sounds -- Getting the State of the Sound -- Stopping Sounds -- Deleting a Source.
Moving the Source -- Compiling the Sample -- Advanced OpenAL -- Volume Control -- Velocity and Doppler Shift -- Reverb and Echo -- Streaming Buffers -- SDL Audio -- Starting an Audio Session -- Loading Samples -- Using the Audio Callback Function -- Playing a Sample -- Pausing the Audio Stream -- Cleaning Up -- Mixing Different Samples -- CD Audio -- Using the ioctl() Function -- Opening the Device -- Getting a Track Listing -- Playing a CD -- Tracks and Indices -- Some Code -- Stopping a CD -- CHAPTER 9 Networking -- Introduction to Modern Networking -- Peer-to-Peer Networking -- Client/Server Networking -- The TCP/IP Stack -- TCP -- UDP -- Socket Programming -- Opening a Socket -- Connecting the Socket -- Sending Data -- Opening a Socket as a File Stream -- Using the send() Function -- The sendto() Function -- A Mini-Exercise -- Receiving Data -- The recvfrom() Function -- The select() Function -- Another Mini-Exercise -- The Complete Mini-Web Browser -- Creating a Server -- Binding the Socket -- Using the listen() Function -- Accepting Connections -- Threads and Networking -- Clients -- Using Flags to Communicate between Threads -- Using Message Queues to Communicate between Threads -- Servers -- Pings -- Networking in Games -- Dead Reckoning -- Sending Controller Inputs -- Lobbies -- What Is OpenLobby? -- Using Lobby Server -- Connecting to the Lobby Server -- Logging In to the Lobby Server -- Finding Games -- Creating Games -- Ending Games -- Chatting in the Lobby -- Listing Chat Rooms -- Joining and Leaving Rooms -- Sending Chat Messages -- Cleaning Up -- Security Issues -- Buffer Overflows -- Denial-of-Service Attacks -- CHAPTER 10 Artificial Intelligence -- Basic Artificial Intelligence -- Route Finding -- State-Trees -- Generate the State-Tree -- Modify the Tree to Accommodate Multiple Routes -- Search the Tree -- The Nurgle Method.
Grouping -- Problem Solving -- Trial and Error -- Min-Max Trees -- The Theory Behind the Tree -- Putting It into Practice -- Advanced Artificial Intelligence -- Genetic Algorithms -- Memes -- The Genebot -- Neural Networks -- Artificial Neural Networks -- Changing the Behavior of the Network -- Personality -- CHAPTER 11 OpenSource: Friend or Foe? -- What Is OpenSource? -- Unlimited Redistribution -- Ability to Charge for Expenses -- The Development Models -- The Cathedral -- The Bazaar -- Reasons For and Against OpenSource -- Ten Reasons to Use OpenSource -- Peer Reviews -- Security -- Available Code -- Free Software, Free Information -- Many Developers -- Fame -- The Community Aspect -- Availability of the Software -- The Cause -- Because You Like It -- Ten Reasons to Write Closed-Source Software -- Money -- Paranoia -- Plagiarists -- New Technologies -- Contracts -- Selfishness -- The "He Didn't, So I Won't" Attitude -- Politics -- Ego -- Security through Obscurity -- The Middle Ground -- Free Code, Private Data -- OpenSource Later -- Limited License -- Key Technologies -- The Indrema Debate -- Big Red Warning -- Emulators -- Time-Share -- Registered Testers -- The Solution -- Which License to Use? -- Viral Licenses -- Open Licenses -- Custom Licenses -- Liability -- APPENDIX A OpenSource License Agreements -- GNU General Public License -- Preamble -- TERMS AND CONDITIONS FOR COPYING, DISTRIBUTION, AND MODIFICATION -- GNU Lesser General Public License -- Preamble -- TERMS AND CONDITIONS FOR COPYING, DISTRIBUTION, AND MODIFICATION -- BSD License -- Artistic License -- Preamble -- Definitions -- Mozilla Public License -- 1.0. Definitions -- 2.0. Source Code License -- 3.0. Distribution Obligations -- 4.0. Inability to Comply Due to Statute or Regulation -- 5.0 Application of this License -- 6.0. Versions of the License -- 7.0. DISCLAIMER OF WARRANTY.
8.0. TERMINATION -- 9.0. LIMITATION OF LIABILITY -- 10.0. U.S. GOVERNMENT END USERS -- 11.0. MISCELLANEOUS -- 12.0. RESPONSIBILITY FOR CLAIMS -- EXHIBIT A. -- The Apache Software License -- MIT License -- APPENDIX B Porting -- Porting Strategy -- Try Not to Fork the Codebase -- Getting Started -- #ifdef Is Your Friend -- The Harder Stuff -- First Light -- Compiler Issues -- Visual C/C++ Improper Scoping in for Loops -- GCC Code Size -- Mixing Signed and Unsigned Types -- Assignment from void* -- GCC Doesn't Complain About Missing Return Values -- GCC Doesn't Complain About Use of Uninitialized Variables -- GCC Is More Strict About Implicit Casting -- Include Filenames -- Struct Packing -- File System Issues -- Case Sensitivity -- Drive Letters and Path Separators -- Line Termination -- File Attributes and Home Directories -- Assembly Language -- Operand Order -- Register Naming -- Opcode Naming and Operand Size -- Immediate Values -- Addressing Modes -- Endianess -- Windows Issues -- WinMain() and Message Loops -- Timers -- The Registry -- Hungarian Notation -- Graphics Issues -- DirectDraw -- DOS Frame Buffer -- Direct3D -- Coordinate Systems -- Polygon Winding -- Texture Management -- OpenGL Extensions -- Sound Issues -- Networking Issues -- Winsock -- File and Socket Handles -- Include Files -- Return Codes -- Different Calls -- DirectPlay Is Evil -- Useful Links -- Footnotes -- APPENDIX C References -- Web Sites -- GameDev.Net -- GamaSutra -- Flipcode -- Happy Penguin -- Indrema Developer Network -- Linux Games -- Linux Game Developer Resource Centre -- Books -- OpenGL Super Bible (The Red Book) -- OpenGL Reference (The Blue Book) -- Graphics Programming Black Book -- Game Architecture and Design -- TCP/IP Illustrated (Volume 2) -- Linux Device Drivers -- Game Development Series -- Magazines -- Game Developer Magazine -- Develop -- Edge.
Newspapers.
Notlar:
Electronic reproduction. Ann Arbor, Michigan : ProQuest Ebook Central, 2017. Available via World Wide Web. Access may be limited to ProQuest Ebook Central affiliated libraries.
Tür:
Elektronik Erişim:
Click to View