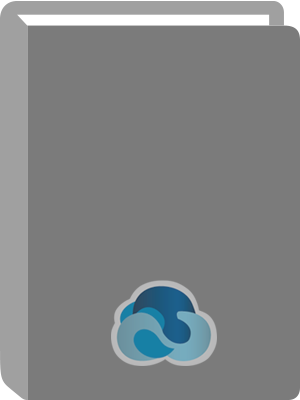
Node.js Design Patterns.
Başlık:
Node.js Design Patterns.
Yazar:
Casciaro, Mario.
ISBN:
9781783287321
Yazar Ek Girişi:
Fiziksel Tanımlama:
1 online resource (497 pages)
İçerik:
Node.js Design Patterns -- Table of Contents -- Node.js Design Patterns -- Credits -- About the Author -- Acknowledgments -- About the Reviewers -- www.PacktPub.com -- Support files, eBooks, discount offers, and more -- Why subscribe? -- Free access for Packt account holders -- Preface -- What this book covers -- What you need for this book -- Who this book is for -- Conventions -- Reader feedback -- Customer support -- Downloading the example code -- Errata -- Piracy -- Questions -- 1. Node.js Design Fundamentals -- The Node.js philosophy -- Small core -- Small modules -- Small surface area -- Simplicity and pragmatism -- The reactor pattern -- I/O is slow -- Blocking I/O -- Non-blocking I/O -- Event demultiplexing -- The reactor pattern -- The non-blocking I/O engine of Node.js - libuv -- The recipe for Node.js -- The callback pattern -- The continuation-passing style -- Synchronous continuation-passing style -- Asynchronous continuation-passing style -- Non continuation-passing style callbacks -- Synchronous or asynchronous? -- An unpredictable function -- Unleashing Zalgo -- Using synchronous APIs -- Deferred execution -- Node.js callback conventions -- Callbacks come last -- Error comes first -- Propagating errors -- Uncaught exceptions -- The module system and its patterns -- The revealing module pattern -- Node.js modules explained -- A homemade module loader -- Defining a module -- Defining globals -- module.exports vs exports -- require is synchronous -- The resolving algorithm -- The module cache -- Cycles -- Module definition patterns -- Named exports -- Exporting a function -- Exporting a constructor -- Exporting an instance -- Modifying other modules or the global scope -- The observer pattern -- The EventEmitter -- Create and use an EventEmitter -- Propagating errors -- Make any object observable.
Synchronous and asynchronous events -- EventEmitter vs Callbacks -- Combine callbacks and EventEmitter -- Summary -- 2. Asynchronous Control Flow Patterns -- The difficulties of asynchronous programming -- Creating a simple web spider -- The callback hell -- Using plain JavaScript -- Callback discipline -- Applying the callback discipline -- Sequential execution -- Executing a known set of tasks in sequence -- Sequential iteration -- Web spider version 2 -- Sequential crawling of links -- The pattern -- Parallel execution -- Web spider version 3 -- The pattern -- Fixing race conditions in the presence of concurrent tasks -- Limited parallel execution -- Limiting the concurrency -- Globally limiting the concurrency -- Queues to the rescue -- Web spider version 4 -- The async library -- Sequential execution -- Sequential execution of a known set of tasks -- Sequential iteration -- Parallel execution -- Limited parallel execution -- Promises -- What is a promise? -- Promises/A+ implementations -- Promisifying a Node.js style function -- Sequential execution -- Sequential iteration -- Sequential iteration - the pattern -- Parallel execution -- Limited parallel execution -- Generators -- The basics -- A simple example -- Generators as iterators -- Passing values back to a generator -- Asynchronous control flow with generators -- Generator-based control flow using co -- Sequential execution -- Parallel execution -- Limited parallel execution -- Producer-consumer pattern -- Limiting the download tasks concurrency -- Comparison -- Summary -- 3. Coding with Streams -- Discovering the importance of streams -- Buffering vs Streaming -- Spatial efficiency -- Gzipping using a buffered API -- Gzipping using streams -- Time efficiency -- Composability -- Getting started with streams -- Anatomy of streams -- Readable streams -- Reading from a stream.
The non-flowing mode -- The flowing mode -- Implementing Readable streams -- Writable streams -- Writing to a stream -- Back-pressure -- Implementing Writable streams -- Duplex streams -- Transform streams -- Implementing Transform streams -- Connecting streams using pipes -- Useful packages for working with streams -- readable-stream -- through and from -- Asynchronous control flow with streams -- Sequential execution -- Unordered parallel execution -- Implementing an unordered parallel stream -- Implementing a URL status monitoring application -- Unordered limited parallel execution -- Ordered parallel execution -- Piping patterns -- Combining streams -- Implementing a combined stream -- Forking streams -- Implementing a multiple checksum generator -- Merging streams -- Creating a tarball from multiple directories -- Multiplexing and demultiplexing -- Building a remote logger -- Client side - Multiplexing -- Server side - demultiplexing -- Running the mux/demux application -- Multiplexing and demultiplexing object streams -- Summary -- 4. Design Patterns -- Factory -- A generic interface for creating objects -- A mechanism to enforce encapsulation -- Building a simple code profiler -- In the wild -- Proxy -- Techniques for implementing proxies -- Object composition -- Object augmentation -- A comparison of the different techniques -- Creating a logging Writable stream -- Proxy in the ecosystem - function hooks and AOP -- In the wild -- Decorator -- Techniques for implementing decorators -- Composition -- Object augmentation -- Decorating a LevelUP database -- Introducing LevelUP and LevelDB -- Implementing a LevelUP plugin -- In the wild -- Adapter -- Using LevelUP through the filesystem API -- In the wild -- Strategy -- Multi-format configuration objects -- In the wild -- State -- Implementing a basic fail-safe socket -- Template.
A configuration manager template -- In the wild -- Middleware -- Middleware in Express -- Middleware as a pattern -- Creating a middleware framework for ØMQ -- The Middleware Manager -- A middleware to support JSON messages -- Using the ØMQ middleware framework -- The server -- The client -- Command -- A flexible pattern -- The task pattern -- A more complex command -- Summary -- 5. Wiring Modules -- Modules and dependencies -- The most common dependency in Node.js -- Cohesion and Coupling -- Stateful modules -- The Singleton pattern in Node.js -- Patterns for wiring modules -- Hardcoded dependency -- Building an authentication server using hardcoded dependencies -- The db module -- The authService module -- The authController module -- The app module -- Running the authentication server -- Pros and cons of hardcoded dependencies -- Dependency injection -- Refactoring the authentication server to use dependency injection -- The different types of dependency injection -- Pros and cons of dependency injection -- Service locator -- Refactoring the authentication server to use a service locator -- Pros and cons of a service locator -- Dependency injection container -- Declaring a set of dependencies to a DI container -- Refactoring the authentication server to use a DI container -- Pros and cons of a Dependency Injection container -- Wiring plugins -- Plugins as packages -- Extension points -- Plugin-controlled vs Application-controlled extension -- Implementing a logout plugin -- Using hardcoded dependencies -- Exposing services using a service locator -- Exposing services using dependency injection -- Exposing services using a dependency injection container -- Summary -- 6. Recipes -- Requiring asynchronously initialized modules -- Canonical solutions -- Preinitialization queues -- Implementing a module that initializes asynchronously.
Wrapping the module with preinitialization queues -- In the wild -- Asynchronous batching and caching -- Implementing a server with no caching or batching -- Asynchronous request batching -- Batching requests in the total sales web server -- Asynchronous request caching -- Caching requests in the total sales web server -- Notes about implementing caching mechanisms -- Batching and caching with Promises -- Running CPU-bound tasks -- Solving the subset sum problem -- Interleaving with setImmediate -- Interleaving the steps of the subset sum algorithm -- Considerations on the interleaving pattern -- Using multiple processes -- Delegating the subset sum task to other processes -- Implementing a process pool -- Communicating with a child process -- Communicating with the parent process -- Considerations on the multiprocess pattern -- Sharing code with the browser -- Sharing modules -- Universal Module Definition -- Creating an UMD module -- Considerations on the UMD pattern -- Introducing Browserify -- Exploring the magic of Browserify -- The advantages of using Browserify -- Fundamentals of cross-platform development -- Runtime code branching -- Build-time code branching -- Design patterns for cross-platform development -- Sharing business logic and data validation using Backbone Models -- Implementing the shared models -- Implementing the platform-specific code -- Using the isomorphic models -- Running the application -- Summary -- 7. Scalability and Architectural Patterns -- An introduction to application scaling -- Scaling Node.js applications -- The three dimensions of scalability -- Cloning and load balancing -- The cluster module -- Notes on the behavior of the cluster module -- Building a simple HTTP server -- Scaling with the cluster module -- Resiliency and availability with the cluster module -- Zero-downtime restart.
Dealing with stateful communications.
Özet:
If you're a JavaScript developer interested in a deeper understanding of how to create and design Node.js applications, this is the book for you.
Notlar:
Electronic reproduction. Ann Arbor, Michigan : ProQuest Ebook Central, 2017. Available via World Wide Web. Access may be limited to ProQuest Ebook Central affiliated libraries.
Konu Başlığı:
Tür:
Elektronik Erişim:
Click to View